In the ExampleArrays class, you will use the design recipe to write a method called sumPairs that will take 2 Pair[] as arguments and return a Pair[]. Each element of the returned Pair[] will be the result of adding both Pair objects at the same index in the 2 Pair[] given as arguments. If the 2 Pair[] are of different lengths, then you will sum the pairs up to the final pair of the shortest Pair[]. You may safely ignore the remaining Pair objects of the longer Pair[]. If any Pair[] is empty, then you will return an empty (length of o) Pair[]. In the file ExampleArrays.java, you will add a new class OUTSIDE the ExampleArrays class called Pair. It will have two fields of type int called a and b. It's constructor will initialize both fields to be the value as specified by the arguments given to the constructor. Adding 2 Pair objects means to sum their a fields together and to sum their b fields together
In the ExampleArrays class, you will use the design recipe to write a method called sumPairs that will take 2 Pair[] as arguments and return a Pair[]. Each element of the returned Pair[] will be the result of adding both Pair objects at the same index in the 2 Pair[] given as arguments. If the 2 Pair[] are of different lengths, then you will sum the pairs up to the final pair of the shortest Pair[]. You may safely ignore the remaining Pair objects of the longer Pair[]. If any Pair[] is empty, then you will return an empty (length of o) Pair[]. In the file ExampleArrays.java, you will add a new class OUTSIDE the ExampleArrays class called Pair. It will have two fields of type int called a and b. It's constructor will initialize both fields to be the value as specified by the arguments given to the constructor. Adding 2 Pair objects means to sum their a fields together and to sum their b fields together
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
java intro. Could only use simple syntax,like for loop,if,constructor.
![(and to help make sure we agree on how these methods work).
These tests have been provided in Sanity.java for your
convenience.
class ProvidedArrayTests {
ExampleArrays ea = new ExampleArrays ( );
Сорy
Look Up
Translate
Share...
B)};
void testSumPairs (Tester t) {
Pair[] p1 = {new Pair(1,2), new Pair(3,4), new Pair(5,6)};
Pair[] p2 = {new Pair(1,2), new Pair(3,6)};
Pair[] expect_p1_p2 = {new Pair(2, 4), new Pair(6, 10) };
Pair[] result_p1_p2 = ea.sumPairs(p1, p2);
t.checkExpect(result_p1_p2, expect_p1_p2);
}
void testSumPairsEmpty(Tester t) {
Pair[] p1
Pair[] p2 = new Pair[0];
= {new Pair(1,2), new Pair(3,4), new Pair(5,6)};
Pair[] expect_p1_p2 = new Pair [0];
Pair[] result_p1_p2 = ea.sumPairs(p1, p2);
t.checkExpect(result_p1_p2, expect_p1_p2);
void testOnEdge (Tester t) {
Region [] r1 = {new RectRegion(new Point(0,1), new Point(6,6)), new CircleRegion(new Po](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F67a79aa2-f715-406c-8bee-8178252bb86d%2Fc653cd73-9c79-4479-b6b6-cebcddf4b048%2Fvhs2clp_processed.jpeg&w=3840&q=75)
Transcribed Image Text:(and to help make sure we agree on how these methods work).
These tests have been provided in Sanity.java for your
convenience.
class ProvidedArrayTests {
ExampleArrays ea = new ExampleArrays ( );
Сорy
Look Up
Translate
Share...
B)};
void testSumPairs (Tester t) {
Pair[] p1 = {new Pair(1,2), new Pair(3,4), new Pair(5,6)};
Pair[] p2 = {new Pair(1,2), new Pair(3,6)};
Pair[] expect_p1_p2 = {new Pair(2, 4), new Pair(6, 10) };
Pair[] result_p1_p2 = ea.sumPairs(p1, p2);
t.checkExpect(result_p1_p2, expect_p1_p2);
}
void testSumPairsEmpty(Tester t) {
Pair[] p1
Pair[] p2 = new Pair[0];
= {new Pair(1,2), new Pair(3,4), new Pair(5,6)};
Pair[] expect_p1_p2 = new Pair [0];
Pair[] result_p1_p2 = ea.sumPairs(p1, p2);
t.checkExpect(result_p1_p2, expect_p1_p2);
void testOnEdge (Tester t) {
Region [] r1 = {new RectRegion(new Point(0,1), new Point(6,6)), new CircleRegion(new Po
![Number. That method will be called compare and it will take a
Number as an argument and will return an integer.
