Java Program Fix this Rock, Paper and scissor program so I can upload it to Hypergrade and it can pass all the test cases. Here is the program: import java.util.Random; import java.util.Scanner; public class RockPaperScissors { public static void main(String[] args) { if (args.length != 1) { System.out.println("Please provide a seed as a command line argument."); return; } long seed = Long.parseLong(args[0]); Random random = new Random(seed); Scanner scanner = new Scanner(System.in); System.out.println("Enter 1 for rock, 2 for paper, and 3 for scissors."); do { int computerChoice = random.nextInt(3); int userChoice = getUserChoice(scanner); if (userChoice == -1) { continue; } System.out.println("Your choice: " + choiceToString(userChoice) + ". Computer choice: " + choiceToString(computerChoice) + "."); int result = determineWinner(userChoice, computerChoice); if (result == 0) { System.out.println("It's a draw."); } else if (result == 1) { System.out.println("Computer wins."); } else { System.out.println("You win."); } System.out.println("Would you like to play more? (yes/no)"); } while (scanner.next().equalsIgnoreCase("yes")); System.out.println("Thanks for playing!"); scanner.close(); } public static int getUserChoice(Scanner scanner) { int choice; while (true) { System.out.print("Enter your choice: "); if (scanner.hasNextInt()) { choice = scanner.nextInt(); if (choice >= 1 && choice <= 3) { break; } else { System.out.println("Please respond 1, 2, or 3."); } } else { scanner.next(); // Consume invalid input System.out.println("Please respond 1, 2, or 3."); } } return choice - 1; // Map to 0, 1, 2 internally } public static String choiceToString(int choice) { switch (choice) { case 0: return "rock"; case 1: return "paper"; case 2: return "scissors"; default: return "invalid"; } } public static int determineWinner(int userChoice, int computerChoice) { if (userChoice == computerChoice) { return 0; // Draw } else if ((userChoice + 1) % 3 == computerChoice) { return 1; // Computer wins } else { return -1; // User wins } } } Test Case 1 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 1ENTER Your choice: rock. Computer choice: paper.\n Computer wins.\n Would you like to play more?\n nENTER Test Case 2 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 2ENTER Your choice: paper. Computer choice: paper.\n It's a draw.\n Would you like to play more?\n nENTER Test Case 3 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 3ENTER Your choice: scissors. Computer choice: paper.\n You win.\n Would you like to play more?\n nENTER Test Case 4 Command Line arguments: 123456789 Enter 1 for rock, 2 for paper, and 3 for scissors.\n 0ENTER Please respond 1, 2, or 3.\n Enter 1 for rock, 2 for paper, and 3 for scissors.\n 1ENTER Your choice: rock. Computer choice: paper.\n Computer wins.\n Would you like to play more?\n nENTER
Java Program
Fix this Rock, Paper and scissor program so I can upload it to Hypergrade and it can pass all the test cases.
Here is the program:
import java.util.Random;
import java.util.Scanner;
public class RockPaperScissors {
public static void main(String[] args) {
if (args.length != 1) {
System.out.println("Please provide a seed as a command line argument.");
return;
}
long seed = Long.parseLong(args[0]);
Random random = new Random(seed);
Scanner scanner = new Scanner(System.in);
System.out.println("Enter 1 for rock, 2 for paper, and 3 for scissors.");
do {
int computerChoice = random.nextInt(3);
int userChoice = getUserChoice(scanner);
if (userChoice == -1) {
continue;
}
System.out.println("Your choice: " + choiceToString(userChoice) + ". Computer choice: " + choiceToString(computerChoice) + ".");
int result = determineWinner(userChoice, computerChoice);
if (result == 0) {
System.out.println("It's a draw.");
} else if (result == 1) {
System.out.println("Computer wins.");
} else {
System.out.println("You win.");
}
System.out.println("Would you like to play more? (yes/no)");
} while (scanner.next().equalsIgnoreCase("yes"));
System.out.println("Thanks for playing!");
scanner.close();
}
public static int getUserChoice(Scanner scanner) {
int choice;
while (true) {
System.out.print("Enter your choice: ");
if (scanner.hasNextInt()) {
choice = scanner.nextInt();
if (choice >= 1 && choice <= 3) {
break;
} else {
System.out.println("Please respond 1, 2, or 3.");
}
} else {
scanner.next(); // Consume invalid input
System.out.println("Please respond 1, 2, or 3.");
}
}
return choice - 1; // Map to 0, 1, 2 internally
}
public static String choiceToString(int choice) {
switch (choice) {
case 0:
return "rock";
case 1:
return "paper";
case 2:
return "scissors";
default:
return "invalid";
}
}
public static int determineWinner(int userChoice, int computerChoice) {
if (userChoice == computerChoice) {
return 0; // Draw
} else if ((userChoice + 1) % 3 == computerChoice) {
return 1; // Computer wins
} else {
return -1; // User wins
}
}
}
Test Case 1
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER
Test Case 2
2ENTER
Your choice: paper. Computer choice: paper.\n
It's a draw.\n
Would you like to play more?\n
nENTER
Test Case 3
3ENTER
Your choice: scissors. Computer choice: paper.\n
You win.\n
Would you like to play more?\n
nENTER
Test Case 4
0ENTER
Please respond 1, 2, or 3.\n
Enter 1 for rock, 2 for paper, and 3 for scissors.\n
1ENTER
Your choice: rock. Computer choice: paper.\n
Computer wins.\n
Would you like to play more?\n
nENTER

Step by step
Solved in 4 steps with 3 images

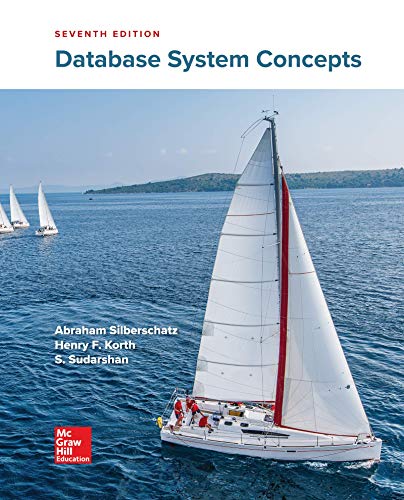
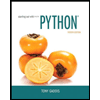
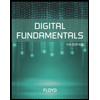
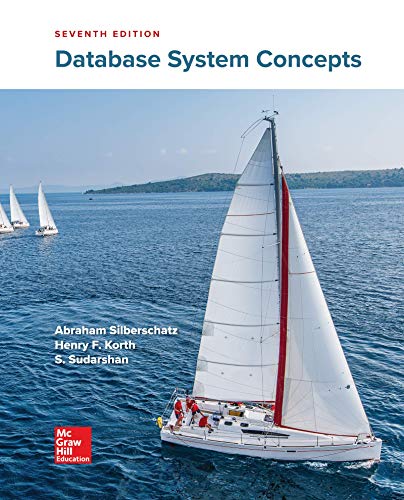
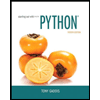
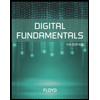
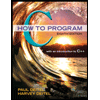
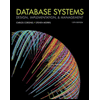
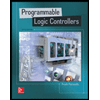