Temperature Class Creat Temreratuce.b (header file) Temperature.cpp (class file) TemperatureDriver.cpp (Driver file) Data members: Appropriate data members are declared Pre-processing directives: Appropriate Pre-processing and using directives Constructors: Two argument constructor initializes data members Member functions are correct and used correctly: Set and get functions for data members Get function for total number of temperatures addTemperaturel) -- add s a single temperature to the vector sortTemreratuces() - sorts vector in ascending order averageTemperatucel) findMinTemperatwcel) and findMaxTemperaturel)
Temperature Class Creat Temreratuce.b (header file) Temperature.cpp (class file) TemperatureDriver.cpp (Driver file) Data members: Appropriate data members are declared Pre-processing directives: Appropriate Pre-processing and using directives Constructors: Two argument constructor initializes data members Member functions are correct and used correctly: Set and get functions for data members Get function for total number of temperatures addTemperaturel) -- add s a single temperature to the vector sortTemreratuces() - sorts vector in ascending order averageTemperatucel) findMinTemperatwcel) and findMaxTemperaturel)
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter12: Adding Functionality To Your Classes
Section12.2: Providing Class Conversion Capabilities
Problem 6E
Related questions
Question
Can I please get help writing this in C++

Transcribed Image Text:Use good coding style (modular, separate .h and cpp class files, no globals. meaningful
comments, etc.) throughout your program. Finally, make sure that you do not misspell the word
"temperature" in your output to the user.
Compiles and Executes without crashing
Style: No globals, Files are closed after use, code is modular
Appropriate Internal Documentation
Temperature Class Created:
Temrerature.b (header file)
Temperature.cpp (class file)
TemperatureDriver.cpp (Driver file)
Data members:
Appropriate data members are declared
Pre-processing directives:
Appropriate Pre-processing and using directives
Constructors:
Two argument constructor initializes data members
Member functions are correct and used correctly:
Set and get functions for data members
Get function for total number of temperatures
addTemperaturel) -- add s a single temperature to the vector
Sottemperatures() - sorts vector in ascending order
averageTemperaturel)
findMinTemperaturel) and findMaxTemperature()
displayTemperatures)
Protection:
Member functions and variables are declared with appropriate
protection (i.e. private or public)
Object Creation:
Temperatures object created correctly
User Interaction:
Prompts user for file name
Appropriate error checking is in place to ensure that the file exists
Temperatures are correctly read in from the file and stored in the temperatures
object
QUeut is labeled and formatted appropriately
Error checking is performed on input data: It outputs appropriate error message;
does not close the program until the error message can be read; indicates the
problem with the invalid item.
Quput consists of the number of temperatures read in, the month and year of the
data, the sorted vector of temperatures, the average of the temperatures, and the
low and high temperatures for the month - all formatted appropriately
![7. A member function to return the number of temperatures that were read in for the month.
This assignment utilizes a class in an application that might be useful in the "real world." It
requires the sorting of, and computation with, data involving temperatures.
8. A member function to display the sorted temperatures.
Requirements:
You are working for a meteorologist to maintain a list of temperatures and some related statistics
for a month.
Write a program (client) that uses the class by creating a Temperatures object and prompting the
user for a file name. Appropriate error checking is required to ensure that the file exists and can
be opened successfully.
Class and Data members:
The client should read in the file contents and store them in the object. The file will be formatted
Create a class called Temperature that stores temperature readings (integers) in a vector (do not
use an array). The class should have data members that store the month name and year of when
such that the first line contains the month name, the second line contains the year, and each
successive line contains a temperature. A typical input file might contain:
June
the temperature readings were collected.
2019
Constructor(s):
The class should have a 2-argument constructor that receives the month name and year as
parameters and sets the appropriate data members to these values.
90
85
97
91
Member Functions:
87
The class should have functions as follows:
86
88
1. Member functions to set and get the month and year variables.
82
83
A member function that adds a single temperature to the vector. Both negative and positive
temperatures are allowed. Call this function AddTenmperature.
2.
85
Note
the
may contain any number of temperatures for a given month. Therefore, you
need to read in and store each temperature until you reach the end of the file.
The client (i.e. main()) should read in the contents of the file. After each temperature is read in,
it should call the member function in the Temperatures class to add the new temperature (ie
3. A member function to sort the vector in ascending order.
Feel free to use the sort function that is available in the algorithm library for sorting vectors.
Or, if you would prefer to write your own sort code, you may find this site to be helpful:
one temperature at a time) to the vector.
http://www.cplusplus.com/articles/NHAORXSZ/
Main() should then produce a report that displays the month and year of the data, the total
number of observations (temperatures) in the file, the lowest temperature, the highest
temperature, the average temperature, and finally, a listing of all of the temperatures that were
read in. The listing of all of the temperatures must be displayed in sorted order (ascending -
from lowest to highest).
