The following code implements a Java Pasta timer that looks like the application below. • Once the start button is pressed the timer will count to two minutes. • The timer can be stopped by pressing stop. • When the timer is finished, it should pop-up an appropriate dialog box that states the time is up. You are given a partial implementation of program. Fill out the missing code to complete the program import javax.swing.*; import java.awt.event.*; import javax.swing.border.*; public class PastaTimerApp extends implements { private JProgressBar progrBar; private JButton startButton, stopButton; private JLabel textLabel; private PastaTimer PastaTimer; public PastaTimerApp() { JPanel p = new JPanel(); p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS)); p.setBorder(new TitledBorder("Pasta Timer:")); progrBar = new JProgressBar(JProgressBar.HORIZONTAL, 0, 540); textLabel = new JLabel(" 0 Seconds "); p.add(progrBar); p.add(textLabel); // Initialize buttons and add action listeners JPanel controls = new JPanel(); controls.add(startButton); controls.add(stopButton); this.getContentPane().add("Center", p); this.getContentPane().add("South", controls); this.setSize(600, 130); //Set default close operation and make it visible. this.PastaTimer = } //end PastTimerApp() constructor method public void actionPerformed(ActionEvent e) { } //end actionPerformed public static void main(String[] args) { } //end main }//end PastaTimerApp class PastaTimer extends { private int numberSeconds = 0; private boolean running = true; private JProgressBar progressReference; private JLabel textLabel; public PastaTimer(JProgressBar progressReference, JLabel textLabel){ } // end PastaTimer constructor public void run() { while (running) { if (this.updateProgress() == false) { // stop the thread try { Thread.currentThread().sleep(100); } } //end while } //end run // Displays a message and returns false when timer is done. private boolean updateProgress() { int current = progressReference.getValue(); int updateTo = ++current; if (updateTo <= 540) { this.progressReference.setValue(updateTo); this.textLabel.setText(" " + updateTo + " Seconds "); return true; } else { this.running = false; this.displayDialog(); return false; }//end if else } //end update progress public void stopTimer() { this.running = false;} private void displayDialog() { } }
The following code implements a Java Pasta timer that looks like the application below.
• Once the start button is pressed the timer will count to two minutes.
• The timer can be stopped by pressing stop.
• When the timer is finished, it should pop-up an appropriate dialog box that states the time
is up.
You are given a partial implementation of program. Fill out the missing code to complete the
program
import javax.swing.*;
import java.awt.event.*;
import javax.swing.border.*;
public class PastaTimerApp extends implements {
private JProgressBar progrBar;
private JButton startButton, stopButton;
private JLabel textLabel;
private PastaTimer PastaTimer;
public PastaTimerApp() {
JPanel p = new JPanel();
p.setLayout(new BoxLayout(p, BoxLayout.Y_AXIS));
p.setBorder(new TitledBorder("Pasta Timer:"));
progrBar = new JProgressBar(JProgressBar.HORIZONTAL, 0, 540);
textLabel = new JLabel(" 0 Seconds ");
p.add(progrBar);
p.add(textLabel);
// Initialize buttons and add action listeners
JPanel controls = new JPanel();
controls.add(startButton);
controls.add(stopButton);
this.getContentPane().add("Center", p);
this.getContentPane().add("South", controls);
this.setSize(600, 130);
//Set default close operation and make it visible.
this.PastaTimer =
} //end PastTimerApp() constructor method
public void actionPerformed(ActionEvent e) {
} //end actionPerformed
public static void main(String[] args) {
} //end main
}//end PastaTimerApp
class PastaTimer extends {
private int numberSeconds = 0;
private boolean running = true;
private JProgressBar progressReference;
private JLabel textLabel;
public PastaTimer(JProgressBar progressReference, JLabel textLabel){
} // end PastaTimer constructor
public void run() {
while (running) {
if (this.updateProgress() == false) { // stop the thread
try {
Thread.currentThread().sleep(100);
}
} //end while
} //end run
// Displays a message and returns false when timer is done.
private boolean updateProgress() {
int current = progressReference.getValue();
int updateTo = ++current;
if (updateTo <= 540) {
this.progressReference.setValue(updateTo);
this.textLabel.setText(" " + updateTo + " Seconds ");
return true;
} else {
this.running = false;
this.displayDialog();
return false;
}//end if else
} //end update progress
public void stopTimer() { this.running = false;}
private void displayDialog() {
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

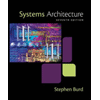
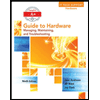
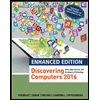
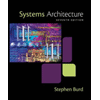
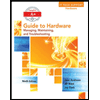
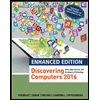