printf("Start Point: "); scanf("%d %d",&MAP_ROWS_S,&MAP_COLUMNS_S); printf("End Point: "); scanf("%d %d",&MAP_ROWS_E,&MAP_COLUMNS_E); map[MAP_ROWS_S][MAP_COLUMNS_S].land = PATH_START; map[MAP_ROWS_E][MAP_COLUMNS_E].land = PATH_END; print_map(map,lives,money); int enemies; printf("Initial Enemies: "); scanf("%d",&enemies); if(enemies > 0){ map[MAP_ROWS_S][MAP_COLUMNS_S].entity = ENEMY; map[MAP_ROWS_S][MAP_COLUMNS_S].n_enemies = enemies; print_map(map,lives,money); }else{ print_map(map,lives,money); } int row,col,height,width; printf("Enter Lake: "); do{ scanf("%d %d %d %d", &row, &col, &height, &width); if (row < 0 || row >=MAP_ROWS || col < 0 || col >=MAP_COLUMNS || row + height >= MAP_ROWS || col + width >= MAP_COLUMNS){ printf("Error: Lake out of bounds, ingorning...\n"); } } while(row < 0 || row >= MAP_ROWS || col < 0 || col >= MAP_COLUMNS || row + height >=MAP_ROWS || col + width >= MAP_COLUMNS); print_map(map,lives,money); } //////////////////////////////////////////////////////////////////////////////// ///////////////////////////// YOUR FUNCTIONS ////////////////////////////////// //////////////////////////////////////////////////////////////////////////////// // TODO: Put your functions here //////////////////////////////////////////////////////////////////////////////// /////////////////////////// PROVIDED FUNCTIONS /////////////////////////////// //////////////////////////////////////////////////////////////////////////////// /** * Initialises map tiles to contain GRASS land and EMPTY entity. * * Parameters: * map - The map to initialise. * Returns: * Nothing. */ void initialise_map(struct tile map[MAP_ROWS][MAP_COLUMNS]) { for (int row = 0; row < MAP_ROWS; ++row) { for (int col = 0; col < MAP_COLUMNS; ++col) { map[row][col].land = GRASS; map[row][col].entity = EMPTY; map[row][col].n_enemies = 0; } } } /** * Prints all map tiles based on their value, with a header displaying lives * and money. * * Parameters: * map - The map to print tiles from. * lives - The number of lives to print with the map. * money - The amount of money to print with the map. * Returns: * Nothing. */ void print_map(struct tile map[MAP_ROWS][MAP_COLUMNS], int lives, int money) { printf("\nLives: %d Money: $%d\n", lives, money); for (int row = 0; row < MAP_ROWS * 2; ++row) { for (int col = 0; col < MAP_COLUMNS; ++col) { print_tile(map[row / 2][col], row % 2); } printf("\n"); } } /** * Prints either the land or entity component of a single tile, based on * the `land_print` parameter; * * Parameters: * tile - The tile to print the land/entity from * land_print - Whether to print the land part of the tile or the entity * part of the tile. If this value is 0, it prints the land, otherwise * it prints the entity. * Returns: * Nothing. */ void print_tile(struct tile tile, int land_print) { if (land_print) { if (tile.land == GRASS) { printf(" . "); } else if (tile.land == WATER) { printf(" ~ "); } else if (tile.land == PATH_START) { printf(" S "); } else if (tile.land == PATH_END) { printf(" E "); } else if (tile.land == PATH_UP) { printf(" ^ "); } else if (tile.land == PATH_RIGHT) { printf(" > "); } else if (tile.land == PATH_DOWN) { printf(" v "); } else if (tile.land == PATH_LEFT) { printf(" < "); } else if (tile.land == TELEPORTER) { printf("( )"); } else { printf(" ? "); } } else { if (tile.entity == EMPTY) { printf(" "); } else if (tile.entity == ENEMY) { printf("%03d", t
printf("Start Point: ");
scanf("%d %d",&MAP_ROWS_S,&MAP_COLUMNS_S);
printf("End Point: ");
scanf("%d %d",&MAP_ROWS_E,&MAP_COLUMNS_E);
map[MAP_ROWS_S][MAP_COLUMNS_S].land = PATH_START;
map[MAP_ROWS_E][MAP_COLUMNS_E].land = PATH_END;
print_map(map,lives,money);
int enemies;
printf("Initial Enemies: ");
scanf("%d",&enemies);
if(enemies > 0){
map[MAP_ROWS_S][MAP_COLUMNS_S].entity = ENEMY;
map[MAP_ROWS_S][MAP_COLUMNS_S].n_enemies = enemies;
print_map(map,lives,money);
}else{
print_map(map,lives,money);
}
int row,col,height,width;
printf("Enter Lake: ");
do{
scanf("%d %d %d %d", &row, &col, &height, &width);
if (row < 0 || row >=MAP_ROWS || col < 0 || col >=MAP_COLUMNS || row + height >= MAP_ROWS || col + width >= MAP_COLUMNS){
printf("Error: Lake out of bounds, ingorning...\n");
}
} while(row < 0 || row >= MAP_ROWS || col < 0 || col >= MAP_COLUMNS || row + height >=MAP_ROWS || col + width >= MAP_COLUMNS);
print_map(map,lives,money);
}
////////////////////////////////////////////////////////////////////////////////
///////////////////////////// YOUR FUNCTIONS //////////////////////////////////
////////////////////////////////////////////////////////////////////////////////
// TODO: Put your functions here
////////////////////////////////////////////////////////////////////////////////
/////////////////////////// PROVIDED FUNCTIONS ///////////////////////////////
////////////////////////////////////////////////////////////////////////////////
/**
* Initialises map tiles to contain GRASS land and EMPTY entity.
*
* Parameters:
* map - The map to initialise.
* Returns:
* Nothing.
*/
void initialise_map(struct tile map[MAP_ROWS][MAP_COLUMNS]) {
for (int row = 0; row < MAP_ROWS; ++row) {
for (int col = 0; col < MAP_COLUMNS; ++col) {
map[row][col].land = GRASS;
map[row][col].entity = EMPTY;
map[row][col].n_enemies = 0;
}
}
}
/**
* Prints all map tiles based on their value, with a header displaying lives
* and money.
*
* Parameters:
* map - The map to print tiles from.
* lives - The number of lives to print with the map.
* money - The amount of money to print with the map.
* Returns:
* Nothing.
*/
void print_map(struct tile map[MAP_ROWS][MAP_COLUMNS], int lives, int money) {
printf("\nLives: %d Money: $%d\n", lives, money);
for (int row = 0; row < MAP_ROWS * 2; ++row) {
for (int col = 0; col < MAP_COLUMNS; ++col) {
print_tile(map[row / 2][col], row % 2);
}
printf("\n");
}
}
/**
* Prints either the land or entity component of a single tile, based on
* the `land_print` parameter;
*
* Parameters:
* tile - The tile to print the land/entity from
* land_print - Whether to print the land part of the tile or the entity
* part of the tile. If this value is 0, it prints the land, otherwise
* it prints the entity.
* Returns:
* Nothing.
*/
void print_tile(struct tile tile, int land_print) {
if (land_print) {
if (tile.land == GRASS) {
printf(" . ");
} else if (tile.land == WATER) {
printf(" ~ ");
} else if (tile.land == PATH_START) {
printf(" S ");
} else if (tile.land == PATH_END) {
printf(" E ");
} else if (tile.land == PATH_UP) {
printf(" ^ ");
} else if (tile.land == PATH_RIGHT) {
printf(" > ");
} else if (tile.land == PATH_DOWN) {
printf(" v ");
} else if (tile.land == PATH_LEFT) {
printf(" < ");
} else if (tile.land == TELEPORTER) {
printf("( )");
} else {
printf(" ? ");
}
} else {
if (tile.entity == EMPTY) {
printf(" ");
} else if (tile.entity == ENEMY) {
printf("%03d", tile.n_enemies);
} else if (tile.entity == BASIC_TOWER) {
printf("[B]");
} else if (tile.entity == POWER_TOWER) {
printf("[P]");
} else if (tile.entity == FORTIFIED_TOWER) {
printf("[F]");
} else {
printf(" ? ");
}
}
}
Need help with outputting the attached picture. The restrictions are already set, however, it does not print the map if the inputs are within restrictions. c coding

![3:42 PM 0.1 KB/s
#include <stdio.h>
#define MAP_ROWS 6
#define MAP_COLUMNS 12
enum land_type {
GRASS,
WATER,
PATH_START,
PATH_END,
PATH_UP,
USER DEFINED TYPES
PATH_RIGHT,
PATH_DOWN,
PATH_LEFT,
TELEPORTER
};
enum entity {
EMPTY,
ENEMY,
BASIC_TOWER,
POWER TOWER,
FORTIFIED_TOWER,
};
struct tile {
enum land_type land;
enum entity entity;
int n_enemies;
};
//////////////////////// YOUR FUNCTION PROTOTYPE
↑
// TODO: Put your function prototypes here
PROVIDED FUNCTION PROTOTYPE
void initialise_map(struct tile map[MAP_ROWS][MAP_COLUMNS]);
void print_map(struct tile map[MAP_ROWS][MAP_COLUMNS], int lives, int money);
void print_tile(struct tile tile, int entity_print);
int main(void) {
// This `map` variable is a 2D array of 'struct tile's.
// It is 'MAP_ROWS' x 'MAP_COLUMNS in size (which is 6x12 for this
int lives;
int money;
// assignment!)
struct tile map[MAP_ROWS][MAP_COLUMNS];
// This will initialise all tiles in the map to have GRASS land and EMPTY
// entity values.
initialise_map(map);
int MAP_ROWS_S;
int MAP_COLUMNS_S;
int MAP_ROWS_E;
int MAP_COLUMNS_E;
// TODO: Start writing code here!
// TODO: Stage 1.1 - Scan in lives, money and start/ending points, then
// print out the map!
printf("Starting Lives: ");
scanf("%d",&lives);
printf("Starting Money($): ");
scanf("%d",&money);
4G
Vo
LTE 4
41](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd0398508-703c-4061-bdaf-d081a740f720%2F3b6e9118-3a85-4507-8862-a5cb193dbf37%2F9n34nsr_processed.jpeg&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

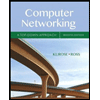
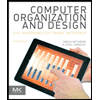
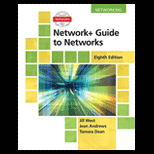
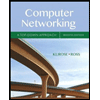
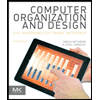
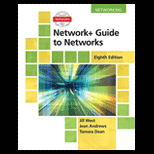
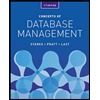
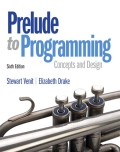
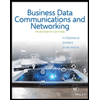