Your compare method will behave as follows:
• Case 1: If this is numerically greater than other, then
return 1
• Case 2: If this is numerically lesser than other, then return
Соpy
Look Up
Translate
Share...
Task 1.2
In the ExampleArrays class, you will use the design recipe to
write a method called sumPairs that will take 2 Pair[] as
arguments and return a Pair[]. Each element of the returned
Pair[] will be the result of adding both Pair objects at the
same index in the 2 Pair[] given as arguments. If the 2 Pair[]
are of different lengths, then you will sum the pairs up to the final
pair of the shortest Pair[]. You may safely ignore the remaining
Pair objects of the longer Pair[]. If any Pair[] is empty,
you will return an empty (length of o) Pair [].
then
In the file ExampleArrays.java, you will add a new class
OUTSIDE the ExampleArrays class called Pair. It will have
two fields of type int called a and b. It's constructor will initialize
both fields to be the value as specified by the arguments given to
the constructor.
Adding 2 Pair objects means to sum their a fields together and
to sum their b fields together
Task 1.3
In the ExampleArrays class, you will use the design recipe to
write a method called onRegionEdge that will take a Region []
and Point as arguments and return a Region []. The resulting
Region [] will consist of all regions where the Point given as
argument lies on the edge of the region. If the Region[] given
as argument is empty, return an empty Region [].](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F67a79aa2-f715-406c-8bee-8178252bb86d%2Fc653cd73-9c79-4479-b6b6-cebcddf4b048%2Ffgyr82h_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Number. That method will be called compare and it will take a
Number as an argument and will return an integer.
Your compare method will behave as follows:
• Case 1: If this is numerically greater than other, then
return 1
• Case 2: If this is numerically lesser than other, then return
Соpy
Look Up
Translate
Share...
Task 1.2
In the ExampleArrays class, you will use the design recipe to
write a method called sumPairs that will take 2 Pair[] as
arguments and return a Pair[]. Each element of the returned
Pair[] will be the result of adding both Pair objects at the
same index in the 2 Pair[] given as arguments. If the 2 Pair[]
are of different lengths, then you will sum the pairs up to the final
pair of the shortest Pair[]. You may safely ignore the remaining
Pair objects of the longer Pair[]. If any Pair[] is empty,
you will return an empty (length of o) Pair [].
then
In the file ExampleArrays.java, you will add a new class
OUTSIDE the ExampleArrays class called Pair. It will have
two fields of type int called a and b. It's constructor will initialize
both fields to be the value as specified by the arguments given to
the constructor.
Adding 2 Pair objects means to sum their a fields together and
to sum their b fields together
Task 1.3
In the ExampleArrays class, you will use the design recipe to
write a method called onRegionEdge that will take a Region []
and Point as arguments and return a Region []. The resulting
Region [] will consist of all regions where the Point given as
argument lies on the edge of the region. If the Region[] given
as argument is empty, return an empty Region [].
Expert Solution

Step 1
public class Pair {
int a, b;
Pair(int a, int b) {
this.a = a;
this.b = b;
}
}
class ExampleArrays {
public Pair[] sumPairs(Pair[] p1, Pair[] p2) {
Pair[] result;
if (p1.length == 0 || p2.length == 0)
result = new Pair[0];
else if (p1.length <= p2.length) {
result = new Pair[p1.length];
for (int i = 0; i < p1.length; i++) {
result[i] = new Pair(p1[i].a + p2[i].a, p1[i].b + p2[i].b);
}
} else {
result = new Pair[p2.length];
for (int i = 0; i < p2.length; i++) {
result[i] = new Pair(p1[i].a + p2[i].a, p1[i].b + p2[i].b);
}
}
return result;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
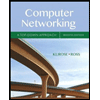
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
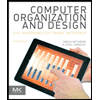
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
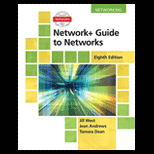
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
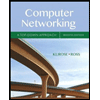
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
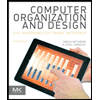
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
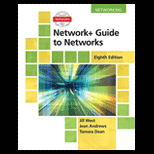
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
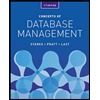
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
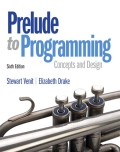
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
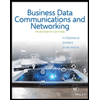
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY