Problem #1 Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console. Ex: The function call: let nums = [4, 2, 9, 1, 8]; H divideArray(nums); produces the console output: Even numbers: 2 4 400 8 Odd numbers: 9 The program should output "None" if no even numbers exist or no odd numbers exist. Ex: The function call: let nums = [4, 2, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: None Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array sort() method a comparison function for sorting numbers correctly. To test your code in your web browser, call divideArray() from the JavaScript console.
Problem #1 Write the function divideArray() in script.js that has a single numbers parameter containing an array of integers. The function should divide numbers into two arrays, evenNums for even numbers and oddNums for odd numbers. Then the function should sort the two arrays and output the array values to the console. Ex: The function call: let nums = [4, 2, 9, 1, 8]; H divideArray(nums); produces the console output: Even numbers: 2 4 400 8 Odd numbers: 9 The program should output "None" if no even numbers exist or no odd numbers exist. Ex: The function call: let nums = [4, 2, 8]; divideArray(nums); produces the console output: Even numbers: 2 4 8 Odd numbers: None Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array sort() method a comparison function for sorting numbers correctly. To test your code in your web browser, call divideArray() from the JavaScript console.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Problem #2
Write a function called playGuessingGame() in script.js that has two parameters:
numToGuess is the number that the user has to guess.
totalGuesses is the total number of times the user is allowed to guess the number. The default value
should be 10.
The playGuessingGame() function should return the number of guesses the user took to enter the
correct number. Ex: If the user guesses the correct number after 3 tries, the function should return 3. If
the user does not guess the correct number before the number of guesses exceeds totalGuesses, the
function should return 0.
The playGuessingGame() function should call the JavaScript prompt() function to read the user's input,
as shown below.
Screenshot of example prompt
This page says
Enter a number between 1 and 100.
5
OK
Cancel
The prompt text should reflect what the user previously entered:
•
If the user is making their first guess, the prompt should read: "Enter a number between 1 and
100."
•
If the user previously guessed a number <numToGuess, the prompt should read "X is too small.
Guess a larger number.", where X is the number previously entered.
•
If the user previously guessed a number> numToGuess, the prompt should read "X is too large.
Guess a smaller number.", where X is the number previously entered.
•
If the user enters an empty string or a string that is not a number, the prompt should read
"Please enter a number." and give the user another chance to enter another number without
losing a turn. Hint: Use isNaN().
If the user presses Cancel, playGuessingGame() should immediately return 0 without prompting
for any more numbers.
For Example:
The function call playGuessingGame(5) allows the user 10 guesses (the default) to guess the number 5.
The function call playGuessingGame(7,3) allows the user 3 guesses to guess the number 7.
To test your code in your web browser, call playGuessingGame() from the JavaScript console.
![Problem #1
Write the function divideArray() in script.js that has a single numbers parameter containing an array of
integers. The function should divide numbers into two arrays, evenNums for even numbers and
oddNums for odd numbers. Then the function should sort the two arrays and output the array values to
the console.
Ex: The function call:
let nums = |
=
divideArray(nums);
produces the console output:
[4, 2, 9, 1, 8];
Even numbers:
2
4
8
Odd numbers:
1
9
The program should output "None" if no even numbers exist or no odd numbers exist.
Ex: The function call:
let nums = [4, 2, 8];
divideArray(nums);
produces the console output:
Even numbers:
2
4
8
Odd numbers:
None
Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array
sort() method a comparison function for sorting numbers correctly.
To test your code in your web browser, call divideArray() from the JavaScript console.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fde785cc7-d8dc-45da-a795-21f941468e52%2F392bcdc3-8741-4815-82e9-45deabea6fa4%2F3lqtr0c_processed.png&w=3840&q=75)
Transcribed Image Text:Problem #1
Write the function divideArray() in script.js that has a single numbers parameter containing an array of
integers. The function should divide numbers into two arrays, evenNums for even numbers and
oddNums for odd numbers. Then the function should sort the two arrays and output the array values to
the console.
Ex: The function call:
let nums = |
=
divideArray(nums);
produces the console output:
[4, 2, 9, 1, 8];
Even numbers:
2
4
8
Odd numbers:
1
9
The program should output "None" if no even numbers exist or no odd numbers exist.
Ex: The function call:
let nums = [4, 2, 8];
divideArray(nums);
produces the console output:
Even numbers:
2
4
8
Odd numbers:
None
Hints: Use the push() method to add numbers to the evenNums and oddNums arrays. Supply the array
sort() method a comparison function for sorting numbers correctly.
To test your code in your web browser, call divideArray() from the JavaScript console.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
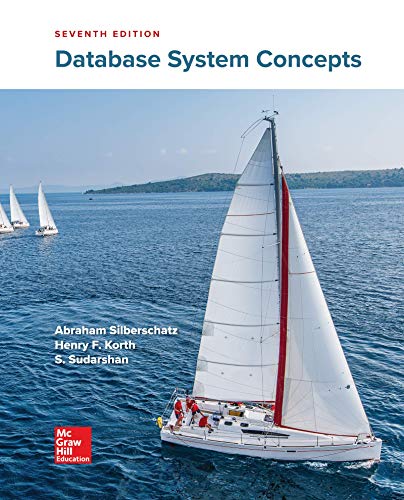
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
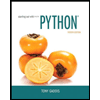
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
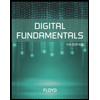
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
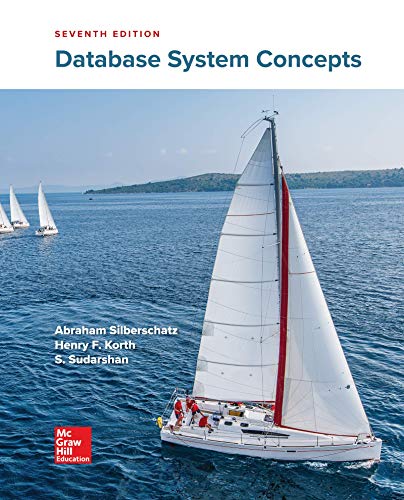
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
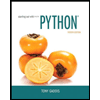
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
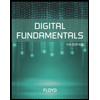
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
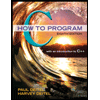
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
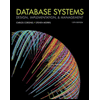
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
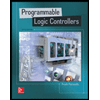
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education