read_file(0, clear_file); // Read in a file with of unknown length, getting the length from the file read. clear_file is a string containing the path to the clear text file. This function returns a char array created with memory allocation. read_file(len, clear_file); // Where len > zero, reads len chars from a file. clear_file is a string containing the path to the clear text file. This function returns a char array created with memory allocation. write_file(0, key, key_file); // Writes a string to file until the \0 char is reached. key is the string of random chars and key_file is a string containing the path of the text file. write_file(len, cipher, cipher_file); // Where len > zero, writes len chars to a file. cipher is the cipher string of chars and cipher_file is a string containing the path of the text file. The menu in main() should look like: Encrypt a file: 1 Decrypt a file: 2 Exit: 3 Enter a choice: I suggest using a loop in main to show the menu, get a choice from the user and a switch statement to execute the chosen function. For the first two choices, the necessary file names should be read by main() and passed to encrypt() and decrypt(). To generate the random key, the length of the key and a char array pointer are passed to make_rand_key(). After make_rand_key() completes, the char array contains the randomly selected chars. When the user chooses to exit the program by entering 3, the loop should exit. The make_rand_key() accepts the length of the random key char array and a pointer to this array. This function uses the srand(time(NULL)) function to seed the rand() function and the rand() function to get random chars. The rand() function accepts no argument and returns an integer value between 0 and RAND_MAX (32,767 on most Intel-based computers). The return from rand must be scaled between 0 and 255 and explicitly castes as a char before being added to the char array. The EOF (integer value of -1) must not be selected as one of the chars in the array so it can be read back from a file during the decrypt operation. If EOF is detected as a random char, subtract 1 from the value to make it -2. It may also be a good idea to replace any null (integer value 0). This may interfere with writing to file and should be replaced with 1. A sample function call to make_rand_key is:
The 2 pictures are the beginning of the lab. The below is for part 2.
- read_file(0, clear_file); // Read in a file with of unknown length, getting the length from the file read. clear_file is a string containing the path to the clear text file. This function returns a char array created with memory allocation.
- read_file(len, clear_file); // Where len > zero, reads len chars from a file. clear_file is a string containing the path to the clear text file. This function returns a char array created with memory allocation.
- write_file(0, key, key_file); // Writes a string to file until the \0 char is reached. key is the string of random chars and key_file is a string containing the path of the text file.
- write_file(len, cipher, cipher_file); // Where len > zero, writes len chars to a file. cipher is the cipher string of chars and cipher_file is a string containing the path of the text file.
The menu in main() should look like:
Encrypt a file: 1
Decrypt a file: 2
Exit: 3
Enter a choice:
I suggest using a loop in main to show the menu, get a choice from the user and a switch statement to execute the chosen function. For the first two choices, the necessary file names should be read by main() and passed to encrypt() and decrypt(). To generate the random key, the length of the key and a char array pointer are passed to make_rand_key(). After make_rand_key() completes, the char array contains the randomly selected chars. When the user chooses to exit the program by entering 3, the loop should exit.
The make_rand_key() accepts the length of the random key char array and a pointer to this array. This function uses the srand(time(NULL)) function to seed the rand() function and the rand() function to get random chars. The rand() function accepts no argument and returns an integer value between 0 and RAND_MAX (32,767 on most Intel-based computers). The return from rand must be scaled between 0 and 255 and explicitly castes as a char before being added to the char array. The EOF (integer value of -1) must not be selected as one of the chars in the array so it can be read back from a file during the decrypt operation. If EOF is detected as a random char, subtract 1 from the value to make it -2. It may also be a good idea to replace any null (integer value 0). This may interfere with writing to file and should be replaced with 1. A sample function call to make_rand_key is:
make_rand_key(len, key); // Where len > zero, generates and returns a random key of len chars.
The encrypt() function accepts the clear text, random key and cipher text file names as arguments. It reads the clear text files, generates a random key, performs XOR on clear text and random key, producing the cipher text, and writes the cipher text to file. Sample function call for encrypt():
encrypt(clear_file, key_file, cipher_file); // Where the three arguments are strings containing filenames.
The decrypt() function accepts the random key, cipher text and cipher text file names as arguments. It reads the random key and cipher text files. Read the random key first using read_file and either getc (in a loop) or fgets to read the cipher text file due to the possibility of a stray EOF in the cipher file. Sample function for decrypt():



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

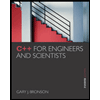
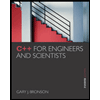