The circular queue is a linear data structure in which the operations are performed based on FIFO (First In First Out) principle and the last position is connected back to the first position to make a circle. One of the benefits of the circular queue is that we can make use of the spaces in front of the queue. In a normal queue, once the queue becomes full, we cannot insert the next element even if there is a space in front of the queue. But using the circular queue, we can use the space to store new values. • Write a C++ class CircularQueue in file CircularQueue.cpp that implements the above stack with following public methods. CircularQueue(int k) : Constructor, set the size of the queue to k. - int front() : Get the front item from the queue. If the queue is empty, return -1. - int rear() : Get the last item from the queue. If the queue is empty, return -1. - bool enqueue(int value) : Insert an element into the circular queue. Return true if the operation is successful. - bool dequeue() : Delete an element from the circular queue. Return true if the operation is successful. - bool isEmpty() : Checks whether the circular queue is empty or not. - bool isFull() : Checks whether the circular queue is full or not. All other methods or variables in your implementation should be private. Example: CircularQueue myQueue(4); // sets the size to be 4. myQueue.enqueue(1); // returns true myQueue.enqueue(2); // returns true myQueue.enqueue(3); // returns true - myQueue.enqueue(4); // returns true - myQueue.enqueue(5); // returns false, the queue is full myQueue.front(); // returns 1 myQueue.rear(); // returns 4 - myQueue.isFull(); // returns true - myQueue.dequeue(); // returns true - myQueue.enqueue(5); // returns true - myQueue.front(); // returns 1 myQueue.rear(); // returns 5
The circular queue is a linear data structure in which the operations are performed based on FIFO (First In First Out) principle and the last position is connected back to the first position to make a circle. One of the benefits of the circular queue is that we can make use of the spaces in front of the queue. In a normal queue, once the queue becomes full, we cannot insert the next element even if there is a space in front of the queue. But using the circular queue, we can use the space to store new values. • Write a C++ class CircularQueue in file CircularQueue.cpp that implements the above stack with following public methods. CircularQueue(int k) : Constructor, set the size of the queue to k. - int front() : Get the front item from the queue. If the queue is empty, return -1. - int rear() : Get the last item from the queue. If the queue is empty, return -1. - bool enqueue(int value) : Insert an element into the circular queue. Return true if the operation is successful. - bool dequeue() : Delete an element from the circular queue. Return true if the operation is successful. - bool isEmpty() : Checks whether the circular queue is empty or not. - bool isFull() : Checks whether the circular queue is full or not. All other methods or variables in your implementation should be private. Example: CircularQueue myQueue(4); // sets the size to be 4. myQueue.enqueue(1); // returns true myQueue.enqueue(2); // returns true myQueue.enqueue(3); // returns true - myQueue.enqueue(4); // returns true - myQueue.enqueue(5); // returns false, the queue is full myQueue.front(); // returns 1 myQueue.rear(); // returns 4 - myQueue.isFull(); // returns true - myQueue.dequeue(); // returns true - myQueue.enqueue(5); // returns true - myQueue.front(); // returns 1 myQueue.rear(); // returns 5
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Please solve using c++
Take your time and do solve

Transcribed Image Text:The circular queue is a linear data structure in which the operations are performed based on FIFO
(First In First Out) principle and the last position is connected back to the first position to make a
circle.
One of the benefits of the circular queue is that we can make use of the spaces in front of the queue.
In a normal queue, once the queue becomes full, we cannot insert the next element even if there is a
space in front of the queue. But using the circular queue, we can use the space to store new values.
• Write a C++ class CircularQueue in file CircularQueue.cpp that implements the above stack
with following public methods.
CircularQueue(int k) : Constructor, set the size of the queue to k.
- int front() : Get the front item from the queue. If the queue is empty, return -1.
- int rear() : Get the last item from the queue. If the queue is empty, return -1.
- bool enqueue(int value) : Insert an element into the circular queue. Return true if the
operation is successful.
- bool dequeue() : Delete an element from the circular queue. Return true if the operation
is successful.
- bool isEmpty() : Checks whether the circular queue is empty or not.
- bool isFull() : Checks whether the circular queue is full or not.
All other methods or variables in your implementation should be private.
Example:
CircularQueue myQueue(4); // sets the size to be 4.
myQueue.enqueue(1); // returns true
myQueue.enqueue(2); // returns true
myQueue.enqueue(3); // returns true
- myQueue.enqueue(4); // returns true
- myQueue.enqueue(5); // returns false, the queue is full
myQueue.front(); // returns 1
myQueue.rear(); // returns 4
- myQueue.isFull(); // returns true
- myQueue.dequeue(); // returns true
- myQueue.enqueue(5); // returns true
- myQueue.front(); // returns 1
myQueue.rear(); // returns 5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
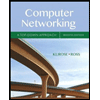
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
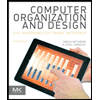
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
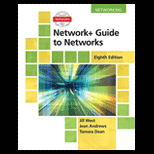
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
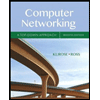
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
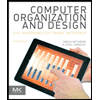
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
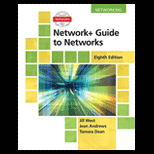
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
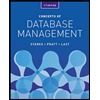
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
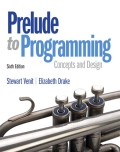
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
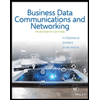
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY