List and indexing practice. Create a list containing all even numbers up to and including 100. Store the list in a variable called even_list. Try using Pythons range() function (docs)and then casting it as a list using list(). Check the length of the even_list. Store the output into the even_list_length variable. Use list indexing to return the last 5 elements of the even_list. Store the output into last_five_even_elements. Use list indexing to return the second to last element of the even_list. Store the output into second_to_last_even_element. Use list indexing to return the first 10 elements of the even_list. Store the output into first_ten_even_elements. # TODO 5.1 even_list = print(f"even_list output: {even_list}") todo_check([ (even_list == [2,4,6,8,10,12,14,16,18,20,22,24,26,28,30,32,34,36,38,40,42,44,46,48,50,52,54,56,58,60,62,64,66,68,70,72,74,76,78,80,82,84,86,88,90,92,94,96,98,100], 'even_list does not match the desired output! Make sure you have even numbers from 2 to and including 100!') ]) # TODO 5.2 even_list_length = print(f"even_list_length output: {even_list_length}") todo_check([ (even_list_length == 50,'even_list_length should be 50') ]) # TODO 5.3 last_five_even_elements = print(f"last_five_even_elements output: {last_five_even_elements}") todo_check([ (last_five_even_elements == [92,94,96,98,100],'last_five_even_elements does not match [92, 94, 96, 98, 100]'), ]) # TODO 5.4 second_to_last_even_element = print(f"second_to_last_even_element output: {second_to_last_even_element}") todo_check([ (second_to_last_even_element == 98,"second_to_last_even_element should be 98") ]) # TODO 5.5 first_ten_even_elements = print(f"first_ten_even_elements output: {first_ten_even_elements}") todo_check([ (first_ten_even_elements == [2,4,6,8,10,12,14,16,18,20], "first_ten_even_elements should be [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]") ])
List and indexing practice. Create a list containing all even numbers up to and including 100. Store the list in a variable called even_list. Try using Pythons range() function (docs)and then casting it as a list using list(). Check the length of the even_list. Store the output into the even_list_length variable. Use list indexing to return the last 5 elements of the even_list. Store the output into last_five_even_elements. Use list indexing to return the second to last element of the even_list. Store the output into second_to_last_even_element. Use list indexing to return the first 10 elements of the even_list. Store the output into first_ten_even_elements. # TODO 5.1 even_list = print(f"even_list output: {even_list}") todo_check([ (even_list == [2,4,6,8,10,12,14,16,18,20,22,24,26,28,30,32,34,36,38,40,42,44,46,48,50,52,54,56,58,60,62,64,66,68,70,72,74,76,78,80,82,84,86,88,90,92,94,96,98,100], 'even_list does not match the desired output! Make sure you have even numbers from 2 to and including 100!') ]) # TODO 5.2 even_list_length = print(f"even_list_length output: {even_list_length}") todo_check([ (even_list_length == 50,'even_list_length should be 50') ]) # TODO 5.3 last_five_even_elements = print(f"last_five_even_elements output: {last_five_even_elements}") todo_check([ (last_five_even_elements == [92,94,96,98,100],'last_five_even_elements does not match [92, 94, 96, 98, 100]'), ]) # TODO 5.4 second_to_last_even_element = print(f"second_to_last_even_element output: {second_to_last_even_element}") todo_check([ (second_to_last_even_element == 98,"second_to_last_even_element should be 98") ]) # TODO 5.5 first_ten_even_elements = print(f"first_ten_even_elements output: {first_ten_even_elements}") todo_check([ (first_ten_even_elements == [2,4,6,8,10,12,14,16,18,20], "first_ten_even_elements should be [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]") ])
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
TODO 5
List and indexing practice.
- Create a list containing all even numbers up to and including 100. Store the list in a variable called even_list. Try using Pythons range() function (docs)and then casting it as a list using list().
- Check the length of the even_list. Store the output into the even_list_length variable.
- Use list indexing to return the last 5 elements of the even_list. Store the output into last_five_even_elements.
- Use list indexing to return the second to last element of the even_list. Store the output into second_to_last_even_element.
- Use list indexing to return the first 10 elements of the even_list. Store the output into first_ten_even_elements.
# TODO 5.1
even_list =
print(f"even_list output: {even_list}")
todo_check([
(even_list == [2,4,6,8,10,12,14,16,18,20,22,24,26,28,30,32,34,36,38,40,42,44,46,48,50,52,54,56,58,60,62,64,66,68,70,72,74,76,78,80,82,84,86,88,90,92,94,96,98,100],
'even_list does not match the desired output! Make sure you have even numbers from 2 to and including 100!')
])
# TODO 5.2
even_list_length =
print(f"even_list_length output: {even_list_length}")
todo_check([
(even_list_length == 50,'even_list_length should be 50')
])
# TODO 5.3
last_five_even_elements =
print(f"last_five_even_elements output: {last_five_even_elements}")
todo_check([
(last_five_even_elements == [92,94,96,98,100],'last_five_even_elements does not match [92, 94, 96, 98, 100]'),
])
# TODO 5.4
second_to_last_even_element =
print(f"second_to_last_even_element output: {second_to_last_even_element}")
todo_check([
(second_to_last_even_element == 98,"second_to_last_even_element should be 98")
])
# TODO 5.5
first_ten_even_elements =
print(f"first_ten_even_elements output: {first_ten_even_elements}")
todo_check([
(first_ten_even_elements == [2,4,6,8,10,12,14,16,18,20],
"first_ten_even_elements should be [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]")
])
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
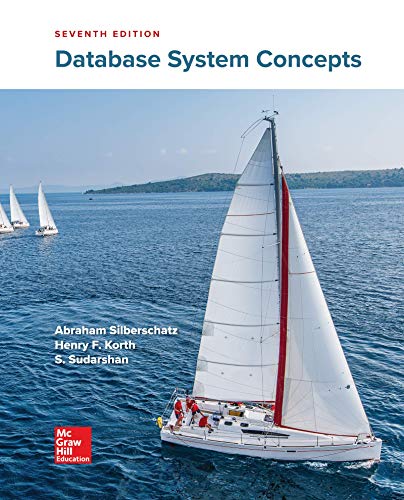
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
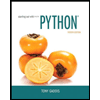
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
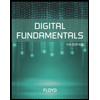
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
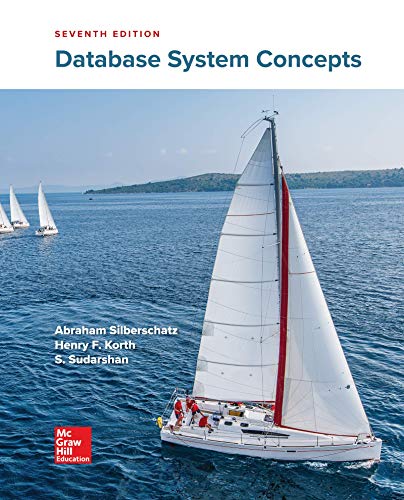
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
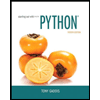
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
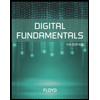
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
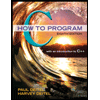
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
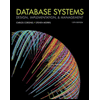
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
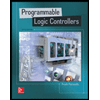
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education