The Doctor program described in Chapter 5 combines the data model of a doctor and the operations for handling user interaction. Restructure this program according to the model/view pattern so that these areas of responsibility are assigned to separate sets of classes. The program should include a Doctor class with an interface that allows one to obtain a greeting, a signoff message, and a reply to a patient’s string. To implement the greeting, define a method named greeting for the Doctor class. To implement the signoff message, define a method named farewell for the Doctor class. Both greeting and farewell should return a string with a greeting or farewell message respectively. The reply function is defined for you, it should be added as a method for the Doctor class. The rest of the program, in a separate main program module, handles the user’s interactions with the Doctor object. Develop this program with a terminal-based user interface. Note: The program should output in the following format: Hello, how can I help you today? > I am sick You seem to think that you are sick? > Yes And what do you think about this? > I am not feeling good Did I just hear you say that you are not feeling good? > Yes Why do you believe that Yes? > My nose is running I would like to hear more about that. > I have a headache too You seem to think that you have a headache too? > Correct Go on. > It doesn't stop Did I just hear you say that It doesn't stop? > Correct Go on. > That's it And what do you think about this? > quit Have a nice day
The Doctor
The program should include a Doctor class with an interface that allows one to obtain a greeting, a signoff message, and a reply to a patient’s string.
To implement the greeting, define a method named greeting for the Doctor class. To implement the signoff message, define a method named farewell for the Doctor class. Both greeting and farewell should return a string with a greeting or farewell message respectively. The reply function is defined for you, it should be added as a method for the Doctor class.
The rest of the program, in a separate main program module, handles the user’s interactions with the Doctor object. Develop this program with a terminal-based user interface.
Note: The program should output in the following format:
Hello, how can I help you today?
> I am sick
You seem to think that you are sick?
> Yes
And what do you think about this?
> I am not feeling good
Did I just hear you say that you are not feeling good?
> Yes
Why do you believe that Yes?
> My nose is running I would like to hear more about that.
> I have a headache too
You seem to think that you have a headache too?
> Correct Go on.
> It doesn't stop
Did I just hear you say that It doesn't stop?
> Correct Go on.
> That's it
And what do you think about this? > quit Have a nice day!
![doctor.py
Doctor.py
+
1
2 File: doctor.py
3 Project 5
4 Conducts an interactive session of nondirective psychotherapy.
5 Adds a history list of earlier patient sentences, which can
6 be chosen for replies to shift the conversation to an earlier topic.
7
8
9 import random
10
11 history = []
12
13 # All doctors share the same qualifiers, replacements, and hedges.
14
15 qualifiers = ['Why do you say that ', 'You seem to think that ',
16
17
18
19 replacements = {'i': 'you', 'me': 'you', 'my': 'your',
20
21
22
23 hedges = [ 'Go on.', 'I would like to hear more about that.',
24
25
26 def reply(sentence):
27
28
29
30
31
32
33
34
35
36
37
38
39
40
'Did I just hear you say that ',
'Why do you believe that']
'we': 'you', 'us': 'you', 'am': 'are',
'you': 'i', 'you': 'I'}
'And what do you think about this?', 'Please continue.]
"" Implements three different reply strategies."""|
probability = random.randint (1, 5)
if probability in (1, 2):
# Just hedge
answer = random.choice (hedges)
elif probability == 3 and len(history) > 3:
# Go back to an earlier topic
answer = "Earlier you said that " + \
changePerson(random.choice(history))
else:
# Transform the current input
answer = random.choice(qualifiers) + changePerson(sentence)
# Always add the current sentence to the history list
history.append(sentence)
41
return answer
42
43 def changePerson (sentence):
44
45
"""Replaces first person pronouns with second person
pronouns, "
words = sentence.split ()
replyWords = []
for word in words:
replyWords.append(replacements.get(word, word))
return " ".join(replyWords)
46
47
48
49
50
51
52 def main():
53
54
55
56
57
58
59
60
61
""Handles the interaction between patient and doctor."""
print("Good morning, I hope you are well today.")
print ("What can I do for you?")
while True:
sentence = input ("\n>> ")
if sentence.upper() == "QUIT":
print("Have a nice day!")
break
print(reply(sentence))
62
63 # The entry point for program execution
64 if -_name__ == "__main__":
65
66
67
main()](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff2366eef-3e30-4c87-8a9d-bef7108edd86%2F138023b4-6435-484e-92f7-b71c9506e9a5%2Fjrdgt0jb_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

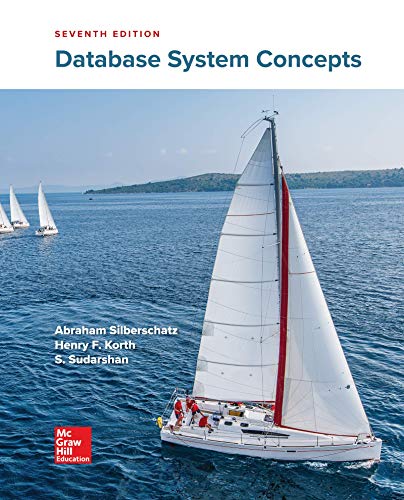
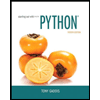
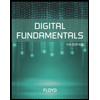
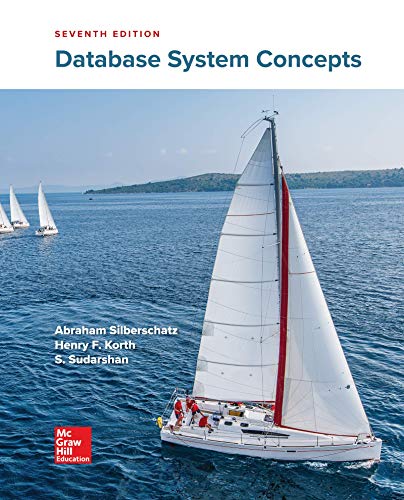
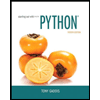
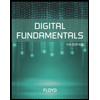
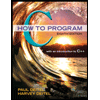
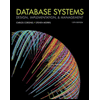
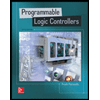