java code does not run and I don't know what is wrong with it. can someone help fix it. import javax.swing.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class RestaurantOrderSystem extends JFrame implements ActionListener { private JLabel title; private JPanel panel; private JComboBox foodList; private JCheckBox extraCheese; private JRadioButton smallSize, mediumSize, largeSize; private JTextField totalPrice; private JButton orderButton; private double basePrice = 0.0; private double total = 0.0; public RestaurantOrderSystem() { setTitle("Restaurant Order System"); setSize(400, 400); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // create title label title = new JLabel("Select your food and options:"); title.setBounds(20, 10, 300, 30); add(title); // create panel for components panel = new JPanel(); panel.setLayout(null); panel.setBounds(20, 50, 300, 140); add(panel); // create food list combo box String[] foodOptions = {"Pizza", "Burger", "Fries", "Salad"}; foodList = new JComboBox<>(foodOptions); foodList.setBounds(10, 10, 120, 30); panel.add(foodList); // create extra cheese check box extraCheese = new JCheckBox("Extra Cheese (+$1.50)"); extraCheese.setBounds(10, 50, 200, 30); panel.add(extraCheese); // create pizza size radio buttons smallSize = new JRadioButton("Small ($5.00)"); smallSize.setBounds(10, 90, 100, 30); smallSize.addActionListener(this); panel.add(smallSize); mediumSize = new JRadioButton("Medium ($7.50)"); mediumSize.setBounds(110, 90, 120, 30); mediumSize.addActionListener(this); panel.add(mediumSize); largeSize = new JRadioButton("Large ($10.00)"); largeSize.setBounds(230, 90, 100, 30); largeSize.addActionListener(this); panel.add(largeSize); // create total price text field totalPrice = new JTextField("$0.00"); totalPrice.setBounds(140, 10, 150, 30); totalPrice.setEditable(false); panel.add(totalPrice); // create order button orderButton = new JButton("Place Order"); orderButton.setBounds(110, 190, 120, 30); orderButton.addActionListener(this); add(orderButton); setVisible(true); } public void actionPerformed(ActionEvent e) { if (e.getSource() == smallSize) { basePrice = 5.0; updateTotalPrice(); } else if (e.getSource() == mediumSize) { basePrice = 7.5; updateTotalPrice(); } else if (e.getSource() == largeSize) { basePrice = 10.0; updateTotalPrice(); } else if (e.getSource() == orderButton) { JOptionPane.showMessageDialog(this, "Your order has been placed. Total cost: " + totalPrice.getText()); } } private void updateTotalPrice() { total = basePrice; if (extraCheese.isSelected()) { total += 1.5; } totalPrice.setText("$" + String.format("%.2f", total)); } public static void main(String[] args) { RestaurantOrderSystem ros = new RestaurantOrderSystem(); } }
java code does not run and I don't know what is wrong with it. can someone help fix it.
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class RestaurantOrderSystem extends JFrame implements ActionListener {
private JLabel title;
private JPanel panel;
private JComboBox<String> foodList;
private JCheckBox extraCheese;
private JRadioButton smallSize, mediumSize, largeSize;
private JTextField totalPrice;
private JButton orderButton;
private double basePrice = 0.0;
private double total = 0.0;
public RestaurantOrderSystem() {
setTitle("Restaurant Order System");
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create title label
title = new JLabel("Select your food and options:");
title.setBounds(20, 10, 300, 30);
add(title);
// create panel for components
panel = new JPanel();
panel.setLayout(null);
panel.setBounds(20, 50, 300, 140);
add(panel);
// create food list combo box
String[] foodOptions = {"Pizza", "Burger", "Fries", "Salad"};
foodList = new JComboBox<>(foodOptions);
foodList.setBounds(10, 10, 120, 30);
panel.add(foodList);
// create extra cheese check box
extraCheese = new JCheckBox("Extra Cheese (+$1.50)");
extraCheese.setBounds(10, 50, 200, 30);
panel.add(extraCheese);
// create pizza size radio buttons
smallSize = new JRadioButton("Small ($5.00)");
smallSize.setBounds(10, 90, 100, 30);
smallSize.addActionListener(this);
panel.add(smallSize);
mediumSize = new JRadioButton("Medium ($7.50)");
mediumSize.setBounds(110, 90, 120, 30);
mediumSize.addActionListener(this);
panel.add(mediumSize);
largeSize = new JRadioButton("Large ($10.00)");
largeSize.setBounds(230, 90, 100, 30);
largeSize.addActionListener(this);
panel.add(largeSize);
// create total price text field
totalPrice = new JTextField("$0.00");
totalPrice.setBounds(140, 10, 150, 30);
totalPrice.setEditable(false);
panel.add(totalPrice);
// create order button
orderButton = new JButton("Place Order");
orderButton.setBounds(110, 190, 120, 30);
orderButton.addActionListener(this);
add(orderButton);
setVisible(true);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == smallSize) {
basePrice = 5.0;
updateTotalPrice();
} else if (e.getSource() == mediumSize) {
basePrice = 7.5;
updateTotalPrice();
} else if (e.getSource() == largeSize) {
basePrice = 10.0;
updateTotalPrice();
} else if (e.getSource() == orderButton) {
JOptionPane.showMessageDialog(this, "Your order has been placed. Total cost: " + totalPrice.getText());
}
}
private void updateTotalPrice() {
total = basePrice;
if (extraCheese.isSelected()) {
total += 1.5;
}
totalPrice.setText("$" + String.format("%.2f", total));
}
public static void main(String[] args) {
RestaurantOrderSystem ros = new RestaurantOrderSystem();
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 6 images

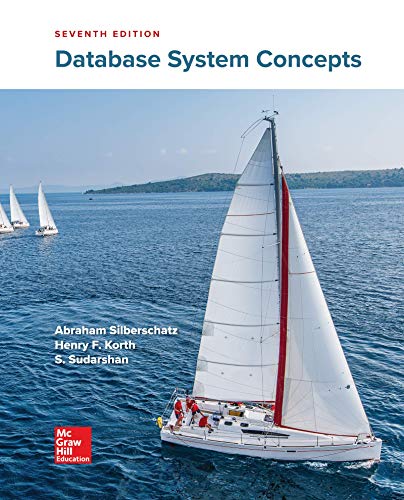
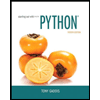
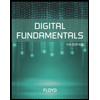
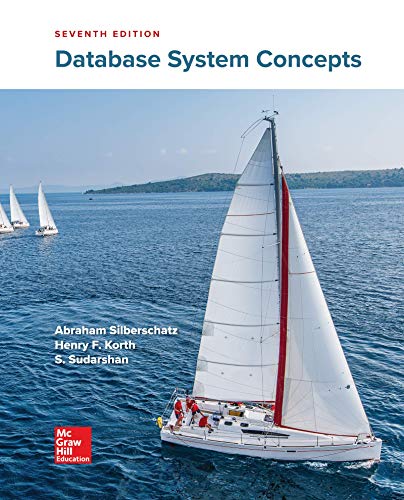
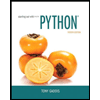
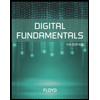
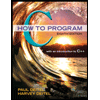
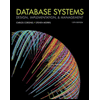
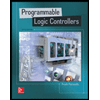