Program Specifications Write a FancyCar class to support basic operations such as drive, add gas, honk horn and start engine. fancy_car.py is provided with function stubs. Follow each step to gradually complete all instance methods. Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main program includes basic calls to the instance methods. Add statements in the main program as instance methods are completed to support development mode testing. **Step 0: Complete the constructor to initialize the model and miles per gallon (MPG) with the values of the parameters. Initialize the odometer to five miles and the tank to be full of gas. By default, the model is initialized to "Old Clunker", and MPG is initialized to 24.0. Note the provided constant variable indicates the gas tank capacity of 14.0 gallons. Step 1 Complete the instance methods to check the odometer, check the gas gauge, get the model, and get the miles per gallon. Submit for grading to confirm 2 tests pass. Step 2 Complete the honk horn() instance method to output the following statement with the car model: The Honda Civic says beep beep! Submit for grading to confirm 3 tests pass. Step 3 Complete the drive() instance method. Miles driven should increase by the distance parameter, and amount of gas should decrease by distance / MPG. Attributes are updated only if distance is positive. Submit for grading to confirm 4 tests pass. Step 4 Complete the add_gas() instance method. Increase gas tank by the amount parameter only if amount is positive, up to a maximum of FULL TANK. Submit for grading to confirm 5 tests pass.
Program Specifications Write a FancyCar class to support basic operations such as drive, add gas, honk horn and start engine. fancy_car.py is provided with function stubs. Follow each step to gradually complete all instance methods. Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. The main program includes basic calls to the instance methods. Add statements in the main program as instance methods are completed to support development mode testing. **Step 0: Complete the constructor to initialize the model and miles per gallon (MPG) with the values of the parameters. Initialize the odometer to five miles and the tank to be full of gas. By default, the model is initialized to "Old Clunker", and MPG is initialized to 24.0. Note the provided constant variable indicates the gas tank capacity of 14.0 gallons. Step 1 Complete the instance methods to check the odometer, check the gas gauge, get the model, and get the miles per gallon. Submit for grading to confirm 2 tests pass. Step 2 Complete the honk horn() instance method to output the following statement with the car model: The Honda Civic says beep beep! Submit for grading to confirm 3 tests pass. Step 3 Complete the drive() instance method. Miles driven should increase by the distance parameter, and amount of gas should decrease by distance / MPG. Attributes are updated only if distance is positive. Submit for grading to confirm 4 tests pass. Step 4 Complete the add_gas() instance method. Increase gas tank by the amount parameter only if amount is positive, up to a maximum of FULL TANK. Submit for grading to confirm 5 tests pass.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 12PE
Related questions
Question

Transcribed Image Text:Program Specifications Write a FancyCar class to support basic operations such as drive, add gas, honk horn and start engine.
fancy_car.py is provided with function stubs. Follow each step to gradually complete all instance methods.
Note: This program is designed for incremental development. Complete each step and submit for grading before starting the next step. Only
a portion of tests pass after each step but confirm progress. The main program includes basic calls to the instance methods. Add
statements in the main program as instance methods are completed to support development mode testing.
**Step 0: Complete the constructor to initialize the model and miles per gallon (MPG) with the values of the parameters. Initialize the
odometer to five miles and the tank to be full of gas. By default, the model is initialized to "Old Clunker", and MPG is initialized to 24.0. Note
the provided constant variable indicates the gas tank capacity of 14.0 gallons.
Step 1 Complete the instance methods to check the odometer, check the gas gauge, get the model, and get the miles per gallon.
Submit for grading to confirm 2 tests pass.
Step 2
Complete the honk horn() instance method to output the following statement with the car model:
The Honda Civic says beep beep!
Submit for grading to confirm 3 tests pass.
Step 3 Complete the drive() instance method. Miles driven should increase by the distance parameter, and amount of gas should
decrease by distance / MPG. Attributes are updated only if distance is positive. Submit for grading to confirm 4 tests pass.
Step 4
Complete the add_gas() instance method. Increase gas tank by the amount parameter only if amount is positive, up to a
maximum of FULL TANK. Submit for grading to confirm 5 tests pass.

Transcribed Image Text:Step 5
Update drive() to determine if the car runs out of gas. If so, the parameter distance will not be achieved and the gas tank will
have 0.0 gallons. Ex drive(100) will not be possible with only three gallons of gas and MPG of 20.0. The maximum driving distance is 60
miles with three gallons of gas and MPG of 20.0. Therefore, the odometer will only increase by 60 instead of the requested 100 and the gas
tank will have 0.0 gallons (not a negative amount). Submit for grading to confirm 6 tests pass.
Step 6 Add a boolean attribute to indicate if the car engine is on or off. 1) Complete the the start engine() instance method to set
the boolean attribute to True. 2) Complete the stop engine() instance method to set the boolean attribute to false. 3) Update the
constructor to start with the engine off. 4) Update drive() to only update attributes if the engine is on, and the engine turns off if the car runs
out of gas, 5) Update add_gas() to only add gas if the engine is off. Submit for grading to confirm all tests pass
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
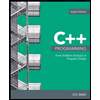
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
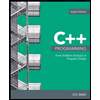
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning