Question: First write a function mulByDigit :: Int -> BigInt -> BigInt which takes an integer digit and a big integer, and returns the big integer list which is the result of multiplying the big integer with the digit. You should get the following behavior: ghci> mulByDigit 9 [9,9,9,9] [8,9,9,9,1] Your implementation should not be recursive. Now, using mulByDigit, fill in the implementation of bigMul :: BigInt -> BigInt -> BigInt Again, you have to fill in implementations for f , base , args only. Once you are done, you should get the following behavior at the prompt: ghci> bigMul [9,9,9,9] [9,9,9,9] [9,9,9,8,0,0,0,1] ghci> bigMul [9,9,9,9,9] [9,9,9,9,9] [9,9,9,9,8,0,0,0,0,1] ghci> bigMul [4,3,7,2] [1,6,3,2,9] [7,1,3,9,0,3,8,8] ghci> bigMul [9,9,9,9] [0] [] Your implementation should not be recursive. Code: import Prelude hiding (replicate, sum)import Data.List (foldl') foldLeft :: (a -> b -> a) -> a -> [b] -> afoldLeft = foldl' ---------------------------------------------------------------------------------- | `mulByDigit i n` returns the result of multiplying-- the digit `i` (between 0..9) with `BigInt` `n`.---- >>> mulByDigit 9 [9,9,9,9]-- [8,9,9,9,1] mulByDigit :: Int -> BigInt -> BigIntmulByDigit i n = error "TBD:mulByDigit" ---------------------------------------------------------------------------------- | `bigMul n1 n2` returns the `BigInt` representing the product of `n1` and `n2`.---- >>> bigMul [9,9,9,9] [9,9,9,9]-- [9,9,9,8,0,0,0,1]---- >>> bigMul [9,9,9,9,9] [9,9,9,9,9]-- [9,9,9,9,8,0,0,0,0,1] bigMul :: BigInt -> BigInt -> BigIntbigMul l1 l2 = res where res = foldLeft f base args f a x = error "TBD:bigMul:f" base = error "TBD:bigMul:base" args = error "TBD:bigMul:args"
Question:
First write a function
which takes an integer digit and a big integer, and returns the big integer list which is the result of multiplying the big integer with the digit. You should get the following behavior:
Your implementation should not be recursive.
Now, using mulByDigit, fill in the implementation of
Again, you have to fill in implementations for f , base , args only. Once you are done, you should get the following behavior at the prompt:
Your implementation should not be recursive.
Code:
import Prelude hiding (replicate, sum)
import Data.List (foldl')
foldLeft :: (a -> b -> a) -> a -> [b] -> a
foldLeft = foldl'
--------------------------------------------------------------------------------
-- | `mulByDigit i n` returns the result of multiplying
-- the digit `i` (between 0..9) with `BigInt` `n`.
--
-- >>> mulByDigit 9 [9,9,9,9]
-- [8,9,9,9,1]
mulByDigit :: Int -> BigInt -> BigInt
mulByDigit i n = error "TBD:mulByDigit"
--------------------------------------------------------------------------------
-- | `bigMul n1 n2` returns the `BigInt` representing the product of `n1` and `n2`.
--
-- >>> bigMul [9,9,9,9] [9,9,9,9]
-- [9,9,9,8,0,0,0,1]
--
-- >>> bigMul [9,9,9,9,9] [9,9,9,9,9]
-- [9,9,9,9,8,0,0,0,0,1]
bigMul :: BigInt -> BigInt -> BigInt
bigMul l1 l2 = res
where
res = foldLeft f base args
f a x = error "TBD:bigMul:f"
base = error "TBD:bigMul:base"
args = error "TBD:bigMul:args"
Unlock instant AI solutions
Tap the button
to generate a solution
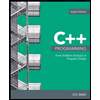
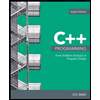