The following BST dataInReverse Order function has an error. Complete the testDataInReverseOrder test function using the provided assert True function to discover dataInReverseOrder's error (i.e., fail). The test should pass once the function is fixed. Note: this code is meant to be compiled by itself, so do not assume the existence of an insert function, etc. #include #include #include using namespace std; void assertTrue (bool b, string s) { if (b) { cout << "PASSED: " << s << endl; } else { cout << "FAILED: " << s << endl; } } struct BSTNode { int data; BSTNode left; BSTNode" right; }; // returns a vector of all the data in a BST in reverse sorted order (i.e., descending order) vector dataInReverseOrder (BSTNode* root) { // empty tree case if (root == nullptr) return {}; // non-empty tree vector right = data InReverseOrder (root->right);
The following BST dataInReverse Order function has an error. Complete the testDataInReverseOrder test function using the provided assert True function to discover dataInReverseOrder's error (i.e., fail). The test should pass once the function is fixed. Note: this code is meant to be compiled by itself, so do not assume the existence of an insert function, etc. #include #include #include using namespace std; void assertTrue (bool b, string s) { if (b) { cout << "PASSED: " << s << endl; } else { cout << "FAILED: " << s << endl; } } struct BSTNode { int data; BSTNode left; BSTNode" right; }; // returns a vector of all the data in a BST in reverse sorted order (i.e., descending order) vector dataInReverseOrder (BSTNode* root) { // empty tree case if (root == nullptr) return {}; // non-empty tree vector right = data InReverseOrder (root->right);
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 28SA
Related questions
Question

Transcribed Image Text:The following BST dataInReverseOrder function has an error. Complete the testDataInReverseOrder test function using the provided assert True function to
discover dataInReverseOrder's error (i.e., fail). The test should pass once the function is fixed.
Note: this code is meant to be compiled by itself, so do not assume the existence of an insert function, etc.
#include <string>
#include <vector>
#include <iostream>
using namespace std;
void assertTrue (bool b, string s) {
if (b) {
cout << "PASSED: " << s << endl;
} else {
cout << "FAILED: " << s << endl;
}
}
struct BSTNode {
int data;
BSTNode* left;
BSTNode* right;
};
// returns a vector of all the data in a BST in reverse sorted order (i.e., descending order)
vector<int> dataInReverseOrder (BSTNode* root) {
}
// empty tree case
if (root == nullptr) return {};
// non-empty tree
vector<int> right = dataInReverseOrder (root->right);
vector<int> left = dataInReverseOrder (root->left);
vector<int> res;
for (int x: right) res.push_back(x);
for (int x : left) res.push_back(x);
res.push_back(root->data);
return res;
}
void testDataInReverseOrder() {
// FIXME: implement a test that calls dataInReverseOrder and assertTrue
// The test should discover the error and fail. The test should
// pass once the function is fixed.
}
int main() {
testDataInReverseOrder();
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
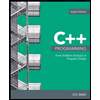
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
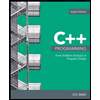
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning