In an old house in Russia several chests of full of hollow dolls have been discovered. These dolls appear to be from sets of matryoshka dolls. A set of matryoshka dolls is a series of dolls where doll1 fits inside doll2, doll2 fits inside doll3, and so on. Your task is to determine the sizes of the largest sets of matryoshka dolls that can be formed from each chest. Inside the chests the dolls have been all jumbled up. Fortunately. each doll has a unique name and your assistant has gone throught the dolls in each chest and determined which dolls fit inside which others. This has not been easy, as one doll may be shorter than another, but also may have larger feet. For this problem, you will be given information about each chest as a dictionary. The keys to this dictionary are doll names, and the value for each key is a list of other dolls in the chest that fit inside it. For a small doll, where no other dolls fit inside it, there will be no dictionary entry with the small dolls name as the key. If there is a dictionary entry with a certain dolls name then there is at least one doll that fits inside it (ie there are no empty list as values in the dictionary) The following is a sample of what this dictionary might look like: chesti = { "Dafna" : ["Vera", "Lada", "Alla","Zoe"], "Yana" : ["Zoe"], "Vera" : ["Inga"], "Alla" : ["Zoe"] In the example above, the doll Dafna can contain Zoe, but not Yana. Your first task is to write a recursive function that will determines the maximum number in a set of matyoshka dolls whose outermost doll's name is given. Your function should have 2 parameters: the chest which is the python dictionary containing the information about doll containment in the indicated chest, anda doll’s name. Your function should return the maximum number of dolls in a set of matyoshka dolls, that can be formed from dolls in the given chest, whose outermost doll has the given name. If the given outermost doll contains no others in the chest then one should be returned. Your second task to write a function that prints the maximum number in a set of matyoshka dolls, that includes at least two dolls, for each outermost doll in the given chest. This function has a single param- eter: the chest which is the python dictionary containing the information about doll containment in the indicated chest. This second function will not be recursive but will call your first recursive function. Finally, in the main program you will call your second function for each of the two provided chest dictio- naries. Provided File We are providing you with 2 chest dictionaries to use; you can find these on Moodle in asn8q2-starter.py. You can just copy/paste the dictionary literals into your code, or rename the file to a8q2.py and put your own code within. Sample Run If you call your second function with the provided chest1, you should be able to get output that looks like this:
In an old house in Russia several chests of full of hollow dolls have been discovered. These dolls appear to be from sets of matryoshka dolls. A set of matryoshka dolls is a series of dolls where doll1 fits inside doll2, doll2 fits inside doll3, and so on. Your task is to determine the sizes of the largest sets of matryoshka dolls that can be formed from each chest. Inside the chests the dolls have been all jumbled up. Fortunately. each doll has a unique name and your assistant has gone throught the dolls in each chest and determined which dolls fit inside which others. This has not been easy, as one doll may be shorter than another, but also may have larger feet. For this problem, you will be given information about each chest as a dictionary. The keys to this dictionary are doll names, and the value for each key is a list of other dolls in the chest that fit inside it. For a small doll, where no other dolls fit inside it, there will be no dictionary entry with the small dolls name as the key. If there is a dictionary entry with a certain dolls name then there is at least one doll that fits inside it (ie there are no empty list as values in the dictionary) The following is a sample of what this dictionary might look like: chesti = { "Dafna" : ["Vera", "Lada", "Alla","Zoe"], "Yana" : ["Zoe"], "Vera" : ["Inga"], "Alla" : ["Zoe"] In the example above, the doll Dafna can contain Zoe, but not Yana. Your first task is to write a recursive function that will determines the maximum number in a set of matyoshka dolls whose outermost doll's name is given. Your function should have 2 parameters: the chest which is the python dictionary containing the information about doll containment in the indicated chest, anda doll’s name. Your function should return the maximum number of dolls in a set of matyoshka dolls, that can be formed from dolls in the given chest, whose outermost doll has the given name. If the given outermost doll contains no others in the chest then one should be returned. Your second task to write a function that prints the maximum number in a set of matyoshka dolls, that includes at least two dolls, for each outermost doll in the given chest. This function has a single param- eter: the chest which is the python dictionary containing the information about doll containment in the indicated chest. This second function will not be recursive but will call your first recursive function. Finally, in the main program you will call your second function for each of the two provided chest dictio- naries. Provided File We are providing you with 2 chest dictionaries to use; you can find these on Moodle in asn8q2-starter.py. You can just copy/paste the dictionary literals into your code, or rename the file to a8q2.py and put your own code within. Sample Run If you call your second function with the provided chest1, you should be able to get output that looks like this:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python
![In an old house in Russia several chests of full of hollow dolls have been discovered. These dolls appear to
be from sets of matryoshka dolls. A set of matryoshka dolls is a series of dolls where doll1 fits inside doll2,
doll2 fits inside doll3, and so on. Your task is to determine the sizes of the largest sets of matryoshka dolls
that can be formed from each chest. Inside the chests the dolls have been all jumbled up. Fortunately.
each doll has a unique name and your assistant has gone throught the dolls in each chest and determined
which dolls fit inside which others. This has not been easy, as one doll may be shorter than another, but
also may have larger feet.
For this problem, you will be given information about each chest as a dictionary. The keys to this dictionary
are doll names, and the value for each key is a list of other dolls in the chest that fit inside it. For a small
doll, where no other dolls fit inside it, there will be no dictionary entry with the small dolls name as the
key. If there is a dictionary entry with a certain dolls name then there is at least one doll that fits inside it
(ie there are no empty list as values in the dictionary)
The following is a sample of what this dictionary might look like:
chesti = {
"Dafna" : ["Vera", "Lada", "Alla","Zoe"],
: ["Zoe"],
"Vera" : ["Inga"],
"Yana"
"Alla" : ["Zoe"]
}
In the example above, the doll Dafna can contain Zoe, but not Yana.
Your first task is to write a recursive function that will determines the maximum number in a set of matyoshka
dolls whose outermost doll's name is given. Your function should have 2 parameters: the chest which is the
python dictionary containing the information about doll containment in the indicated chest, anda doll's
name. Your function should return the maximum number of dolls in a set of matyoshka dolls, that can be
formed from dolls in the given chest, whose outermost doll has the given name. If the given outermost
doll contains no others in the chest then one should be returned.
Your second task to write a function that prints the maximum number in a set of matyoshka dolls, that
includes at least two dolls, for each outermost doll in the given chest. This function has a single param-
eter: the chest which is the python dictionary containing the information about doll containment in the
indicated chest. This second function will not be recursive but will call your first recursive function.
Finally, in the main program you will call your second function for each of the two provided chest dictio-
naries.
Provided File
We are providing you with 2 chest dictionaries to use; you can find these on Moodle in asn8q2-starter.py.
You can just copy/paste the dictionary literals into your code, or rename the file to a8q2.py and put your
own code within.
Sample Run
If you call your second function with the provided chest1, you should be able to get output that looks like
this:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6dd555f4-ee7d-4b78-ae77-9a2f3a6aea24%2F5d327114-ec9d-419e-a374-0b3d2398e2e3%2F4ey02eq_processed.png&w=3840&q=75)
Transcribed Image Text:In an old house in Russia several chests of full of hollow dolls have been discovered. These dolls appear to
be from sets of matryoshka dolls. A set of matryoshka dolls is a series of dolls where doll1 fits inside doll2,
doll2 fits inside doll3, and so on. Your task is to determine the sizes of the largest sets of matryoshka dolls
that can be formed from each chest. Inside the chests the dolls have been all jumbled up. Fortunately.
each doll has a unique name and your assistant has gone throught the dolls in each chest and determined
which dolls fit inside which others. This has not been easy, as one doll may be shorter than another, but
also may have larger feet.
For this problem, you will be given information about each chest as a dictionary. The keys to this dictionary
are doll names, and the value for each key is a list of other dolls in the chest that fit inside it. For a small
doll, where no other dolls fit inside it, there will be no dictionary entry with the small dolls name as the
key. If there is a dictionary entry with a certain dolls name then there is at least one doll that fits inside it
(ie there are no empty list as values in the dictionary)
The following is a sample of what this dictionary might look like:
chesti = {
"Dafna" : ["Vera", "Lada", "Alla","Zoe"],
: ["Zoe"],
"Vera" : ["Inga"],
"Yana"
"Alla" : ["Zoe"]
}
In the example above, the doll Dafna can contain Zoe, but not Yana.
Your first task is to write a recursive function that will determines the maximum number in a set of matyoshka
dolls whose outermost doll's name is given. Your function should have 2 parameters: the chest which is the
python dictionary containing the information about doll containment in the indicated chest, anda doll's
name. Your function should return the maximum number of dolls in a set of matyoshka dolls, that can be
formed from dolls in the given chest, whose outermost doll has the given name. If the given outermost
doll contains no others in the chest then one should be returned.
Your second task to write a function that prints the maximum number in a set of matyoshka dolls, that
includes at least two dolls, for each outermost doll in the given chest. This function has a single param-
eter: the chest which is the python dictionary containing the information about doll containment in the
indicated chest. This second function will not be recursive but will call your first recursive function.
Finally, in the main program you will call your second function for each of the two provided chest dictio-
naries.
Provided File
We are providing you with 2 chest dictionaries to use; you can find these on Moodle in asn8q2-starter.py.
You can just copy/paste the dictionary literals into your code, or rename the file to a8q2.py and put your
own code within.
Sample Run
If you call your second function with the provided chest1, you should be able to get output that looks like
this:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
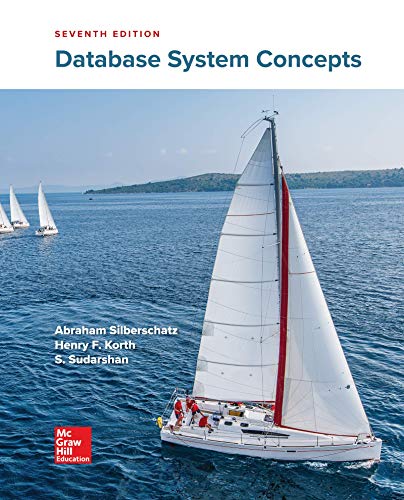
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
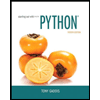
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
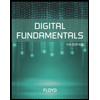
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
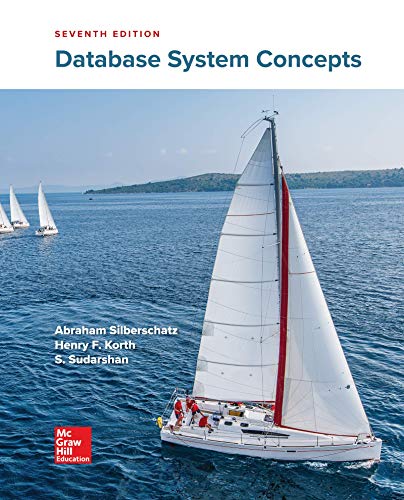
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
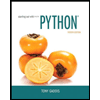
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
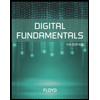
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
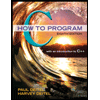
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
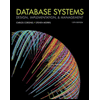
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
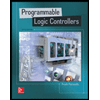
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education