gram that reads a file containing a list of songs and prints the songs to the screen one at a time. After each song is printed, except for the last song, the program asks the user to press enter for more. After the last song, the program should say that this was the last song and quit. If there were no songs in the file to begin with, the program should say that there are no songs to show and quit. The program should begin by asking the user for the name of the input file. Each song consists of a title, artist and year. In the file, each song is given on three consecutive lines. Create this program using the latest versi
Can you help me write a C++ Program that does the following:
Create a program that reads a file containing a list of songs and
prints the songs to the screen one at a time. After each song is printed,
except for the last song, the program asks the user to press enter for more.
After the last song, the program should say that this was the last song and
quit. If there were no songs in the file to begin with, the program should
say that there are no songs to show and quit.
The program should begin by asking the user for the name of the input file.
Each song consists of a title, artist and year.
In the file, each song is given on three consecutive lines.
Create this program using the latest version of the class Song you created. The latest version of the class song I created is below:
#include <iostream>
#include <string>
using namespace std;
class Song {
private:
string Title;
string Artist;
int Year;
public:
Song() : Title("invalid"), Artist("invalid"), Year(-1) {}
Song(const string &Title) : Title(Title), Artist("unknown"), Year(-1) {}
Song(const string &Title, const string &Artist, int Year)
: Title(Title), Artist(Artist), Year(Year) {}
void set_title(const string &title) { Title = title; }
void set_artist(const string &artist) { Artist = artist; }
void set_year(int year) { Year = year; }
void set(const string &title, const string &artist, int year) {
Title = title;
Artist = artist;
Year = year;
}
bool operator==(const Song &other) const {
return Title == other.Title && Artist == other.Artist && Year == other.Year;
}
bool operator<(const Song &other) const {
if (Title < other.Title)
return true;
else if (Title == other.Title) {
if (Artist < other.Artist)
return true;
else if (Artist == other.Artist) {
return Year < other.Year;
}
}
return false;
}
friend std::ostream &operator<<(std::ostream &out, const Song &song) {
out << song.Title << " by " << song.Artist << " (" << song.Year << ")";
return out;
}
friend std::istream &operator>>(std::istream &in, Song &song) {
std::string title, artist;
int year;
in >> title >> artist >> year;
song.set(title, artist, year);
return in;
}
const std::string &get_title() const { return Title; }
const std::string &get_artist() const { return Artist; }
int get_year() const { return Year; }
};

Step by step
Solved in 3 steps with 1 images

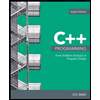
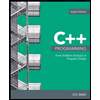