create an automobile class for a dealership. include fields fo an ID number, model, make, color, year and miles per gallon. include get and set methods for each field. do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. do not allow the year to be earlier than 2005 or later than 2019, if it is, set year to 0. do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. include a constructor that accepts arguments for each field value and uses the set methods to assign the values.
create an automobile class for a dealership. include fields fo an ID number, model, make, color, year and miles per gallon. include get and set methods for each field. do not allow the ID to be negative or more than 9999; if it is, set the ID to 0. do not allow the year to be earlier than 2005 or later than 2019, if it is, set year to 0. do not allow the miles per gallon to be less than 10 or more than 60; if it is, set the miles per gallon to 0. include a constructor that accepts arguments for each field value and uses the set methods to assign the values.

import java.util.*;
// class is created
class Automobile
{
//declaration of variables
int ID;
String make;
String model;
String color;
int year;
int vin_no;
double milespergallon;
double speed;
//default constructor is created
Automobile()
{
ID = 0;
make = "";
model = "";
color = "";
year = 0;
vin_no =0;
milespergallon = 0;
speed = 0;
}
//creattion of constructor with parameters
Automobile(int id,String m,String mo,String c,int y,int v, double mil, double s)
{
// set method id created
setID(id);
setMake(m);
setModel(mo);
setColor(c);
setYear(y);
setVIN_NO(v);
setMilespergallon(mil);
setSpeed(s);
}
public double getSpeed()
{
return speed;
}
public void setSpeed(double s)
{
speed = s;
}
public int getVIN_NO()
{
return vin_no;
}
public void setVIN_NO(int v)
{
vin_no = v;
}
public String getModel()
{
return model;
}
public void setModel(String m)
{
model = m;
}
public String getMake()
{
return make;
}
public void setMake(String s)
{
make = s;
}
public String getColor()
{
return color;
}
public void setColor(String c)
{
color = c;
}
public int getID()
{
return ID;
}
public void setID(int id)
{
if( id <0 || id >= 9999 )
ID = 0;
else
ID = id;
}
public int getYear()
{
return year;
}
public void setYear(int y)
{
if(y <2000 || y >2017)
year = 0;
else
year = y;
}
public double getMilespergallon()
{
return milespergallon;
}
public void setMilespergallon(double m)
{
if(m <10 || m >60)
milespergallon = 0;
else
milespergallon = m;
}
public void Accelerator()
{
speed = speed + 5;
}
public void Accelerator(int s)
{
speed = speed + s;
}
public void Brake()
{
speed = speed - 5;
}
public void Brake(int b)
{
speed = speed - b;
}
public void PrintAuto()
{
System.out.println(getID());
System.out.println(getMake());
System.out.println(getModel());
System.out.println(getColor());
System.out.println(getYear());
System.out.println(getVIN_NO());
System.out.println(getMilespergallon());
System.out.println(getSpeed());
}
public static void main(String args[])
{
Automobile a1 = new Automobile();
Automobile a2 = new Automobile(101,"Audi","CS1999","Black",2067,1245,45,101) ;
Automobile a3 = new Automobile();
a3.setID(202);
a3.setMake("Lamborgini");
a3.setModel("ES2000");
a3.setColor("White");
a3.setYear(2015);
a3.setVIN_NO(1234);
a3.setMilespergallon(150);
a3.setSpeed(100);
System.out.println(" default parameters");
a1.PrintAuto();
System.out.println(" constructor with parameters");
a2.PrintAuto();
System.out.println(" calling of setter methods ");
a3.PrintAuto();
}
}
Step by step
Solved in 2 steps

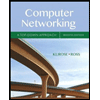
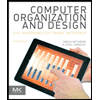
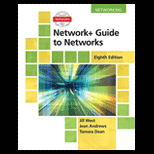
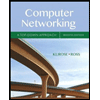
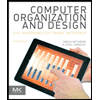
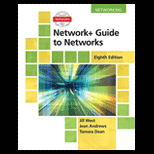
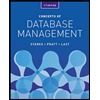
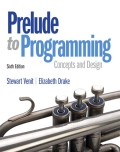
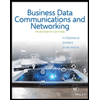