(Intro to Java) AVOID USING BREAKS. DO NOT USE BREAKS New Password In this assignment, you will write a short program to allow a user to create a new password, and then login with this password. You will need to use both if-else and for loops. The program will begin by prompting the user to enter a new password. The program will then ask the user to confirm the password. From there, one of several possible scenarios will occur. 1. The second password the user types does not match the first: In this case, allow the user one more try by asking them to confirm the password again Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc123 Password confirmed. Logging out... Should the user type the password incorrectly twice, the program should exit. Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc124 Sorry! Those passwords don't match. Goodbye! 2. The second password matches the first (either on the first or second attempt): In this case, log the user out, and then ask the user to login using their new password Enter your new password: abc123 Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: Give the user exactly 5 tries to type in their new password correctly (use a for loop) - reporting the number of tries remaining, for each attempt: Should the user type the password correctly within the 5 tries, log the user in Password confirmed. Logging out... Enter your password: abc124 Invalid password. You have 4 more tries. Enter your password: abc125 Invalid password. You have 3 more tries. Enter your password: abc123 Welcome! You are now logged in. Otherwise, should the user not type the password correctly within 5 tries, exit the program. Password confirmed. Logging out... Enter your password: ab Invalid password. You have 4 more tries. Enter your password: abc Invalid password. You have 3 more tries. Enter your password: ab3 Invalid password. You have 2 more tries. Enter your password: abc1 Invalid password. You have 1 more tries. Enter your password: abc12 Invalid password. You have 0 more tries. Goodbye! Starter Code: Copy and paste the below starter code into a file NewPasswords.java /** * @author * @author * CIS 36A */ import java.util.Scanner; public class NewPasswords { public static void main(String[] args) { Scanner input = new Scanner(System.in); String password; String passwordConfirm; final int MAX_TRIES = 5; System.out.print("Enter your new password: "); password = input.next(); //fill in here if (//fill in test condition here){ System.out.println("\nSorry! Those passwords don't match.\nPlease try again"); //fill in here } if (password.equals(passwordConfirm)) { System.out.println("\nPassword confirmed. Logging out...\n"); for (//fill in here... hint count DOWN) { System.out.print("Enter your password: "); //fill in here if (//fill in here)) { System.out.println("\nWelcome! You are now logged in."); i = 0; //What does this line do? Make sure you understand } else { System.out.print("Invalid password.); //fill in here } } } else { System.out.print("Sorry! Those passwords don't match."); } System.out.println("\nGoodbye!"); input.close(); } } Fill in the missing parts of the starter code where the comments indicate When your program works identically to the sample output below, submit it to Canvas. Sample Output: Enter your new password: abc123 Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: abc123 Welcome! You are now logged in. Goodbye! Sample Output: Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: abc124 Invalid password. You have 4 more tries. Enter your password: abc1265 Invalid password. You have 3 more tries. Enter your password: abc124 Invalid password. You have 2 more tries. Enter your password: abc12 Invalid password. You have 1 more tries. Enter your password: abc123 Welcome! You are now logged in. Goodbye!
