OCaml Code: Please fix all the errors needed for interpreter.ml and use the test cases to test the code. Make sure to give the correct code with the screenshots of the test cases passing the code. interpreter.ml type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT let interpreter (input, output) = let ic = open_in input in let oc = open_out output in let rec loop_read acc = try let l = String.trim (input_line ic) in loop_read (l :: acc) with End_of_file -> List.rev acc in let strList = loop_read [] in let str2sv s = match s with | ":true:" -> BOOL true | ":false:" -> BOOL false | ":error:" -> ERROR | ":unit:" -> UNIT | _ -> let rec try_parse_int str = try INT (int_of_string str) with Failure _ -> try_parse_name str and try_parse_name str = NAME str in try_parse_int s in let str2com s = match s with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv s) in let com2str c = match c with | ADD -> "Add" | SUB -> "Sub" | MUL -> "Mul" | DIV -> "Div" | SWAP -> "Swap" | NEG -> "Neg" | REM -> "Rem" | TOSTRING -> "ToString" | PRINTLN -> "Println" | QUIT -> "Quit" | PUSH sv -> sv2str sv in let sv2str sv = match sv with | BOOL b -> string_of_bool b | INT i -> string_of_int i | ERROR -> ":error:" | STRING s -> s | NAME n -> n | UNIT -> ":unit:" in let rec processor cmds stack = match (cmds, stack) with | (ADD :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a + b) :: restOfStack) | (ADD :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (SUB :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a - b) :: restOfStack) | (SUB :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (MUL :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a * b) :: restOfStack) | (MUL :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (DIV :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a / b) :: restOfStack) | (DIV :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (REM :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a mod b) :: restOfStack) | (REM :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (SWAP :: restOfCommands, x :: y :: restOfStack) -> processor restOfCommands (y :: x :: restOfStack) | (SWAP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (NEG :: restOfCommands, INT a :: restOfStack) -> processor restOfCommands (INT (-a) :: restOfStack) | (NEG :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (TOSTRING :: restOfCommands, x :: restOfStack) -> processor restOfCommands (STRING (sv2str x) :: restOfStack) | (TOSTRING :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (PUSH sv :: restOfCommands, _) -> processor restOfCommands (sv :: stack) | (POP :: restOfCommands, _ :: restOfStack) -> processor restOfCommands restOfStack | (POP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (PRINTLN :: restOfCommands, STRING s :: restOfStack) -> output_string oc (s ^ "\n"); flush oc; processor restOfCommands restOfStack | (PRINTLN :: restOfCommands, _ :: restOfStack) -> processor restOfCommands (ERROR :: stack) | (QUIT :: _, _) -> () | (_, _) -> () in let comList = List.map str2com strList in let _ = processor comList [] in close_in ic; close_out oc Test cases: input1.txt: push 1 toString println quit output1.txt: 1
OCaml Code: Please fix all the errors needed for interpreter.ml and use the test cases to test the code. Make sure to give the correct code with the screenshots of the test cases passing the code. interpreter.ml type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT let interpreter (input, output) = let ic = open_in input in let oc = open_out output in let rec loop_read acc = try let l = String.trim (input_line ic) in loop_read (l :: acc) with End_of_file -> List.rev acc in let strList = loop_read [] in let str2sv s = match s with | ":true:" -> BOOL true | ":false:" -> BOOL false | ":error:" -> ERROR | ":unit:" -> UNIT | _ -> let rec try_parse_int str = try INT (int_of_string str) with Failure _ -> try_parse_name str and try_parse_name str = NAME str in try_parse_int s in let str2com s = match s with | "Add" -> ADD | "Sub" -> SUB | "Mul" -> MUL | "Div" -> DIV | "Swap" -> SWAP | "Neg" -> NEG | "Rem" -> REM | "ToString" -> TOSTRING | "Println" -> PRINTLN | "Quit" -> QUIT | _ -> PUSH (str2sv s) in let com2str c = match c