def wear_a_jacket(us_zip:str) -> bool: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. The purpose of this function is to inform you if you should wear a jacket or not. It will be given a US zip code. This function should query the API to figure out the current temperature in the region associated with the US zip code. If the temperature is less than 60 degrees Fahrenheit (main.feels_like float: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. This function should retrieve the historical temperature in Fahrenheit at a particular US location. You will be given a number of days, hours, and minutes and a United States zip code. The time values given are the cumulative total time in the past minus the current time, you should retrieve the historical temperature (current.temp). In other words, if the parameter days is 4 hours is 0 and minutes is 5. Then you should return the temperature 4 days and 5 minutes ago at the specified zip code. Since the open weather API does not have a method querying for historical weather with a zip code, please make use of this git hub document that maps US zip codes to latitude and longitude. https://gist.githubusercontent.com/erichurst/7882666/raw/5bdc46db47d9515269ab12ed6fb2850377fd869e/US%2520Zip%2520Codes%2520from%25202013%2520Government%2520Data Keep in mind, you might need to remove erroneous white space characters from the beginning and end of the latitude and longitude values in this document after splitting the line with a comma delimiter ",". You might find it helpful to use the following API handle(s): http://api.openweathermap.org/data/2.5/onecall/timemachine - to retrieve past weather information def borders(country_name_a: str, country_name_b: str) -> bool: Using the https://restcountries.com/ API, this function will answer the question, are two countries neighbors? The function will be given two arguments country_name_a and country_name_b. Both arguments are partial names of countries. The function's job is to find all nations with a name that contains country_name_a. Then check whether any countries are neighbors of the matching countries for country_name_b. One challenging aspect of this problem is that the country's name will not be complete. For example, the partial country name may be "united" which will yield a long list of possible countries because many countries' official names include the word "united" for example, United States of America, United States Minor Outlying Islands, United Republic of Tanzania, United Mexican States, United Arab Emirates, and many more. Your goal is to check if the specified language is spoken in any countries that match the partial name. Use the endpoint https://restcountries.com/v3.1/name/{name} to get a complete list of countries with the partial name in their official name (replace {name} with the actual country_partial_name argument's value). The endpoint https://restcountries.com/v3.1/name/{name} will return a lot of information about each country. The critical bits you want to look at are the keys "borders" and "cca3". The key "borders" will tell you which country(s) border the given nation. The value associated with the key "borders" will be a list of 3-letter country codes. Remember that the key "border" will not be in the dictionary if the nation has no neighbors because it's an island. The key "cca3" will tell you the 3-letter country code for the given nation. The function should return True if a country matching country_name_a borders a country matching country_name_b. Example: assert borders("united", "united") assert borders("sweden", "republic") assert not borders("united", "tanzania") assert not borders("british", "sweden")
def wear_a_jacket(us_zip:str) -> bool: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. The purpose of this function is to inform you if you should wear a jacket or not. It will be given a US zip code. This function should query the API to figure out the current temperature in the region associated with the US zip code. If the temperature is less than 60 degrees Fahrenheit (main.feels_like float: This function should use https://openweathermap.org/ API (user account required) to collect its weather information. This function should retrieve the historical temperature in Fahrenheit at a particular US location. You will be given a number of days, hours, and minutes and a United States zip code. The time values given are the cumulative total time in the past minus the current time, you should retrieve the historical temperature (current.temp). In other words, if the parameter days is 4 hours is 0 and minutes is 5. Then you should return the temperature 4 days and 5 minutes ago at the specified zip code. Since the open weather API does not have a method querying for historical weather with a zip code, please make use of this git hub document that maps US zip codes to latitude and longitude. https://gist.githubusercontent.com/erichurst/7882666/raw/5bdc46db47d9515269ab12ed6fb2850377fd869e/US%2520Zip%2520Codes%2520from%25202013%2520Government%2520Data Keep in mind, you might need to remove erroneous white space characters from the beginning and end of the latitude and longitude values in this document after splitting the line with a comma delimiter ",". You might find it helpful to use the following API handle(s): http://api.openweathermap.org/data/2.5/onecall/timemachine - to retrieve past weather information def borders(country_name_a: str, country_name_b: str) -> bool: Using the https://restcountries.com/ API, this function will answer the question, are two countries neighbors? The function will be given two arguments country_name_a and country_name_b. Both arguments are partial names of countries. The function's job is to find all nations with a name that contains country_name_a. Then check whether any countries are neighbors of the matching countries for country_name_b. One challenging aspect of this problem is that the country's name will not be complete. For example, the partial country name may be "united" which will yield a long list of possible countries because many countries' official names include the word "united" for example, United States of America, United States Minor Outlying Islands, United Republic of Tanzania, United Mexican States, United Arab Emirates, and many more. Your goal is to check if the specified language is spoken in any countries that match the partial name. Use the endpoint https://restcountries.com/v3.1/name/{name} to get a complete list of countries with the partial name in their official name (replace {name} with the actual country_partial_name argument's value). The endpoint https://restcountries.com/v3.1/name/{name} will return a lot of information about each country. The critical bits you want to look at are the keys "borders" and "cca3". The key "borders" will tell you which country(s) border the given nation. The value associated with the key "borders" will be a list of 3-letter country codes. Remember that the key "border" will not be in the dictionary if the nation has no neighbors because it's an island. The key "cca3" will tell you the 3-letter country code for the given nation. The function should return True if a country matching country_name_a borders a country matching country_name_b. Example: assert borders("united", "united") assert borders("sweden", "republic") assert not borders("united", "tanzania") assert not borders("british", "sweden")
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
def wear_a_jacket(us_zip:str) -> bool:
This function should use https://openweathermap.org/ API (user account required) to collect its weather information. The purpose of this function is to inform you if you should wear a jacket or not. It will be given a US zip code. This function should query the API to figure out the current temperature in the region associated with the US zip code. If the temperature is less than 60 degrees Fahrenheit (main.feels_like float:
This function should use https://openweathermap.org/ API (user account required) to collect its weather information. This function should retrieve the historical temperature in Fahrenheit at a particular US location. You will be given a number of days, hours, and minutes and a United States zip code. The time values given are the cumulative total time in the past minus the current time, you should retrieve the historical temperature (current.temp). In other words, if the parameter days is 4 hours is 0 and minutes is 5. Then you should return the temperature 4 days and 5 minutes ago at the specified zip code.
Since the open weather API does not have a method querying for historical weather with a zip code, please make use of this git hub document that maps US zip codes to latitude and longitude. https://gist.githubusercontent.com/erichurst/7882666/raw/5bdc46db47d9515269ab12ed6fb2850377fd869e/US%2520Zip%2520Codes%2520from%25202013%2520Government%2520Data Keep in mind, you might need to remove erroneous white space characters from the beginning and end of the latitude and longitude values in this document after splitting the line with a comma delimiter ",".
You might find it helpful to use the following API handle(s):
http://api.openweathermap.org/data/2.5/onecall/timemachine - to retrieve past weather information
def borders(country_name_a: str, country_name_b: str) -> bool:
Using the https://restcountries.com/ API, this function will answer the question, are two countries neighbors? The function will be given two arguments country_name_a and country_name_b. Both arguments are partial names of countries. The function's job is to find all nations with a name that contains country_name_a. Then check whether any countries are neighbors of the matching countries for country_name_b.
One challenging aspect of this problem is that the country's name will not be complete. For example, the partial country name may be "united" which will yield a long list of possible countries because many countries' official names include the word "united" for example, United States of America, United States Minor Outlying Islands, United Republic of Tanzania, United Mexican States, United Arab Emirates, and many more. Your goal is to check if the specified language is spoken in any countries that match the partial name. Use the endpoint https://restcountries.com/v3.1/name/{name} to get a complete list of countries with the partial name in their official name (replace {name} with the actual country_partial_name argument's value).
The endpoint https://restcountries.com/v3.1/name/{name} will return a lot of information about each country. The critical bits you want to look at are the keys "borders" and "cca3". The key "borders" will tell you which country(s) border the given nation. The value associated with the key "borders" will be a list of 3-letter country codes. Remember that the key "border" will not be in the dictionary if the nation has no neighbors because it's an island. The key "cca3" will tell you the 3-letter country code for the given nation.
The function should return True if a country matching country_name_a borders a country matching country_name_b.
Example:
assert borders("united", "united")
assert borders("sweden", "republic")
assert not borders("united", "tanzania")
assert not borders("british", "sweden")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
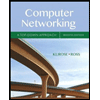
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
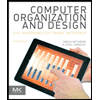
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
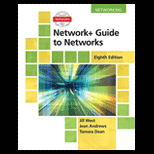
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
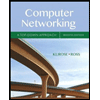
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
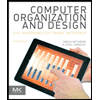
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
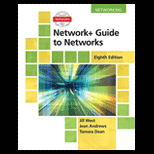
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
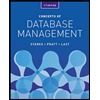
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
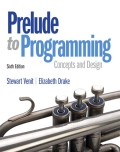
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
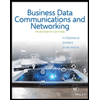
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY