I'm creating a Django project and I am having troubles using a model that I created for one of my classes *allgroups\models.py* class Allgroups(models.Model): title = models.CharField(max_length=255) body = models.TextField() date = models.DateTimeField(auto_now_add=True) private = models.BooleanField(default=False) discussion = models.CharField(max_length=255) author = models.ForeignKey( get_user_model(), on_delete=models.CASCADE, ) def __str__(self): return self.title def get_absolute_url(self): return reverse('allgroups_detail', args=[str(self.id)]) class userList(models.Model): allgroups = models.ForeignKey( Allgroups, on_delete=models.CASCADE, related_name = 'userList', ) user = models.ForeignKey( get_user_model(), on_delete=models.CASCADE, ) def __str__(self): return str(self.user) def get_absolute_url(self): return reverse('allgroups_list') ------------------------------------------------------------------------- *allgroups\views.py* from .models import Allgroups, userList class AllgroupsListView(ListView): model = Allgroups template_name = 'allgroups_list.html' class AllgroupsDetailView(DetailView): model = Allgroups template_name = 'allgroups_detail.html' class userListUpdateView(LoginRequiredMixin, UserPassesTestMixin, UpdateView): model = userList fields = ('user',) template_name = 'userlist_update.html' login_url = 'login' def test_func(self): obj = self.get_object() return obj.author == self.request.user ----------------------------------------------------------------------------------- *allgroups\urls.py* from django.urls import path, include from .views import ( AllgroupsListView, AllgroupsDetailView, userListUpdateView, ) urlpatterns = [ path('', AllgroupsListView.as_view(), name='allgroups_list'), path('/', AllgroupsDetailView.as_view(), name='allgroups_detail'), path('/update/', userListUpdateView.as_view(), name='userlist_update'), ] ---------------------------------------------------------------------------------------------------------------- *templates\allgroups_list.html* {% extends 'base.html' %} {% block title %}All Groups{% endblock title %} {% block content %} . . . {{ allgroups.body }} Updatet | . . . ----------------------------------------------------------------------- *templates\userlist_update* {% extends 'base.html' %} {% block content %} Working? {% csrf_token %} {{ form.as_p }} Update {% endblock content %} ------------------------------------------------------------------------------------------------ I'm not sure why I'm unable to use the userList class as a model for my view. When I change the userListUpdateView model = allgroups and fields = ('author'), it works perfectly. I'm able to update the userList in admin. Any help would be greatly appreciated! Thanks!
I'm creating a Django project and I am having troubles using a model that I created for one of my classes
*allgroups\models.py*
class Allgroups(models.Model):
title = models.CharField(max_length=255)
body = models.TextField()
date = models.DateTimeField(auto_now_add=True)
private = models.BooleanField(default=False)
discussion = models.CharField(max_length=255)
author = models.ForeignKey(
get_user_model(),
on_delete=models.CASCADE,
)
def __str__(self):
return self.title
def get_absolute_url(self):
return reverse('allgroups_detail', args=[str(self.id)])
class userList(models.Model):
allgroups = models.ForeignKey(
Allgroups,
on_delete=models.CASCADE,
related_name = 'userList',
)
user = models.ForeignKey(
get_user_model(),
on_delete=models.CASCADE,
)
def __str__(self):
return str(self.user)
def get_absolute_url(self):
return reverse('allgroups_list')
-------------------------------------------------------------------------
*allgroups\views.py*
from .models import Allgroups, userList
class AllgroupsListView(ListView):
model = Allgroups
template_name = 'allgroups_list.html'
class AllgroupsDetailView(DetailView):
model = Allgroups
template_name = 'allgroups_detail.html'
class userListUpdateView(LoginRequiredMixin, UserPassesTestMixin, UpdateView):
model = userList
fields = ('user',)
template_name = 'userlist_update.html'
login_url = 'login'
def test_func(self):
obj = self.get_object()
return obj.author == self.request.user
-----------------------------------------------------------------------------------
*allgroups\urls.py*
from django.urls import path, include
from .views import (
AllgroupsListView,
AllgroupsDetailView,
userListUpdateView,
)
urlpatterns = [
path('', AllgroupsListView.as_view(), name='allgroups_list'),
path('<int:pk>/', AllgroupsDetailView.as_view(), name='allgroups_detail'),
path('<int:pk>/update/', userListUpdateView.as_view(), name='userlist_update'),
]
----------------------------------------------------------------------------------------------------------------
*templates\allgroups_list.html*
{% extends 'base.html' %}
{% block title %}All Groups{% endblock title %}
{% block content %}
.
.
.
<div class="card=body">
<p>{{ allgroups.body }}</p>
<a href="{% url 'userlist_update' allgroups.pk %}">Updatet</a> |
</div>
.
.
.
-----------------------------------------------------------------------
*templates\userlist_update*
{% extends 'base.html' %}
{% block content %}
<h1>Working?</h1>
<form action="" method="post">{% csrf_token %}
{{ form.as_p }}
<button class="btn btn-info ml-2" type="submit">Update</button>
</form>
{% endblock content %}
------------------------------------------------------------------------------------------------
I'm not sure why I'm unable to use the userList class as a model for my view. When I change the userListUpdateView model = allgroups and fields = ('author'), it works perfectly. I'm able to update the userList in admin.
Any help would be greatly appreciated!
Thanks!

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

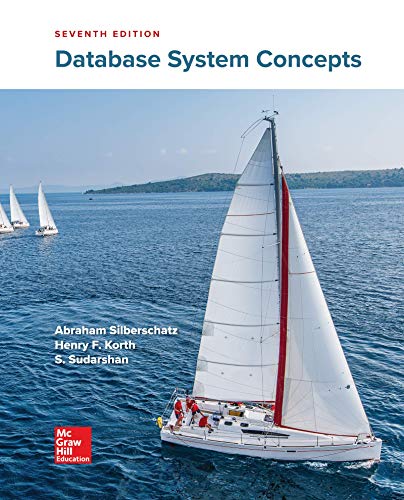
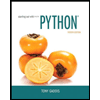
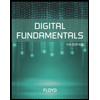
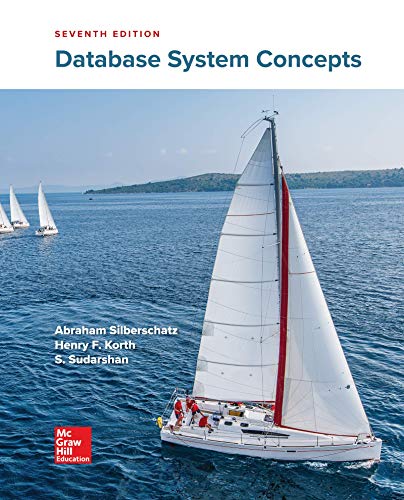
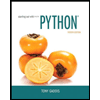
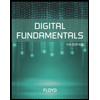
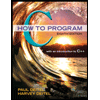
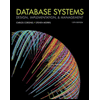
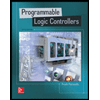