with | ADD -> "Add" | SUB -> "Sub" | MUL -> "Mul" | DIV -> "Div" | SWAP -> "Swap" | NEG -> "Neg" | REM -> "Rem" | TOSTRING -> "ToString" | PRINTLN -> "Println" | QUIT -> "Quit" | PUSH sv -> sv2str sv in let sv2str sv = match sv with | BOOL b -> string_of_bool b | INT i -> string_of_int i | ERROR -> ":error:" | STRING s -> s | NAME n -> n | UNIT -> ":unit:" in let rec processor cmds stack = match (cmds, stack) with | (ADD :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a + b) :: restOfStack) | (ADD :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (SUB :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a - b) :: restOfStack) | (SUB :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (MUL :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a * b) :: restOfStack) | (MUL :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (DIV :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a / b) :: restOfStack) | (DIV :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (REM :: restOfCommands, INT a :: INT b :: restOfStack) -> processor restOfCommands (INT (a mod b) :: restOfStack) | (REM :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (SWAP :: restOfCommands, x :: y :: restOfStack) -> processor restOfCommands (y :: x :: restOfStack) | (SWAP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (NEG :: restOfCommands, INT a :: restOfStack) -> processor restOfCommands (INT (-a) :: restOfStack) | (NEG :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (TOSTRING :: restOfCommands, x :: restOfStack) -> processor restOfCommands (STRING (sv2str x) :: restOfStack) | (TOSTRING :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (PUSH sv :: restOfCommands, _) -> processor restOfCommands (sv :: stack) | (POP :: restOfCommands, _ :: restOfStack) -> processor restOfCommands restOfStack | (POP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack) | (PRINTLN :: restOfCommands, STRING s :: restOfStack) -> output_string oc (s ^ "\n"); flush oc; processor restOfCommands restOfStack | (PRINTLN :: restOfCommands, _ :: restOfStack) -> processor restOfCommands (ERROR :: stack) | (QUIT :: _, _) -> () | (_, _) -> () in let comList = List.map str2com strList in let _ = processor comList [] in close_in ic; close_out oc Test cases: input1.txt: push 1 toString println quit output1.txt: 1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
OCaml Code: Please fix all the errors needed for interpreter.ml and use the test cases to test the code. Make sure to give the correct code with the screenshots of the test cases passing the code.
interpreter.ml
type stackValue = BOOL of bool | INT of int | ERROR | STRING of string | NAME of string | UNIT
type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT
let interpreter (input, output) =
let ic = open_in input in
let oc = open_out output in
let rec loop_read acc =
try
let l = String.trim (input_line ic) in
loop_read (l :: acc)
with
End_of_file -> List.rev acc
in
let strList = loop_read [] in
let str2sv s =
match s with
| ":true:" -> BOOL true
| ":false:" -> BOOL false
| ":error:" -> ERROR
| ":unit:" -> UNIT
| _ ->
let rec try_parse_int str =
try INT (int_of_string str)
with Failure _ -> try_parse_name str
and try_parse_name str = NAME str
in
try_parse_int s
in
let str2com s =
match s with
| "Add" -> ADD
| "Sub" -> SUB
| "Mul" -> MUL
| "Div" -> DIV
| "Swap" -> SWAP
| "Neg" -> NEG
| "Rem" -> REM
| "ToString" -> TOSTRING
| "Println" -> PRINTLN
| "Quit" -> QUIT
| _ -> PUSH (str2sv s)
in
let com2str c =
match c with
| ADD -> "Add"
| SUB -> "Sub"
| MUL -> "Mul"
| DIV -> "Div"
| SWAP -> "Swap"
| NEG -> "Neg"
| REM -> "Rem"
| TOSTRING -> "ToString"
| PRINTLN -> "Println"
| QUIT -> "Quit"
| PUSH sv -> sv2str sv
in
let sv2str sv =
match sv with
| BOOL b -> string_of_bool b
| INT i -> string_of_int i
| ERROR -> ":error:"
| STRING s -> s
| NAME n -> n
| UNIT -> ":unit:"
in
let rec processor cmds stack =
match (cmds, stack) with
| (ADD :: restOfCommands, INT a :: INT b :: restOfStack) ->
processor restOfCommands (INT (a + b) :: restOfStack)
| (ADD :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (SUB :: restOfCommands, INT a :: INT b :: restOfStack) ->
processor restOfCommands (INT (a - b) :: restOfStack)
