Morse Code Python Part 1: Variable setup and prompt for input • To create the Morse representations, use periods “.” for dots and hyphens “-“ for dashes. For example, the Morse representation for the letter “C” is “-.-.”. A table of the alphabet can be found here. • Create a dictionary where the keys are a single letter (you can choose if it’s upper case or lower case) and the value is a string with the Morse representation of the letter. • Create a loop to prompt the user for input – if they press the enter key, quit the loop. Otherwise, continue to prompt the user for input. Part 2: Convert to visual Morse code • Create an empty string to eventually hold the user’s output in Morse code. • Analyze each character of the user’s input. Since Morse code is capitalization independent the algorithm should work the same for uppercase letters and lowercase letter. I recommend perfecting your code for 1 of the two cases, then fixing it for both cases. • If the user types anything except a letter or a space, ignore the input as it doesn’t belong in the final output. • Visual Morse code relies on spaces between each letter. So after each Morse representation for a letter is added to the output string, add a regular space character in the same manner as you added other strings to the output string. After each word, add 3 space characters to visually separate out the words. • Output for Part 2 should look something like this (user input in red): Input a message to translate into Morse code: SOS, monsters SOS! Your message translated into Morse code is: ... --- ... -- --- -. ... - . .-. ... ... --- ... Input a message to translate into Morse code: The eagle has landed Your message translated into Morse code is: - .... . . .- --. .-.. . .... .- ... .-.. .- -. -.. . -.. Your message translated into Morse code is: Goodbye! Part 3: Play Morse sounds • Ask the user if they’d like to hear their message. • To convert the user’s visual Morse code message to audio Morse code, you will need to... o First initialize the Morse code sound generation system with the following function call: initMorse(); o Process each character of the Morse code string you displayed in part 2. o For each character in the displayed Morse code, every time a dot is encountered call the function addMorseDot(); o For each character in the displayed Morse code, every time a dash is encountered call the function addMorseDash(); o For each character in the displayed Morse code, every time a space is encountered call the function addMorsePause(); o When finished processing the displayed Morse code, call the function: playMorse();
Morse Code Python
Part 1: Variable setup and prompt for input
• To create the Morse representations, use periods “.” for dots and hyphens “-“ for dashes. For
example, the Morse representation for the letter “C” is “-.-.”. A table of the alphabet can be
found here.
• Create a dictionary where the keys are a single letter (you can choose if it’s upper case or lower
case) and the value is a string with the Morse representation of the letter.
• Create a loop to prompt the user for input – if they press the enter key, quit the loop.
Otherwise, continue to prompt the user for input.
Part 2: Convert to visual Morse code
• Create an empty string to eventually hold the user’s output in Morse code.
• Analyze each character of the user’s input. Since Morse code is capitalization independent the
algorithm should work the same for uppercase letters and lowercase letter. I recommend
perfecting your code for 1 of the two cases, then fixing it for both cases.
• If the user types anything except a letter or a space, ignore the input as it doesn’t belong in the
final output.
• Visual Morse code relies on spaces between each letter. So after each Morse representation for
a letter is added to the output string, add a regular space character in the same manner as you
added other strings to the output string. After each word, add 3 space characters to visually
separate out the words.
• Output for Part 2 should look something like this (user input in red):
Input a message to translate into Morse code: SOS, monsters SOS!
Your message translated into Morse code is:
... --- ... -- --- -. ... - . .-. ... ... --- ...
Input a message to translate into Morse code: The eagle has landed
Your message translated into Morse code is:
- .... . . .- --. .-.. . .... .- ... .-.. .- -. -.. . -..
Your message translated into Morse code is:
Goodbye!
Part 3: Play Morse sounds
• Ask the user if they’d like to hear their message.
• To convert the user’s visual Morse code message to audio Morse code, you will need to...
o First initialize the Morse code sound generation system with the following function call:
initMorse();
o Process each character of the Morse code string you displayed in part 2.
o For each character in the displayed Morse code, every time a dot is encountered call the
function
addMorseDot();
o For each character in the displayed Morse code, every time a dash is encountered call
the function
addMorseDash();
o For each character in the displayed Morse code, every time a space is encountered call
the function
addMorsePause();
o When finished processing the displayed Morse code, call the function:
playMorse();

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

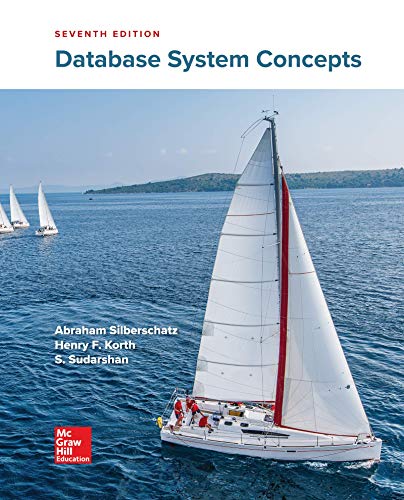
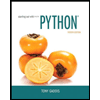
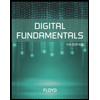
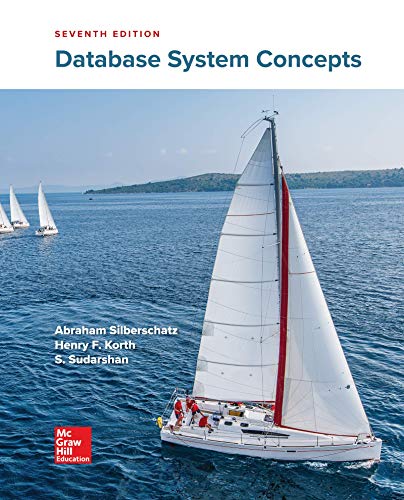
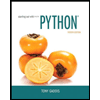
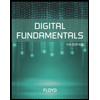
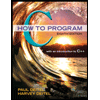
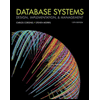
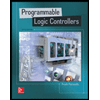