bubblesort.h is in second picture This is bublesort.c int compare(Entry a, Entry b) { // IMPLEMENT } /* TEST: ./mergesort < test.in OUTPUT: 1 lsdfjl 2 ghijk 3 ksdljf 5 abc 6 def */ int main(void) { // IMPLEMENT } this is test.in 5 5 abc 6 def 2 ghijk 3 ksdljf 1 lsdfjl
bubblesort.h is in second picture This is bublesort.c int compare(Entry a, Entry b) { // IMPLEMENT } /* TEST: ./mergesort < test.in OUTPUT: 1 lsdfjl 2 ghijk 3 ksdljf 5 abc 6 def */ int main(void) { // IMPLEMENT } this is test.in 5 5 abc 6 def 2 ghijk 3 ksdljf 1 lsdfjl
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 5RQ
Related questions
Question
bubblesort.h is in second picture
This is bublesort.c
int compare(Entry a, Entry b) {
// IMPLEMENT
}
/*
TEST: ./mergesort < test.in
OUTPUT:
1 lsdfjl
2 ghijk
3 ksdljf
5 abc
6 def
*/
int main(void) {
// IMPLEMENT
}
// IMPLEMENT
}
/*
TEST: ./mergesort < test.in
OUTPUT:
1 lsdfjl
2 ghijk
3 ksdljf
5 abc
6 def
*/
int main(void) {
// IMPLEMENT
}
this is test.in
5
5 abc
6 def
2 ghijk
3 ksdljf
1 lsdfjl
5 abc
6 def
2 ghijk
3 ksdljf
1 lsdfjl

Transcribed Image Text:H
1
2
3
4
LO
bubblesort.h X
#define MAX_NAME_LENGTH 30
↓↑ ↓↑
typedef struct EntryStruct {
int data;
char *name;
5
6 Ⓒ} Entry;
7
8
9
10
void sort (Entry *in, int nL, void *comparator);
int compare (Entry a, Entry b);
![In this task, you will be implementing a simple sorting algorithm Bubble Sort in C. To keep things simple,
we have provided you with the pseudo code; you only need to translate it to C.
Let Entry be a C-struct with the following fields: data (int), name (char *). We have already
defined them for you in bubblesort.h. Entries are sorted by using name as the key (Repeat again, you
are sorting using the string part as the key). Your task is to finish the implementation in sort() that also
takes in a function pointer representing our comparator function: sort and also the main function
main.
• sort - Sort the data based on the input with size n
// input is the input data you want to sort,
// n is the size and
// comparator is the comparator function used by your implementation of bubblesort
void sort (Entry *input, int n, void comparator) {
# Traverse through all array elements
for i from to n:
}
# Last i elements are already in place
for j from to (n-i-1):
main() {
}
.main-The "main" function
#Swap if the element found is greater
#than the next element
if comparator (input[j], input[j+1]) > 0:
swap input[j] and input [j+1]
Read the number of items from the STDIN.
Allocate an array for the input.
Read data from STDIN and store them the array.
Create a comparator function that would allow you to scrt our data from smallest to largest
Call sort() on the array.
Print out the entries.
Free all the memory that you have allocated.
<number of items>
<data_1> <name_1>
<data_2> <name_2>
Input & Output Your program should read input from the standard input. The format of the input is
as follows:
<data_n> <name_n>
where data_i is an int and name_i is a string of length strictly smaller than MAX_NAME_LENGTH
which is defined in mergesort.h. Here is an example input:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff97c0725-689e-40d7-87a0-46553d65cfc3%2F40429926-df2f-40c4-ba78-9023624223ed%2F8yuki6h_processed.png&w=3840&q=75)
Transcribed Image Text:In this task, you will be implementing a simple sorting algorithm Bubble Sort in C. To keep things simple,
we have provided you with the pseudo code; you only need to translate it to C.
Let Entry be a C-struct with the following fields: data (int), name (char *). We have already
defined them for you in bubblesort.h. Entries are sorted by using name as the key (Repeat again, you
are sorting using the string part as the key). Your task is to finish the implementation in sort() that also
takes in a function pointer representing our comparator function: sort and also the main function
main.
• sort - Sort the data based on the input with size n
// input is the input data you want to sort,
// n is the size and
// comparator is the comparator function used by your implementation of bubblesort
void sort (Entry *input, int n, void comparator) {
# Traverse through all array elements
for i from to n:
}
# Last i elements are already in place
for j from to (n-i-1):
main() {
}
.main-The "main" function
#Swap if the element found is greater
#than the next element
if comparator (input[j], input[j+1]) > 0:
swap input[j] and input [j+1]
Read the number of items from the STDIN.
Allocate an array for the input.
Read data from STDIN and store them the array.
Create a comparator function that would allow you to scrt our data from smallest to largest
Call sort() on the array.
Print out the entries.
Free all the memory that you have allocated.
<number of items>
<data_1> <name_1>
<data_2> <name_2>
Input & Output Your program should read input from the standard input. The format of the input is
as follows:
<data_n> <name_n>
where data_i is an int and name_i is a string of length strictly smaller than MAX_NAME_LENGTH
which is defined in mergesort.h. Here is an example input:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
sort function is missing also I want program to recieve standard input so it can run with this command after compile ./bubblesort < test.in
Solution
Follow-up Question
I want
> ./bubblesort < test.in
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
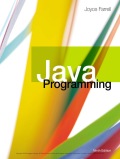
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
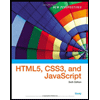
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
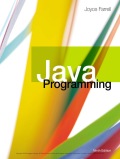
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
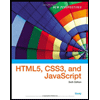
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
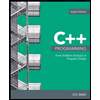
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning