This codes not complie it can you fix the code can complie it? import java.util.ArrayList; public class Heap { void heapify(ArrayList hT, int i) { int size = hT.size(); int largest = i; int l = 2 * i + 1; int r = 2 * i + 2; if (l < size && hT.get(l) > hT.get(largest)) largest = l; if (r < size && hT.get(r) > hT.get(largest)) largest = r; if (largest != i) { int temp = hT.get(largest); hT.set(largest, hT.get(i)); hT.set(i, temp); heapify(hT, largest); } } void insert(ArrayList hT, int newNum) { int size = hT.size(); if (size == 0) { hT.add(newNum); } else { hT.add(newNum); for (int i = size / 2 - 1; i >= 0; i--) { heapify(hT, i); } } } void deleteNode(ArrayList hT, int num) { int size = hT.size(); int i; for (i = 0; i < size; i++) { if (num == hT.get(i)) break; } int temp = hT.get(i); hT.set(i, hT.get(size-1)); hT.set(size-1, temp); hT.remove(size-1); for (int j = size / 2 - 1; j >= 0; j--) { heapify(hT, j); } } void printArray(ArrayList array, int size) { for (Integer i : array) { System.out.print(i + " "); } class Main{ public static void main(String args[]) { ArrayList array = new ArrayList(); int size = array.size(); Heap h = new Heap(); h.insert(array, 3); h.insert(array, 4); h.insert(array, 9); h.insert(array, 5); h.insert(array, 2); System.out.println("Max-Heap array: "); h.printArray(array, size); h.deleteNode(array, 4); System.out.println("After deleting an element: "); h.printArray(array, size); } }
This codes not complie it can you fix the code can complie it? import java.util.ArrayList; public class Heap { void heapify(ArrayList hT, int i) { int size = hT.size(); int largest = i; int l = 2 * i + 1; int r = 2 * i + 2; if (l < size && hT.get(l) > hT.get(largest)) largest = l; if (r < size && hT.get(r) > hT.get(largest)) largest = r; if (largest != i) { int temp = hT.get(largest); hT.set(largest, hT.get(i)); hT.set(i, temp); heapify(hT, largest); } } void insert(ArrayList hT, int newNum) { int size = hT.size(); if (size == 0) { hT.add(newNum); } else { hT.add(newNum); for (int i = size / 2 - 1; i >= 0; i--) { heapify(hT, i); } } } void deleteNode(ArrayList hT, int num) { int size = hT.size(); int i; for (i = 0; i < size; i++) { if (num == hT.get(i)) break; } int temp = hT.get(i); hT.set(i, hT.get(size-1)); hT.set(size-1, temp); hT.remove(size-1); for (int j = size / 2 - 1; j >= 0; j--) { heapify(hT, j); } } void printArray(ArrayList array, int size) { for (Integer i : array) { System.out.print(i + " "); } class Main{ public static void main(String args[]) { ArrayList array = new ArrayList(); int size = array.size(); Heap h = new Heap(); h.insert(array, 3); h.insert(array, 4); h.insert(array, 9); h.insert(array, 5); h.insert(array, 2); System.out.println("Max-Heap array: "); h.printArray(array, size); h.deleteNode(array, 4); System.out.println("After deleting an element: "); h.printArray(array, size); } }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 3PE
Related questions
Question
This codes not complie it can you fix the code can complie it?
import java.util.ArrayList;
public class Heap {
void heapify(ArrayList hT, int i) {
int size = hT.size();
int largest = i;
int l = 2 * i + 1;
int r = 2 * i + 2;
if (l < size && hT.get(l) > hT.get(largest))
largest = l;
if (r < size && hT.get(r) > hT.get(largest))
largest = r;
if (largest != i) {
int temp = hT.get(largest);
hT.set(largest, hT.get(i));
hT.set(i, temp);
heapify(hT, largest);
}
}
void insert(ArrayList hT, int newNum) {
int size = hT.size();
if (size == 0) {
hT.add(newNum);
} else {
hT.add(newNum);
for (int i = size / 2 - 1; i >= 0; i--) {
heapify(hT, i);
}
}
}
void deleteNode(ArrayList hT, int num) {
int size = hT.size();
int i;
for (i = 0; i < size; i++) {
if (num == hT.get(i))
break;
}
int temp = hT.get(i);
hT.set(i, hT.get(size-1));
hT.set(size-1, temp);
hT.remove(size-1);
for (int j = size / 2 - 1; j >= 0; j--) {
heapify(hT, j);
}
}
void printArray(ArrayList array, int size) {
for (Integer i : array) {
System.out.print(i + " ");
}
class Main{
public static void main(String args[]) {
ArrayList array = new ArrayList();
int size = array.size();
Heap h = new Heap();
h.insert(array, 3);
h.insert(array, 4);
h.insert(array, 9);
h.insert(array, 5);
h.insert(array, 2);
System.out.println("Max-Heap array: ");
h.printArray(array, size);
h.deleteNode(array, 4);
System.out.println("After deleting an element: ");
h.printArray(array, size);
}
}
![Heap data structure called to be a complete binary tree while maintaining the heap property,
where given node is
always greater than its child node/s and the key of the root node is the largest
among all other nodes. This property is also called max heap property.
always smaller than the child node/s and the key of the root node is the smallest
among all other nodes. This property is also called min heap property.