(Intro to Java) AVOID USING BREAKS. DO NOT USE BREAKS New Password In this assignment, you will write a short program to allow a user to create a new password, and then login with this password. You will need to use both if-else and for loops. The program will begin by prompting the user to enter a new password. The program will then ask the user to confirm the password. From there, one of several possible scenarios will occur. 1. The second password the user types does not match the first: In this case, allow the user one more try by asking them to confirm the password again Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc123 Password confirmed. Logging out... Should the user type the password incorrectly twice, the program should exit. Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc124 Sorry! Those passwords don't match. Goodbye! 2. The second password matches the first (either on the first or second attempt): In this case, log the user out, and then ask the user to login using their new password Enter your new password: abc123 Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: Give the user exactly 5 tries to type in their new password correctly (use a for loop) - reporting the number of tries remaining, for each attempt: Should the user type the password correctly within the 5 tries, log the user in Password confirmed. Logging out... Enter your password: abc124 Invalid password. You have 4 more tries. Enter your password: abc125 Invalid password. You have 3 more tries. Enter your password: abc123 Welcome! You are now logged in. Otherwise, should the user not type the password correctly within 5 tries, exit the program. Password confirmed. Logging out... Enter your password: ab Invalid password. You have 4 more tries. Enter your password: abc Invalid password. You have 3 more tries. Enter your password: ab3 Invalid password. You have 2 more tries. Enter your password: abc1 Invalid password. You have 1 more tries. Enter your password: abc12 Invalid password. You have 0 more tries. Goodbye! Starter Code: Copy and paste the below starter code into a file NewPasswords.java /** * @author * @author * CIS 36A */ import java.util.Scanner; public class NewPasswords { public static void main(String[] args) { Scanner input = new Scanner(System.in); String password; String passwordConfirm; final int MAX_TRIES = 5; System.out.print("Enter your new password: "); password = input.next(); //fill in here if (//fill in test condition here){ System.out.println("\nSorry! Those passwords don't match.\nPlease try again"); //fill in here } if (password.equals(passwordConfirm)) { System.out.println("\nPassword confirmed. Logging out...\n"); for (//fill in here... hint count DOWN) { System.out.print("Enter your password: "); //fill in here if (//fill in here)) { System.out.println("\nWelcome! You are now logged in."); i = 0; //What does this line do? Make sure you understand } else { System.out.print("Invalid password.); //fill in here } } } else { System.out.print("Sorry! Those passwords don't match."); } System.out.println("\nGoodbye!"); input.close(); } } Fill in the missing parts of the starter code where the comments indicate When your program works identically to the sample output below, submit it to Canvas. Sample Output: Enter your new password: abc123 Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: abc123 Welcome! You are now logged in. Goodbye! Sample Output: Enter your new password: abc123 Enter your new password again: abc124 Sorry! Those passwords don't match. Please try again Enter your new password again: abc123 Password confirmed. Logging out... Enter your password: abc124 Invalid password. You have 4 more tries. Enter your password: abc1265 Invalid password. You have 3 more tries. Enter your password: abc124 Invalid password. You have 2 more tries. Enter your password: abc12 Invalid password. You have 1 more tries. Enter your password: abc123 Welcome! You are now logged in. Goodbye!
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
(Intro to Java)
AVOID USING BREAKS. DO NOT USE BREAKS
New Password
- In this assignment, you will write a short program to allow a user to create a new password, and then login with this password.
- You will need to use both if-else and for loops.
- The program will begin by prompting the user to enter a new password. The program will then ask the user to confirm the password.
- From there, one of several possible scenarios will occur.
1. The second password the user types does not match the first:
- In this case, allow the user one more try by asking them to confirm the password again
Enter your new password: abc123
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc123
Password confirmed. Logging out...
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc123
Password confirmed. Logging out...
- Should the user type the password incorrectly twice, the program should exit.
Enter your new password: abc123
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc124
Sorry! Those passwords don't match.
Goodbye!
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc124
Sorry! Those passwords don't match.
Goodbye!
2. The second password matches the first (either on the first or second attempt):
- In this case, log the user out, and then ask the user to login using their new password
Enter your new password: abc123
Enter your new password again: abc123
Password confirmed. Logging out...
Enter your password:
Enter your new password again: abc123
Password confirmed. Logging out...
Enter your password:
- Give the user exactly 5 tries to type in their new password correctly (use a for loop) - reporting the number of tries remaining, for each attempt:
- Should the user type the password correctly within the 5 tries, log the user in
Password confirmed. Logging out...
Enter your password: abc124
Invalid password. You have 4 more tries.
Enter your password: abc125
Invalid password. You have 3 more tries.
Enter your password: abc123
Welcome! You are now logged in.
Enter your password: abc124
Invalid password. You have 4 more tries.
Enter your password: abc125
Invalid password. You have 3 more tries.
Enter your password: abc123
Welcome! You are now logged in.
- Otherwise, should the user not type the password correctly within 5 tries, exit the program.
Password confirmed. Logging out...
Enter your password: ab
Invalid password. You have 4 more tries.
Enter your password: abc
Invalid password. You have 3 more tries.
