// lab8ExB.cpp #include using namespace std; void insertion_sort(int *int_array, int n); /* REQUIRES * n > 0. * Array elements int_array[0] ... int_array[n - 1] exist. * PROMISES * Element values are rearranged in non-decreasing order. */ void insertion_sort(const char** str_array, int n); /* REQUIRES * n > 0. * Array elements str_array[0] ... str_array[n - 1] exist. * PROMISES * pointers in str_array are rearranged so that strings: * str_array[0] points to a string with the smallest string (lexicographicall) , * str_array[1] points to the second smallest string, ..., str_array[n-2] * points to the second largest, and str_array[n-1] points to the largest string */ int main(void) { const char* s[] = { "AB", "XY", "EZ"}; const char** z = s; z += 1; cout << "The value of **z is: " << **z << endl; cout << "The value of *z is: " << *z << endl; cout << "The value of **(z-1) is: " << **(z-1)<< endl; cout << "The value of *(z-1) is: " << *(z-1)<< endl; cout << "The value of z[1][1] is: " << z[1][1]<< endl; cout << "The value of *(*(z+1)+1) is: " << *(*(z+1)+1)<< endl; // point 1 int a[] = { 413, 282, 660, 171, 308, 537 }; int i; int n_elements = sizeof(a) / sizeof(int); cout << "Here is your array of integers before sorting: \n"; for(i = 0; i < n_elements; i++) cout << a[i] << endl; cout << endl; insertion_sort(a, n_elements); cout << "Here is your array of ints after sorting: \n" ; for(i = 0; i < n_elements; i++) cout << a[i] << endl; #if 0 const char* strings[] = { "Red", "Blue", "pink","apple", "almond","white", "nut", "Law", "cup"}; n_elements = sizeof(strings) / sizeof(char*); cout << "\nHere is your array of strings before sorting: \n"; for(i = 0; i < n_elements; i++) cout << strings[i] << endl; cout << endl; insertion_sort(strings, 9); cout << "Here is your array of strings after sorting: \n" ; for(i = 0; i < n_elements; i++) cout << strings[i] << endl; cout << endl; #endif return 0; } void insertion_sort(int *a, int n) { int i; int j; int value_to_insert; for (i = 1; i < n; i++) { value_to_insert = a[i]; /* Shift values greater than value_to_insert. */ j = i; while ( j > 0 && a[j - 1] > value_to_insert ) { a[j] = a[j - 1]; j--; } a[j] = value_to_insert; } }
// lab8ExB.cpp
#include <iostream>
using namespace std;
void insertion_sort(int *int_array, int n);
/* REQUIRES
* n > 0.
* Array elements int_array[0] ... int_array[n - 1] exist.
* PROMISES
* Element values are rearranged in non-decreasing order.
*/
void insertion_sort(const char** str_array, int n);
/* REQUIRES
* n > 0.
* Array elements str_array[0] ... str_array[n - 1] exist.
* PROMISES
* pointers in str_array are rearranged so that strings:
* str_array[0] points to a string with the smallest string (lexicographicall) ,
* str_array[1] points to the second smallest string, ..., str_array[n-2]
* points to the second largest, and str_array[n-1] points to the largest string
*/
int main(void)
{
const char* s[] = { "AB", "XY", "EZ"};
const char** z = s;
z += 1;
cout << "The value of **z is: " << **z << endl;
cout << "The value of *z is: " << *z << endl;
cout << "The value of **(z-1) is: " << **(z-1)<< endl;
cout << "The value of *(z-1) is: " << *(z-1)<< endl;
cout << "The value of z[1][1] is: " << z[1][1]<< endl;
cout << "The value of *(*(z+1)+1) is: " << *(*(z+1)+1)<< endl;
// point 1
int a[] = { 413, 282, 660, 171, 308, 537 };
int i;
int n_elements = sizeof(a) / sizeof(int);
cout << "Here is your array of integers before sorting: \n";
for(i = 0; i < n_elements; i++)
cout << a[i] << endl;
cout << endl;
insertion_sort(a, n_elements);
cout << "Here is your array of ints after sorting: \n" ;
for(i = 0; i < n_elements; i++)
cout << a[i] << endl;
#if 0
const char* strings[] = { "Red", "Blue", "pink","apple", "almond","white",
"nut", "Law", "cup"};
n_elements = sizeof(strings) / sizeof(char*);
cout << "\nHere is your array of strings before sorting: \n";
for(i = 0; i < n_elements; i++)
cout << strings[i] << endl;
cout << endl;
insertion_sort(strings, 9);
cout << "Here is your array of strings after sorting: \n" ;
for(i = 0; i < n_elements; i++)
cout << strings[i] << endl;
cout << endl;
#endif
return 0;
}
void insertion_sort(int *a, int n)
{
int i;
int j;
int value_to_insert;
for (i = 1; i < n; i++) {
value_to_insert = a[i];
/* Shift values greater than value_to_insert. */
j = i;
while ( j > 0 && a[j - 1] > value_to_insert ) {
a[j] = a[j - 1];
j--;
}
a[j] = value_to_insert;
}
}
![Exercise B: Working with Array of Pointers (UNGRADED)
What to Do:
1. Predict what is the program output at point one.
2. Compile and run the program to find out if your prediction is correct.
3. Read the function interface comment and the definition of function
insertion_sort, which sorts an array of n integers. Its function prototype is
as follows:
void insertion_sort (int *int_array, int n);
5. Change the pre-processor directive #if 0 to #if 1 and write the
definition of the other overloaded
definition of insertion_sort with the following prototype:
void insertion sort (const char** str_array, int n);
The first argument of this function is a pointer that points to an array of n C-
strings. The job of the
function is to rearrange the pointers in str_array in a way that,
lexicographically: str_array [0]
smallest,
points to the smallest string, str_array[1] points to the second
str_array[n-2] points to second largest, and finally
str_array[n-1] points to the largest string.
The following code segment shows the concept of rearranging the pointers in
an array of pointers, and
referring to their target strings in a non-decreasing order:
.../
const char* str_array[3] = {“xyz”, “klm”, “abc”}
const char* tmp = str_array[o];
str_array[o] = str_array[2];
str_array[2] = tmp;
for(int j=0; j < 3; j++)
cout << str[i] << endl;
And, here is the output of this code segment:
abc
klm
xyz](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F008f31aa-ffde-472d-81b9-49fb75f4a0c2%2Fa4ea8177-c0b8-413b-88ea-22eaaa12493f%2F2pueg8p_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 3 images

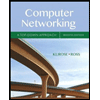
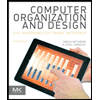
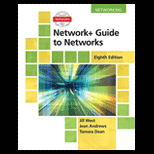
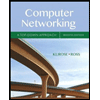
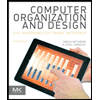
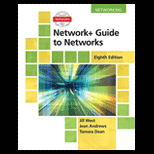
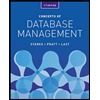
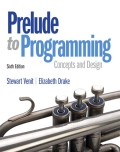
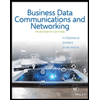