You are given a code with Pseudo code. Knowing the properties from the Chapter, write the
logic for following methos- heapify, insert, deleteNode
import java.util.ArrayList;
public class Heap {
Max Heap Lab
void heapify(ArrayList<Integer> hT, int i) {
/* Heapify(array, size, i)
set i as largest
leftChild 21 + 1
rightChild 21 + 2
*/
}
void
*/
if leftChild > array [largest]
set leftChildIndex as largest
if rightChild > array[largest]
set rightChild Index as largest
swap array[i] and array [largest]
To create a Max-Heap:
}
void insert (ArrayList<Integer> hT, int newNum) {
/* If there is no node,
MaxHeap (array, size)
loop from the first index of non-leaf node down to zero.
call heapify
create a newNode.
else (a node is already present)
insert the newNode at the end (last node from left to right.)
heapify the array
deleteNode(ArrayList<Integer> hT, int num) {
/* If nodeToBeDeleted is the leafNode
remove the node
Else swap nodeToBeDeleted with the lastLeafNode
remove noteToBeDeleted
heapify the array
}
void printArray(ArrayList<Integer> array, int size) {
3
+](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6dc011f-ed7d-4edd-97c9-d61f64950d9d%2Fd63a0124-d4f6-4540-8dab-2205b8e670fc%2Fmxwrfmd_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Heap data structure called to be a complete binary tree while maintaining the heap property,
where given node is
always greater than its child node/s and the key of the root node is the largest
among all other nodes. This property is also called max heap property.
always smaller than the child node/s and the key of the root node is the smallest
among all other nodes. This property is also called min heap property.
You are given a code with Pseudo code. Knowing the properties from the Chapter, write the
logic for following methos- heapify, insert, deleteNode
import java.util.ArrayList;
public class Heap {
Max Heap Lab
void heapify(ArrayList<Integer> hT, int i) {
/* Heapify(array, size, i)
set i as largest
leftChild 21 + 1
rightChild 21 + 2
*/
}
void
*/
if leftChild > array [largest]
set leftChildIndex as largest
if rightChild > array[largest]
set rightChild Index as largest
swap array[i] and array [largest]
To create a Max-Heap:
}
void insert (ArrayList<Integer> hT, int newNum) {
/* If there is no node,
MaxHeap (array, size)
loop from the first index of non-leaf node down to zero.
call heapify
create a newNode.
else (a node is already present)
insert the newNode at the end (last node from left to right.)
heapify the array
deleteNode(ArrayList<Integer> hT, int num) {
/* If nodeToBeDeleted is the leafNode
remove the node
Else swap nodeToBeDeleted with the lastLeafNode
remove noteToBeDeleted
heapify the array
}
void printArray(ArrayList<Integer> array, int size) {
3
+
![}
}
for (Integer i : array) {
System.out.print(i + " ");
}
}
System.out.println();
public static void main(String args[]) {
ArrayList<Integer> array = new ArrayList<Integer>();
int size = array.size();
Heap h = new Heap();
h.insert (array, 3);
h.insert (array, 4);
h.insert (array, 9);
h.insert (array, 5);
h.insert (array, 2);
System.out.println("Max-Heap array: ");
h.printArray(array, size);
h.deleteNode (array, 4);
System.out.println("After deleting an element: ");
h.printArray(array, size);
Put all your work in ONE Zip file and submit it in Canvas.
Zip filename should be in format of FirstLast_Lab6.zip (ex. John Doe_Lab6.zip)
Zip file should contain 2 files only:
(1) Heap.java
(2) Output.docx (screenshot of input and output)
< ~\3 >
A](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6dc011f-ed7d-4edd-97c9-d61f64950d9d%2Fd63a0124-d4f6-4540-8dab-2205b8e670fc%2F0tuzubh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:}
}
for (Integer i : array) {
System.out.print(i + " ");
}
}
System.out.println();
public static void main(String args[]) {
ArrayList<Integer> array = new ArrayList<Integer>();
int size = array.size();
Heap h = new Heap();
h.insert (array, 3);
h.insert (array, 4);
h.insert (array, 9);
h.insert (array, 5);
h.insert (array, 2);
System.out.println("Max-Heap array: ");
h.printArray(array, size);
h.deleteNode (array, 4);
System.out.println("After deleting an element: ");
h.printArray(array, size);
Put all your work in ONE Zip file and submit it in Canvas.
Zip filename should be in format of FirstLast_Lab6.zip (ex. John Doe_Lab6.zip)
Zip file should contain 2 files only:
(1) Heap.java
(2) Output.docx (screenshot of input and output)
< ~\3 >
A
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Exception in thread "main" java.lang.Error: Unresolved compilation problem:
at Main.main(Heap.java:66)
what this mean is?
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
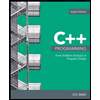
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
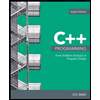
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning