I am writing a C# program that uses LINQ. This is what it is supposed to do: Take a sequence of integers Remove all odd integers, leaving only even ones Squares each integer Removes any resulting integer that is greater than 50 But I am getting a System.Linq.Enumerable+WhereEnumerableIterator`1[System.Int32] error. What is causing it and how can I fix it?
I am writing a C# program that uses LINQ. This is what it is supposed to do: Take a sequence of integers Remove all odd integers, leaving only even ones Squares each integer Removes any resulting integer that is greater than 50 But I am getting a System.Linq.Enumerable+WhereEnumerableIterator`1[System.Int32] error. What is causing it and how can I fix it?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I am writing a C#
Take a sequence of integers
Remove all odd integers, leaving only even ones
Squares each integer
Removes any resulting integer that is greater than 50
But I am getting a System.Linq.Enumerable+WhereEnumerableIterator`1[System.Int32] error. What is causing it and how can I fix it?
![var numbers = Enumerable.Range(1,
var evenNumbers = numbers.where(n
Console.WriteLine(evenNumbers);
10);
=> n % 2 == 0);
// combine projection and filtering
var oddSquares = numbers.Select(x => x * x).where(y => y % 2 != 0);
Console.WriteLine(oddSquares);
// filter by type
object[] values = { 1, 2.5, 3, 4.56 };
var wholeNumbers = values.OfType<int>(); // try float or double
Console.WriteLine(wholeNumbers);
var rand = new Random();
var randomValues = Enumerable.Range(1, 10).Select(_ => rand.Next(10) - 5);
var csvString = new Func<IEnumerable<int>, string>(values =>
{
return string.Join(",", values.Select(v => v.ToString()).ToArray());|
});
// different set of values each time
Console.WriteLine(csvString(randomValues));
Console.WriteLine(csvString(randomValues.OrderBy(x => x)));
Console.WriteLine(csvString(randomValues.OrderByDescending(x => x)));](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc1602b80-c5b7-44a0-838e-9b62644e132e%2F080ec6ec-a571-4fc0-8193-2442b61021f7%2Fdzx9u3u_processed.png&w=3840&q=75)
Transcribed Image Text:var numbers = Enumerable.Range(1,
var evenNumbers = numbers.where(n
Console.WriteLine(evenNumbers);
10);
=> n % 2 == 0);
// combine projection and filtering
var oddSquares = numbers.Select(x => x * x).where(y => y % 2 != 0);
Console.WriteLine(oddSquares);
// filter by type
object[] values = { 1, 2.5, 3, 4.56 };
var wholeNumbers = values.OfType<int>(); // try float or double
Console.WriteLine(wholeNumbers);
var rand = new Random();
var randomValues = Enumerable.Range(1, 10).Select(_ => rand.Next(10) - 5);
var csvString = new Func<IEnumerable<int>, string>(values =>
{
return string.Join(",", values.Select(v => v.ToString()).ToArray());|
});
// different set of values each time
Console.WriteLine(csvString(randomValues));
Console.WriteLine(csvString(randomValues.OrderBy(x => x)));
Console.WriteLine(csvString(randomValues.OrderByDescending(x => x)));
![C: Microsoft Visual Studio Debug Console
System.Linq.
Enumerable+WhereEnumerableIterator 1[System.Int32]
System.Linq. Enumerable+<OfTypeIterator>d_62`1[System. Int32]
3,-2,2,-1,-5,4,1,-3,3,3
-5, -4,-3, -2,0,0,0,2,3,4
4,4,3,2,0,-1,-1,-3,-4, -5
X
C:\Users\keyon\OneDrive\Desktop\LINQ_Lecture\bin\Debug\net5.0\LINQ_Lecture.exe (process 12244) exited with code 0.
To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically
le when debugging stops.
close the conso
Press any key to close this window ...](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc1602b80-c5b7-44a0-838e-9b62644e132e%2F080ec6ec-a571-4fc0-8193-2442b61021f7%2F6zhxtq_processed.png&w=3840&q=75)
Transcribed Image Text:C: Microsoft Visual Studio Debug Console
System.Linq.
Enumerable+WhereEnumerableIterator 1[System.Int32]
System.Linq. Enumerable+<OfTypeIterator>d_62`1[System. Int32]
3,-2,2,-1,-5,4,1,-3,3,3
-5, -4,-3, -2,0,0,0,2,3,4
4,4,3,2,0,-1,-1,-3,-4, -5
X
C:\Users\keyon\OneDrive\Desktop\LINQ_Lecture\bin\Debug\net5.0\LINQ_Lecture.exe (process 12244) exited with code 0.
To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically
le when debugging stops.
close the conso
Press any key to close this window ...
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
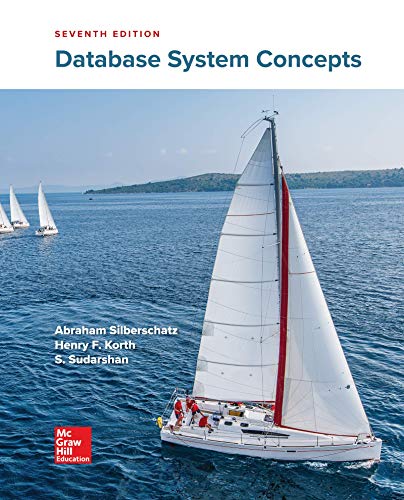
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
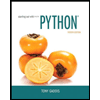
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
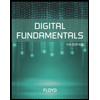
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
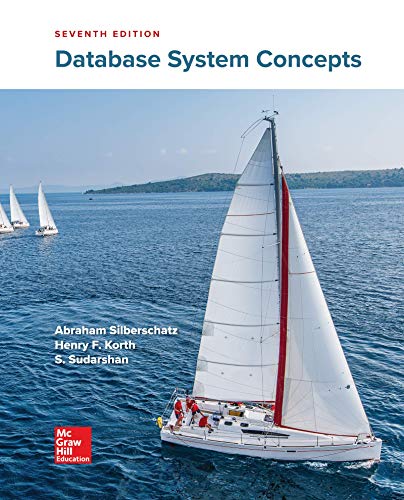
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
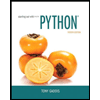
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
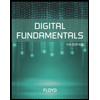
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
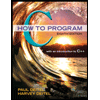
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
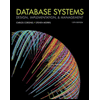
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
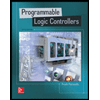
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education