Suppose now that the university is going to extend their system by creating two derived classes based on the Student class, UGStudent and GradStudent: class GradStudent (Student): def _init_(self, first, last, id): Student._init_(self, first, last, id) self.passed_writtens = False self.lab = None def take_writtens (grade): if grade > 80: self.passed_writtens = True class UGStudent (Student): def _init_(self, first, last, id, on_campus = False): Student._init_(self, first, last, id) self.on_campus = on_campus self.dorm = None Given that the following declarations are made: u1 = UGStudent('Bob', 'Jackson', 112345, False) g1 = GradStudent('Greg', 'Student', 712331) s1 = Student('Sally', 'Tester', 234663) Which of the following expressions are legal and will not generate errors? u1.add_course('CSC12021') s1.take_writtens(92) print(g1.on_campus) g1.take_writtens(76) print(g1.overload()) print(s1.dorm) print(g1.jd)
Suppose now that the university is going to extend their system by creating two derived classes based on the Student class, UGStudent and GradStudent: class GradStudent (Student): def _init_(self, first, last, id): Student._init_(self, first, last, id) self.passed_writtens = False self.lab = None def take_writtens (grade): if grade > 80: self.passed_writtens = True class UGStudent (Student): def _init_(self, first, last, id, on_campus = False): Student._init_(self, first, last, id) self.on_campus = on_campus self.dorm = None Given that the following declarations are made: u1 = UGStudent('Bob', 'Jackson', 112345, False) g1 = GradStudent('Greg', 'Student', 712331) s1 = Student('Sally', 'Tester', 234663) Which of the following expressions are legal and will not generate errors? u1.add_course('CSC12021') s1.take_writtens(92) print(g1.on_campus) g1.take_writtens(76) print(g1.overload()) print(s1.dorm) print(g1.jd)
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Question

Transcribed Image Text:Suppose now that the university is going to extend their system by creating two derived classes based on the Student class, UGStudent and GradStudent:
class GradStudent(Student):
def _init_(self, first, last, id):
Student._init_(self, first, last, id)
self.passed_writtens = False
self.lab = None
def take_writtens (grade):
if grade > 80:
self.passed_writtens = True
class UGStudent (Student):
def _init_(self, first, last, id, on_campus = False):
Student._init_(self, first, last, id)
self.on_campus = on_campus
self.dorm = None
Given that the following declarations are made:
u1 = UGStudent('Bob', 'Jackson', 112345, False)
g1 = GradStudent('Greg', 'Student', 712331)
s1 = Student('Sally', 'Tester', 234663)
Which of the following expressions are legal and will not generate errors?
u1.add_course('CSC12021')
s1.take_writtens(92)
print(g1.on_campus)
g1.take_writtens(76)
print(g1.overload())
print(s1.dorm)
print(g1.id)
OOO 0 000
![class Student:
def _init_(self, firstname, lastname, idnum):
self.first = firstname
self.last = lastname
self.id = idnum
self.courses = []
def add_course(self, course):
''' don't allow overloads or signing up for the same course twice
if course not in self.courses and len(self.courses) < 5:
self.courses.append (course)
def clear_courses(self):
''clear this student's course schedule''"
self.courses = []
def overload (self):
return len(self.courses) >= 4
What are all of the attributes of this class?
last
overload
idnum
add_course
self
first
course
firstname
courses
id
clear_courses
lastname](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7949c58c-a906-41a4-97ee-5d0bfe78cf99%2F70aba321-c58c-45a4-a923-3900344f665f%2Fe49cbf_processed.png&w=3840&q=75)
Transcribed Image Text:class Student:
def _init_(self, firstname, lastname, idnum):
self.first = firstname
self.last = lastname
self.id = idnum
self.courses = []
def add_course(self, course):
''' don't allow overloads or signing up for the same course twice
if course not in self.courses and len(self.courses) < 5:
self.courses.append (course)
def clear_courses(self):
''clear this student's course schedule''"
self.courses = []
def overload (self):
return len(self.courses) >= 4
What are all of the attributes of this class?
last
overload
idnum
add_course
self
first
course
firstname
courses
id
clear_courses
lastname
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
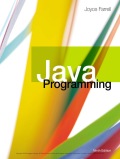
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
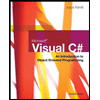
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
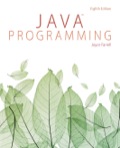
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
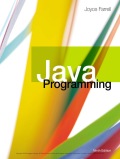
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
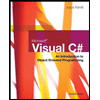
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
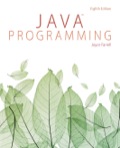
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT