PLEASE HELP! I created this code, however I am having errors in figuring out the issue. It works, no errors but I don't get the correct output as promoted in the instructions. Instructions: This program accepts a single-letter keyboard input at a time. The letter inputs must be lower case a through. Only one letter must be input at a time. - In the data declaration, declare an alphabetized string of letters (named “str”), a-z. -The program must input a character from the keyboard, determine its place in the string, and make a space in the string for this character, inserting it in the proper alphabetical order. - After the alphabetized string of 26 letters, declare a sequence of 30 nulls (using the “.space” directive), as expansion space for the string as you insert characters into the alphabetized list. - At any point, you can ask to print the current string (for example, with as many letter inserts as have been made so far) by inputting capital P from the keyboard. -Finally, this program MUST use a recursive routine to insert the letter in the correct point in the program. --The goal for this program is: Program a recursive algorithm which will place random letters in an already-existing alphabetical list. A single letter will be input from the console at a time, which the program will then insert into a list of alphabetized letters defined in the data declaration. --I am using an online MIPS compiler by jpspim. This program HAS to work with this compiler. If you have any other free compilers besides QTspim let me know. here is the program: .data str: .space 56 # 26 letters (26 * 2 bytes) + 30 nulls (30 * 2 bytes) prompt: .asciiz "Enter a single letter (or 'P' to print): " newline: .asciiz "\n" inserted: .asciiz "\nThe letter has been inserted.\n" .text # Function to print a string print_string: li $v0, 4 # syscall code for print string syscall jr $ra # return # Function to read a single character from input read_char: li $v0, 12 # syscall code for read character syscall jr $ra # return insert_letter: li $t0, 26 # number of characters in the string la $t1, str check_loop: lb $t2, ($t1) # load a byte from the string beqz $t2, insert_letter # branch if the byte is null blt $a1, $t2, shift_loop addi $t1, $t1, 1 # move to the next character in the string addi $a2, $a2, 1 # increment the index j check_loop shift_loop: move $t3, $t1 # $t3 will hold the pointer to the next character addi $t3, $t3, 1 shift_chars: move $t4, $a2 # $t4 holds the current index move $t5, $a0 # $t5 holds the pointer to the string add $t5, $t5, $t4 shift_loop2: lb $t6, ($t5) # load byte from the string sb $t6, 1($t5) # shift the byte to the right sub $t5, $t5, 1 # move to the previous byte bgez $t4, shift_loop2 sb $a1, ($t1) # insert the letter at the current position la $a0, inserted # load message for successful insertion jal print_string # print the success message jr $ra main: li $t3, 26 # counter for the string length la $t4, str # pointer to the string la $t5, inserted input_loop: li $v0, 4 # syscall code for print string la $a0, prompt # load prompt message syscall jal read_char # read a single character from input move $t6, $v0 li $v0, 11 # syscall code for print character move $a0, $t6 # load the character to print syscall li $v0, 4 # syscall code for print string la $a0, newline # load newline character syscall li $t0, 'P' # check if the input is 'P' to print the current string beq $t6, $t0, print_current jal insert_letter # insert the character into the string j input_loop li $v0, 4 # syscall code for print string la $a0, str # load the current string to print syscall j main Double check on the ssspim if the codes does what the prompt says. then post a question.. please
PLEASE HELP! I created this code, however I am having errors in figuring out the issue. It works, no errors but I don't get the correct output as promoted in the instructions.
Instructions: This
- In the data declaration, declare an alphabetized string of letters (named “str”), a-z. -The program must input a character from the keyboard, determine its place in the string, and make a space in the string for this character, inserting it in the proper alphabetical order.
- After the alphabetized string of 26 letters, declare a sequence of 30 nulls (using the “.space” directive), as expansion space for the string as you insert characters into the alphabetized list.
- At any point, you can ask to print the current string (for example, with as many letter inserts as have been made so far) by inputting capital P from the keyboard.
-Finally, this program MUST use a recursive routine to insert the letter in the correct point in the program.
--The goal for this program is: Program a recursive
--I am using an online MIPS compiler by jpspim. This program HAS to work with this compiler. If you have any other free compilers besides QTspim let me know.
here is the program:
.data
str: .space 56 # 26 letters (26 * 2 bytes) + 30 nulls (30 * 2 bytes)
prompt: .asciiz "Enter a single letter (or 'P' to print): "
newline: .asciiz "\n"
inserted: .asciiz "\nThe letter has been inserted.\n"
.text
# Function to print a string
print_string:
li $v0, 4 # syscall code for print string
syscall
jr $ra # return
# Function to read a single character from input
read_char:
li $v0, 12 # syscall code for read character
syscall
jr $ra # return
insert_letter:
li $t0, 26 # number of characters in the string
la $t1, str
check_loop:
lb $t2, ($t1) # load a byte from the string
beqz $t2, insert_letter # branch if the byte is null
blt $a1, $t2, shift_loop
addi $t1, $t1, 1 # move to the next character in the string
addi $a2, $a2, 1 # increment the index
j check_loop
shift_loop:
move $t3, $t1 # $t3 will hold the pointer to the next character
addi $t3, $t3, 1
shift_chars:
move $t4, $a2 # $t4 holds the current index
move $t5, $a0 # $t5 holds the pointer to the string
add $t5, $t5, $t4
shift_loop2:
lb $t6, ($t5) # load byte from the string
sb $t6, 1($t5) # shift the byte to the right
sub $t5, $t5, 1 # move to the previous byte
bgez $t4, shift_loop2
sb $a1, ($t1) # insert the letter at the current position
la $a0, inserted # load message for successful insertion
jal print_string # print the success message
jr $ra
main:
li $t3, 26 # counter for the string length
la $t4, str # pointer to the string
la $t5, inserted
input_loop:
li $v0, 4 # syscall code for print string
la $a0, prompt # load prompt message
syscall
jal read_char # read a single character from input
move $t6, $v0
li $v0, 11 # syscall code for print character
move $a0, $t6 # load the character to print
syscall
li $v0, 4 # syscall code for print string
la $a0, newline # load newline character
syscall
li $t0, 'P' # check if the input is 'P' to print the current string
beq $t6, $t0, print_current
jal insert_letter # insert the character into the string
j input_loop
li $v0, 4 # syscall code for print string
la $a0, str # load the current string to print
syscall
j main
Double check on the ssspim if the codes does what the prompt says. then post a question.. please

Step by step
Solved in 3 steps

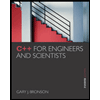
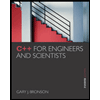