Irvine Ranch Water District (adapted from irwd.com) Write a C++ program to estimate your water bill for next month. Your water meter measures the volume of water you use in hundreds of cubic feet (CCF). One CCF of water equals approximately 748 gallons. INPUT All input statements and their user prompts need to be in main() since only pass by value is being used in this lab. Your program is to prompt the user for: Number of residents in household (integer > 0) Integer number between 1 to 12 inclusive for the month of the year. Number of days in billing cycle Type of residence (user to enter character as follows): S – Single-family Residence C – Condo A – Apartment Program should not be case-sensitive. That is if user enters a lowercase ‘s’ your program is to treat it as an uppercase ‘S’ either by using toupper() function or through ‘if’ statement. If user enters a negative number for the number of residents, output an error message and exit the program. If the month is entered out of range 1. . .12, output an error message and exit the program. If letter other than s, S, c, C, a, or A is entered, output an error message and exit the program. CALCULATE Calculate the amount of bill. This calculation is to be completed in four separate functions each called from main(). FIRST CALCULATION FUNCTION to be called from main().Calculate budget (water allocation) for people. Allow 50 gallons per person, per day (assume a 30 day month). So if 4 people: 8.02 =50*people*30/748 =50*4*30/748 but in code use number of days in billing cycle SECOND CALCULATION FUNCTION to be called from main(). Then calculate budget for outdoor landscape: Type of residence Month budget Single-family Residence 1, 2, 3, 4, 10, 11, 12 2.30 Single Family Residence 5, 6, 7, 8, 9 4.25 Condo 1, 2, 3, 4, 10, 11, 12 0.75 Condo 5, 6, 7, 8, 9 1.50 Apartment All months 0.00 THIRD CALCULATION FUNCTION to be called from main(). Budget Total = Total Allocation = budget for people + budget for landscape Return the budget total (alias total allocation for the customer). The word 'budget' in this problem is not money but CCFs of water. FOURTH CALCULATION FUNCTION Calculate Cost as $1.74 per CCF used under or equal to their water budget and on the amount of water over their water budget $6.25 per CCF . Add to this: Water service = number of days in billing cycle times $0.8966 per day Sewer service = number of days in billing cycle times $0.6818 per day This total is to be returned from the function. OUTPUT Separate output function called from main() performs all output. A sample run might look like this: Sample Run Enter number of people in household: 4 Enter type of residence: ‘S’ for single family, ‘C’ for condo, or ‘A’ for apartment: s Enter Month 3 Enter number of days in billing cycle: 28 Enter Actual CCF used: 12.0 Budget for people and landscape: 9.79 Total cost: $ 75.04 THEME ISSUES Functions (pass by value only), IF Control Structures, character type
Irvine Ranch Water District (adapted from irwd.com)
Write a C++ program to estimate your water bill for next month. Your water meter measures the volume of water you use in hundreds of cubic feet (CCF). One CCF of water equals approximately 748 gallons.
INPUT
All input statements and their user prompts need to be in main() since only pass by value is being used in this lab. Your program is to prompt the user for:
- Number of residents in household (integer > 0)
- Integer number between 1 to 12 inclusive for the month of the year.
- Number of days in billing cycle
- Type of residence (user to enter character as follows):
S – Single-family Residence
C – Condo
A – Apartment
Program should not be case-sensitive. That is if user enters a lowercase ‘s’ your program is to treat it as an uppercase ‘S’ either by using toupper() function or through ‘if’ statement.
If user enters a negative number for the number of residents, output an error message and exit the program. If the month is entered out of range 1. . .12, output an error message and exit the program. If letter other than s, S, c, C, a, or A is entered, output an error message and exit the program.
CALCULATE
Calculate the amount of bill. This calculation is to be completed in four separate functions each called from main().
FIRST CALCULATION FUNCTION to be called from main().Calculate budget (water allocation) for people. Allow 50 gallons per person, per day (assume a 30 day month).
So if 4 people: 8.02 =50*people*30/748 =50*4*30/748 but in code use number of days in billing cycle
SECOND CALCULATION FUNCTION to be called from main().
Then calculate budget for outdoor landscape:
Single-family Residence 1, 2, 3, 4, 10, 11, 12 2.30
Single Family Residence 5, 6, 7, 8, 9 4.25
Condo 1, 2, 3, 4, 10, 11, 12 0.75
Condo 5, 6, 7, 8, 9 1.50
Apartment All months 0.00
THIRD CALCULATION FUNCTION to be called from main().
Calculate Cost as $1.74 per CCF used under or equal to their water budget and on the amount of water over their water budget $6.25 per CCF . Add to this:
Sewer service = number of days in billing cycle times $0.6818 per day
Enter number of people in household: 4
Enter type of residence: ‘S’ for single family, ‘C’ for condo, or ‘A’ for apartment: s
Enter Month 3
Enter number of days in billing cycle: 28
Enter Actual CCF used: 12.0
Total cost: $ 75.04

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

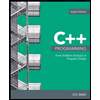
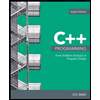