Java Only Do Method, I will give LinkedList Implementation Write a method checkForEquality to be included in class KWLinkedList that does not have any parameter. Assume the list is having even number of nodes. The method returns true if the first half of the list is equal to the second half of the list in the reverse order, otherwise it returns false. If the list is empty, the method returns false; import java.util.NoSuchElementException; /** Implementation of the interface StackInt using a linked list. The * top element of the stack is in the first (front) node of the linked list. */ public class LinkedStack implements StackInt { /** Node: inner class to create nodes for linked list based stack. */ private static class Node { // Data Fields private E data; private Node next; // The reference to the next node. // Constructors /** * Creates a new node with a null next field. * @param dataItem The data to be stored in the node */ private Node(E dataItem) { data = dataItem; next = null; } /** * Creates a new node that references another node. * @param dataItem The data to be stored in the node * @param nodeRef The node referenced by new node */ private Node(E dataItem, Node nodeRef) { data = dataItem; next = nodeRef; } } //End of class Node // Data Field: The reference to the first node of the stack. private Node topOfStack; // Constructor public LinkedStack() { topOfStack = null; // Initially the stack is empty } // copy constructor public LinkedStack(LinkedStack other) { if(other.isEmpty()) tofOfStack=null; else { Node newNode = new Node (other.topOfStack.data); topOfStack = newNode; Node ptr= other.topOdStack.next; Node ptr1 = topOdStack; while (ptr != null) { newNode = new Node(ptr.data); ptr1.next=newNode; ptr = ptr.next; ptr1=ptr1.next; } } } /** * Insert a new item on top of the stack. * @post The new item is the top item on the stack. * @param obj The item to be inserted * @return The item that was inserted */ @Override public E push(E obj) { topOfStack = new Node(obj, topOfStack); return obj; } /** * Remove and return the top item on the stack. * @pre The stack is not empty. * @post The top item on the stack has been removed and * the stack is one item smaller. * @return The top item on the stack * @throws NoSuchElementException, if the stack is empty */ @Override public E pop() { if (isEmpty()) { throw new NoSuchElementException(); } else { E result = topOfStack.data; topOfStack = topOfStack.next; return result; } } /** * Return the top item on the stack. * @pre The stack is not empty. * @post The stack remains unchanged. * @return The top item on the stack * @throws NoSuchElementException if the stack is empty */ @Override public E peek() { if (isEmpty()) { throw new NoSuchElementException(); } else { return topOfStack.data; } } /** * See whether the stack is empty. * @return true if the stack is empty */ @Override public boolean isEmpty() { return (topOfStack == null); } } // End of class LinkedStack
Java
Only Do Method, I will give LinkedList Implementation
Write a method checkForEquality to be included in class KWLinkedList that does not have any parameter. Assume the list is having even number of nodes. The method returns true if the first half of the list is equal to the second half of the list in the reverse order, otherwise it returns false. If the list is empty, the method returns false;
import java.util.NoSuchElementException;
/** Implementation of the interface StackInt using a linked list. The
* top element of the stack is in the first (front) node of the linked list.
*/
public class LinkedStack<E> implements StackInt<E>
{
/** Node: inner class to create nodes for linked list based stack. */
private static class Node<E>
{
// Data Fields
private E data;
private Node<E> next; // The reference to the next node.
// Constructors
/**
* Creates a new node with a null next field.
* @param dataItem The data to be stored in the node
*/
private Node(E dataItem)
{
data = dataItem;
next = null;
}
/**
* Creates a new node that references another node.
* @param dataItem The data to be stored in the node
* @param nodeRef The node referenced by new node
*/
private Node(E dataItem, Node<E> nodeRef)
{
data = dataItem;
next = nodeRef;
}
} //End of class Node
// Data Field: The reference to the first node of the stack.
private Node<E> topOfStack;
// Constructor
public LinkedStack()
{
topOfStack = null; // Initially the stack is empty
}
// copy constructor
public LinkedStack(LinkedStack<E> other)
{
if(other.isEmpty()) tofOfStack=null;
else
{
Node<E> newNode = new Node<E> (other.topOfStack.data);
topOfStack = newNode;
Node<E> ptr= other.topOdStack.next;
Node<E> ptr1 = topOdStack;
while (ptr != null)
{
newNode = new Node<E>(ptr.data);
ptr1.next=newNode;
ptr = ptr.next;
ptr1=ptr1.next;
}
}
}
/**
* Insert a new item on top of the stack.
* @post The new item is the top item on the stack.
* @param obj The item to be inserted
* @return The item that was inserted
*/
@Override
public E push(E obj)
{
topOfStack = new Node<E>(obj, topOfStack);
return obj;
}
/**
* Remove and return the top item on the stack.
* @pre The stack is not empty.
* @post The top item on the stack has been removed and
* the stack is one item smaller.
* @return The top item on the stack
* @throws NoSuchElementException, if the stack is empty
*/
@Override
public E pop()
{
if (isEmpty()) {
throw new NoSuchElementException();
}
else {
E result = topOfStack.data;
topOfStack = topOfStack.next;
return result;
}
}
/**
* Return the top item on the stack.
* @pre The stack is not empty.
* @post The stack remains unchanged.
* @return The top item on the stack
* @throws NoSuchElementException if the stack is empty
*/
@Override
public E peek()
{
if (isEmpty()) {
throw new NoSuchElementException();
}
else {
return topOfStack.data;
}
}
/**
* See whether the stack is empty.
* @return true if the stack is empty
*/
@Override
public boolean isEmpty()
{
return (topOfStack == null);
}
} // End of class LinkedStack

Step by step
Solved in 3 steps with 1 images

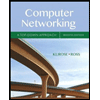
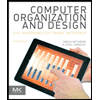
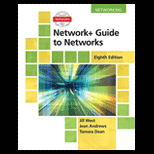
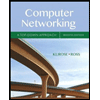
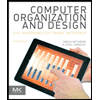
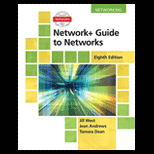
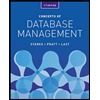
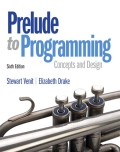
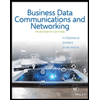