4. A member function to compute the average (x) of the temperatures in the vector. The formula for
calculating an average is
x= Exi/n
where xi is the value of each temperature reading and
n is the total number of temperature readings in the vector.
All output should be labeled appropriately, and validity checking should be done on input of the
filename and also the temperatures that are read in.
5. A member function to determine the lowest temperature for the month. [Note that to receive
If a non-numeric value is encountered in reading in the temperatures, the program should output
an error message indicating that a non-numeric value was found in the file, and the entire
program should then terminate. If a non-numeric is found, consider the entire file to be
corrupted and don't try to produce any calculations nor display the contents of the vector - just
end the program with an appropriate error message. (Make sure the error message is displayed
long enough for the user to read it before ending the program.) Again, negative temperatures are
permitted, but non-numeric temperatures should be caught.
credit for this function, it must contain an algorithm to search through the vector to determine the
minimum value. You cannot simply sort the vector and return the first data member.]
6. A member function to determine the highest temperature for the month. [Note that to receive
credit for this function, it must contain an algorithm to search through the vector to determine the
maximum value. You cannot simply sort the vector and return the last data member.]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb7c26524-1992-4254-a68c-dd4401eae989%2F212320c0-6597-4e53-aaeb-08e93410d50b%2F278l7qh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:7. A member function to return the number of temperatures that were read in for the month.
This assignment utilizes a class in an application that might be useful in the "real world." It
requires the sorting of, and computation with, data involving temperatures.
8. A member function to display the sorted temperatures.
Requirements:
You are working for a meteorologist to maintain a list of temperatures and some related statistics
for a month.
Write a program (client) that uses the class by creating a Temperatures object and prompting the
user for a file name. Appropriate error checking is required to ensure that the file exists and can
be opened successfully.
Class and Data members:
The client should read in the file contents and store them in the object. The file will be formatted
Create a class called Temperature that stores temperature readings (integers) in a vector (do not
use an array). The class should have data members that store the month name and year of when
such that the first line contains the month name, the second line contains the year, and each
successive line contains a temperature. A typical input file might contain:
June
the temperature readings were collected.
2019
Constructor(s):
The class should have a 2-argument constructor that receives the month name and year as
parameters and sets the appropriate data members to these values.
90
85
97
91
Member Functions:
87
The class should have functions as follows:
86
88
1. Member functions to set and get the month and year variables.
82
83
A member function that adds a single temperature to the vector. Both negative and positive
temperatures are allowed. Call this function AddTenmperature.
2.
85
Note
the
may contain any number of temperatures for a given month. Therefore, you
need to read in and store each temperature until you reach the end of the file.
The client (i.e. main()) should read in the contents of the file. After each temperature is read in,
it should call the member function in the Temperatures class to add the new temperature (ie
3. A member function to sort the vector in ascending order.
Feel free to use the sort function that is available in the algorithm library for sorting vectors.
Or, if you would prefer to write your own sort code, you may find this site to be helpful:
one temperature at a time) to the vector.
http://www.cplusplus.com/articles/NHAORXSZ/
Main() should then produce a report that displays the month and year of the data, the total
number of observations (temperatures) in the file, the lowest temperature, the highest
temperature, the average temperature, and finally, a listing of all of the temperatures that were
read in. The listing of all of the temperatures must be displayed in sorted order (ascending -
from lowest to highest).
4. A member function to compute the average (x) of the temperatures in the vector. The formula for
calculating an average is
x= Exi/n
where xi is the value of each temperature reading and
n is the total number of temperature readings in the vector.
All output should be labeled appropriately, and validity checking should be done on input of the
filename and also the temperatures that are read in.
5. A member function to determine the lowest temperature for the month. [Note that to receive
If a non-numeric value is encountered in reading in the temperatures, the program should output
an error message indicating that a non-numeric value was found in the file, and the entire
program should then terminate. If a non-numeric is found, consider the entire file to be
corrupted and don't try to produce any calculations nor display the contents of the vector - just
end the program with an appropriate error message. (Make sure the error message is displayed
long enough for the user to read it before ending the program.) Again, negative temperatures are
permitted, but non-numeric temperatures should be caught.
credit for this function, it must contain an algorithm to search through the vector to determine the
minimum value. You cannot simply sort the vector and return the first data member.]
6. A member function to determine the highest temperature for the month. [Note that to receive
credit for this function, it must contain an algorithm to search through the vector to determine the
maximum value. You cannot simply sort the vector and return the last data member.]
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
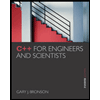
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
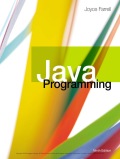
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
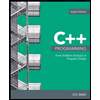
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
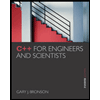
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
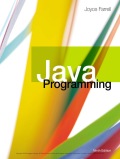
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
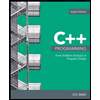
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning