Lab8: Vector merge CS-102 2020 Fall Semester E6.20 (Big C++ Chapter 6 – Arrays & Vector Exercise E6.20) Write a function vector merge_sorted(vector a, vector b) that merges two sorted vectors, producing a new sorted vector. Keep an index into each vector, indicating how much of it has been processed already. Each time, append the smallest unprocessed element from either vector, then advance the index. For example, if a is 0 1 49 16 25 36 and b is 4 5 8 13 20 then merge_sorted returns the vector 0 1 4 4 5 89 13 16 20 25 36
Lab8: Vector merge CS-102 2020 Fall Semester E6.20 (Big C++ Chapter 6 – Arrays & Vector Exercise E6.20) Write a function vector merge_sorted(vector a, vector b) that merges two sorted vectors, producing a new sorted vector. Keep an index into each vector, indicating how much of it has been processed already. Each time, append the smallest unprocessed element from either vector, then advance the index. For example, if a is 0 1 49 16 25 36 and b is 4 5 8 13 20 then merge_sorted returns the vector 0 1 4 4 5 89 13 16 20 25 36
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter16: Searching, Sorting And Vector Type
Section: Chapter Questions
Problem 24SA
Related questions
Question
The
help me write a program.
![Lab8: Vector merge
CS-102
2020 Fall Semester
E6.20 (Big C++ Chapter 6 - Arrays & Vector Exercise E6.20)
Write a function
vector<int> merge_sorted(vector<init> a, vector<int> b)
that merges two sorted vectors, producing a new sorted vector. Keep an index into each vector,
indicating how much of it has been processed already. Each time, append the smallest
unprocessed element from either vector, then advance the index.
For example, if a is
0 1 49 16 25 36
and b is
4 5 8 13 20
then merge_sorted returns the vector
0 1 4 4 5 8 9 13 16 20 25 36
Test cases:
o Assign int value 0 1 4 9 16 25 36 to vector <int> a (7) using a for loop where the
value is the product of the index. For example:
For ( index = 0; index < a.size (); index ++)
a[index] = index * index;
cout << a[index] << “ “;
}
O Assign int value 4 5 8 13 20 to vector <int> b (4) using a for loop where the value
is the the current for loop index * index + 4 (see example above). For example, if
index is 0:0 * 0 + 4 = 4; if index is 1 then 1 * 1+ 4 = 5; if index is 2 then 2 * 2 + 4 = 8
o After you call the function:
vector < int > c = merge_sorted (a, b);
vector c would have the following value:
0 14 4 5 8 9 13 16 20 25 36](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa0f860c2-5561-4733-a576-f630bdcf959c%2F372fa3b4-4ba3-4d65-bd2b-68e9ab9001fb%2Ftks492e_processed.png&w=3840&q=75)
Transcribed Image Text:Lab8: Vector merge
CS-102
2020 Fall Semester
E6.20 (Big C++ Chapter 6 - Arrays & Vector Exercise E6.20)
Write a function
vector<int> merge_sorted(vector<init> a, vector<int> b)
that merges two sorted vectors, producing a new sorted vector. Keep an index into each vector,
indicating how much of it has been processed already. Each time, append the smallest
unprocessed element from either vector, then advance the index.
For example, if a is
0 1 49 16 25 36
and b is
4 5 8 13 20
then merge_sorted returns the vector
0 1 4 4 5 8 9 13 16 20 25 36
Test cases:
o Assign int value 0 1 4 9 16 25 36 to vector <int> a (7) using a for loop where the
value is the product of the index. For example:
For ( index = 0; index < a.size (); index ++)
a[index] = index * index;
cout << a[index] << “ “;
}
O Assign int value 4 5 8 13 20 to vector <int> b (4) using a for loop where the value
is the the current for loop index * index + 4 (see example above). For example, if
index is 0:0 * 0 + 4 = 4; if index is 1 then 1 * 1+ 4 = 5; if index is 2 then 2 * 2 + 4 = 8
o After you call the function:
vector < int > c = merge_sorted (a, b);
vector c would have the following value:
0 14 4 5 8 9 13 16 20 25 36

Transcribed Image Text:Your program output should be similar to below.
Vector a:
e 1 4 9 16 25 36
Vector b:
4 5 8 13 20
Result of interleaved merge of sorted a and b is
e 1 4 4 5 8 9 13 16 20 25 36
Lab Submission: See the lab submission requirements published in canvas.
To submit your assignment in canvas, you must submit TWO files (one pdf
and one zip) as follows:
1. Attach pdt file which contains source codes you have written and program output
screenshot so I can easily read in one file. You can use a word editor to place all the required
programs and screenshots, and then use 'Print' to 'Microsoft Print to PDF' to save to a pdf file
Print
Copies
Print
Printer
Microsoft Print to PDF
Ready
The pdf file MUST have the following sections (1. Program Description, 2. Program Source
Code and 3. Program Output).
1. Program Description
• brief description of the purpose of the program and
an explanation of what your software does and what problem it solves
2. Program Source Code
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
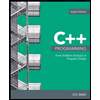
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
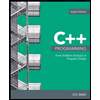
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning