Program Requirements: You will develop a program capable of encrypt and decrypting text using Caesar cipher. In order to do this, you will be required to implement several functions, specified in the template provided. Once complete, your programs main() method should do the following: 1. Prompt users to select a mode (encrypt or decrypt). 2. Check if the mode the user entered is valid. If not, continue to prompt the user until a valid mode is selected. 3. Prompt the user for the message they would like to encrypt or decrypt. 4. Encrypt or decrypt the message as appropriate and print the output. 5. Prompt the user whether they would like to encrypt or decrypt another message. 6. Check if the user has entered a valid input (y/n) If not, continue to prompt the user until they enter a valid response. Depending upon the response you should either: a. End the program if the user selects no. b. Proceed directly to step 2 if the user says yes. You should use a loop to keep the programming running if the user says that he/she would like to encrypt or decrypt more messages. Your program should handle both uppercase and lowercase inputs. You can use str.upper() and str.lower() to convert strings to a specific case. However, you should only output only uppercase messages. (Use the template used in the picture, it is a MUST, or the resolving is not taken in consideration as the exaple says, I need to learn to do it as the template shows , thanks) (Please make it in python, I struggle at some points so a full resolvation made exactly like how the template was done will be spot on, thanks again)
Program Requirements:
You will develop a program capable of encrypt and decrypting text using Caesar cipher. In order
to do this, you will be required to implement several functions, specified in the template
provided.
Once complete, your programs main() method should do the following:
1. Prompt users to select a mode (encrypt or decrypt).
2. Check if the mode the user entered is valid. If not, continue to prompt the user until a
valid mode is selected.
3. Prompt the user for the message they would like to encrypt or decrypt.
4. Encrypt or decrypt the message as appropriate and print the output.
5. Prompt the user whether they would like to encrypt or decrypt another message.
6. Check if the user has entered a valid input (y/n) If not, continue to prompt the user until
they enter a valid response. Depending upon the response you should either:
a. End the program if the user selects no.
b. Proceed directly to step 2 if the user says yes.
You should use a loop to keep the
to encrypt or decrypt more messages.
Your program should handle both uppercase and lowercase inputs. You can use
str.upper() and str.lower() to convert strings to a specific case. However, you should
only output only uppercase messages.
(Use the template used in the picture, it is a MUST, or the resolving is not taken in consideration as the exaple says, I need to learn to do it as the template shows , thanks)
(Please make it in python, I struggle at some points so a full resolvation made exactly like how the template was done will be spot on, thanks again)

![# A Caesar Cipher Program
import os.path
def welcome ():
# add your code here
return
def enter_message():
mode = ''
message =
shift = 0
# add your code here
return (mode, message, shift)
def encrypt (message, shift):
# add your code here.
return
def decrypt (message, shift):
# add your code here
return
def process_file(filename, mode, shift):
list_messages = []
# add your code here
return list_messages
def write_messages (lines):
# add your code here
return
def is file (filename):
return False
def message_or_file():
11
MUST
USE
TEMPLATE
mode=
filename = None
message
None
shift = 0
# add your code here
return (mode, message, filename, shift)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fba87a338-86ce-43b8-9eb9-6a97197ccbb8%2F48ae2240-7a76-40f7-8344-645cacb3bc07%2Fxe21b1_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

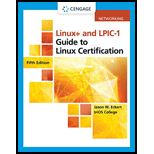
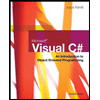
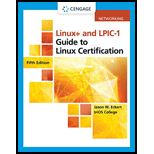
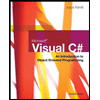
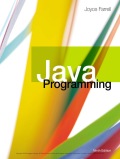
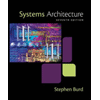