| (SUB :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (MUL :: restOfCommands, INT a :: INT b :: restOfStack) ->
processor restOfCommands (INT (a * b) :: restOfStack)
| (MUL :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (DIV :: restOfCommands, INT a :: INT b :: restOfStack) ->
processor restOfCommands (INT (a / b) :: restOfStack)
| (DIV :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (REM :: restOfCommands, INT a :: INT b :: restOfStack) ->
processor restOfCommands (INT (a mod b) :: restOfStack)
| (REM :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (SWAP :: restOfCommands, x :: y :: restOfStack) ->
processor restOfCommands (y :: x :: restOfStack)
| (SWAP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (NEG :: restOfCommands, INT a :: restOfStack) ->
processor restOfCommands (INT (-a) :: restOfStack)
| (NEG :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (TOSTRING :: restOfCommands, x :: restOfStack) ->
processor restOfCommands (STRING (sv2str x) :: restOfStack)
| (TOSTRING :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (PUSH sv :: restOfCommands, _) -> processor restOfCommands (sv :: stack)
| (POP :: restOfCommands, _ :: restOfStack) -> processor restOfCommands restOfStack
| (POP :: restOfCommands, _) -> processor restOfCommands (ERROR :: stack)
| (PRINTLN :: restOfCommands, STRING s :: restOfStack) ->
output_string oc (s ^ "\n");
flush oc;
processor restOfCommands restOfStack
| (PRINTLN :: restOfCommands, _ :: restOfStack) ->
processor restOfCommands (ERROR :: stack)
| (QUIT :: _, _) -> ()
| (_, _) -> ()
in
let comList = List.map str2com strList in
let _ = processor comList [] in
close_in ic;
close_out oc
Test cases:
input1.txt:
push 1
toString
println
quit
output1.txt:
1
![ERROR STRING of string | NAME of string
10 type stackValue = BOOL of bool INT of int
11
12 type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT
13
14 let interpreter (input, output) =
15
let ic = open_in input in
16
let oc = open_out output in
17
18
19
20
21
22
23
24
25
26
27
28
29
let rec loop_read acc =
try
let 1 = String.trim (input_line ic) in
loop_read (1 :: acc)
End_of_file -> List.rev acc
in
let strList = loop_read [] in
with
let str2sv s =
match s with
| ":true:" -> BOOL true
input
Compilation failed due to following error(s).
File "main.ml", line 69, characters 17-23:
69 |
| PUSH sv -> sv2str sv
^^^^^^
Error: Unbound value sv2str
UNIT
stderr](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe7ddc10c-4670-40fd-b02c-6a60c5fcc2f2%2F13761889-820d-49e7-9a52-2a580d861394%2Fgpeq7ph_processed.png&w=3840&q=75)
Transcribed Image Text:ERROR STRING of string | NAME of string
10 type stackValue = BOOL of bool INT of int
11
12 type command = ADD | SUB | MUL | DIV | PUSH of stackValue | POP | REM | NEG | TOSTRING | SWAP | PRINTLN | QUIT
13
14 let interpreter (input, output) =
15
let ic = open_in input in
16
let oc = open_out output in
17
18
19
20
21
22
23
24
25
26
27
28
29
let rec loop_read acc =
try
let 1 = String.trim (input_line ic) in
loop_read (1 :: acc)
End_of_file -> List.rev acc
in
let strList = loop_read [] in
with
let str2sv s =
match s with
| ":true:" -> BOOL true
input
Compilation failed due to following error(s).
File "main.ml", line 69, characters 17-23:
69 |
| PUSH sv -> sv2str sv
^^^^^^
Error: Unbound value sv2str
UNIT
stderr
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
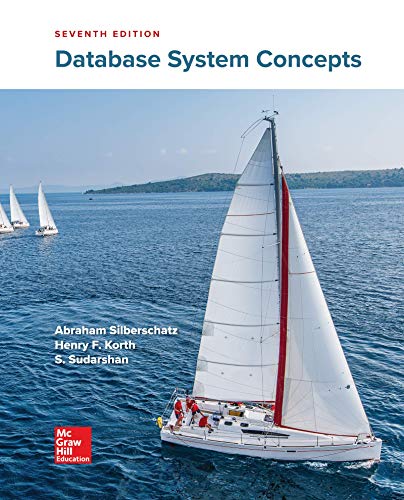
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
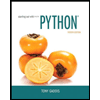
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
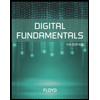
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
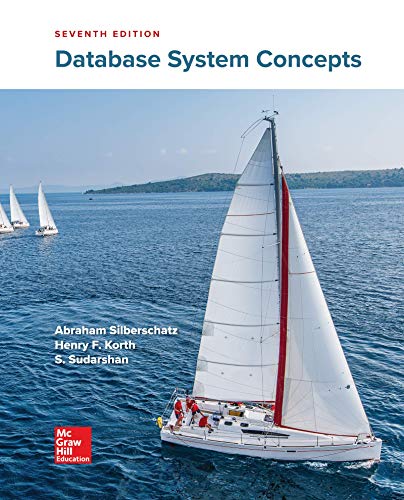
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
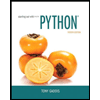
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
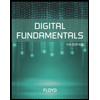
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
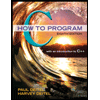
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
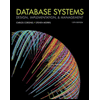
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
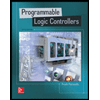
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education