Enter your password: ab3
Invalid password. You have 2 more tries.
Enter your password: abc1
Invalid password. You have 1 more tries.
Enter your password: abc12
Invalid password. You have 0 more tries.
Goodbye!
Enter your password: ab
Invalid password. You have 4 more tries.
Enter your password: abc
Invalid password. You have 3 more tries.
Enter your password: ab3
Invalid password. You have 2 more tries.
Enter your password: abc1
Invalid password. You have 1 more tries.
Enter your password: abc12
Invalid password. You have 0 more tries.
Goodbye!
Starter Code: Copy and paste the below starter code into a file NewPasswords.java
/**
* @author
* @author
* CIS 36A
*/
import java.util.Scanner;
public class NewPasswords {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String password;
String passwordConfirm;
final int MAX_TRIES = 5;
System.out.print("Enter your new password: ");
password = input.next();
* @author
* @author
* CIS 36A
*/
import java.util.Scanner;
public class NewPasswords {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
String password;
String passwordConfirm;
final int MAX_TRIES = 5;
System.out.print("Enter your new password: ");
password = input.next();
//fill in here
if (//fill in test condition here){
System.out.println("\nSorry! Those passwords don't match.\nPlease try again");
//fill in here
}
if (password.equals(passwordConfirm)) {
System.out.println("\nPassword confirmed. Logging out...\n");
for (//fill in here... hint count DOWN) {
System.out.print("Enter your password: ");
if (password.equals(passwordConfirm)) {
System.out.println("\nPassword confirmed. Logging out...\n");
for (//fill in here... hint count DOWN) {
System.out.print("Enter your password: ");
//fill in here
if (//fill in here)) {
System.out.println("\nWelcome! You are now logged in.");
i = 0; //What does this line do? Make sure you understand
} else {
System.out.print("Invalid password.);
System.out.println("\nWelcome! You are now logged in.");
i = 0; //What does this line do? Make sure you understand
} else {
System.out.print("Invalid password.);
//fill in here
}
}
} else {
System.out.print("Sorry! Those passwords don't match.");
}
System.out.println("\nGoodbye!");
input.close();
}
}
}
} else {
System.out.print("Sorry! Those passwords don't match.");
}
System.out.println("\nGoodbye!");
input.close();
}
}
- Fill in the missing parts of the starter code where the comments indicate
- When your program works identically to the sample output below, submit it to Canvas.
Sample Output:
Enter your new password: abc123Enter your new password again: abc123
Password confirmed. Logging out...
Enter your password: abc123
Welcome! You are now logged in.
Goodbye!
Sample Output:
Enter your new password: abc123
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc123
Password confirmed. Logging out...
Enter your password: abc124
Invalid password. You have 4 more tries.
Enter your password: abc1265
Invalid password. You have 3 more tries.
Enter your password: abc124
Invalid password. You have 2 more tries.
Enter your password: abc12
Invalid password. You have 1 more tries.
Enter your password: abc123
Welcome! You are now logged in.
Goodbye!
Enter your new password again: abc124
Sorry! Those passwords don't match.
Please try again
Enter your new password again: abc123
Password confirmed. Logging out...
Enter your password: abc124
Invalid password. You have 4 more tries.
Enter your password: abc1265
Invalid password. You have 3 more tries.
Enter your password: abc124
Invalid password. You have 2 more tries.
Enter your password: abc12
Invalid password. You have 1 more tries.
Enter your password: abc123
Welcome! You are now logged in.
Goodbye!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
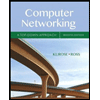
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
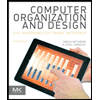
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
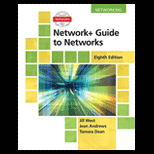
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
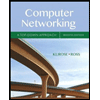
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
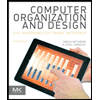
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
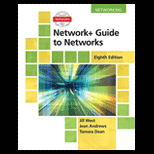
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
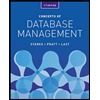
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
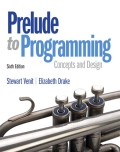
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
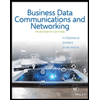
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY