Create a new file in C++ and save it as lab12_XYZ.cpp Consider rolling two six-sided dice. While the result of each throw is independent of all rolls that have come before, we can still calculate the odds of rolling any given number between 2 and 12. This program will calculate those odds by generating a large number (100,000) of random values (i.e. "rolling the dice") and storing the results in an array. When all values have been rolled, we will display the results (counts and odds to 2 decimal places) to the user. x x 2 3 4 5 6 7 8 9 10 11 12 //value for sum of 2 dice rolls +---+---+---+---+---+---+---+---+---+---+--- | | | | | | | | | | | | | | //counters for number of times value is rolled +---+---+---+---+---+---+---+---+---+---+---+---+---+ 0 1 2 3 4 5 6 7 8 9 10 11 12 //array index In Lab 5, sample code was provided for generating a random number. In this lab, I've provided a header file for you to include, getRandomLib.h, which lets you get all kinds of random things. We need some functions: void displayMessage(); //hello, goodbye, etc. int rollDice(); //returns a number between 2 and 12 (by rolling two dice and summing the result) void displayResults(int[]); //displays results in the array HINT: Make sure to initialize counters before starting loop HINT: To calculate odds: odds = count * 100 / NUM_ROLLS; Header comments must be present Prototypes must be present if functions are used Hello and goodbye messages must be shown Array(s) must be used for implementation Results should be formatted neatly (2 decimal places for odds, decimals lined up, etc.) Use comments and good style practices Make sure to #include "getRandomLib.h". Download the attached header file and place in same location as your program. SAMPLE OUTPUT: Welcome! This program will calculate the odds of rolling 2 - 12 with two 6-sided dice. Number of 2s rolled: 270 ( 2.70%) Number of 3s rolled: 556 ( 5.56%) Number of 4s rolled: 814 ( 8.14%) Number of 5s rolled: 1136 (11.36%) Number of 6s rolled: 1379 (13.79%) Number of 7s rolled: 1705 (17.05%) Number of 8s rolled: 1397 (13.97%) Number of 9s rolled: 1140 (11.40%) Number of 10s rolled: 818 ( 8.18%) Number of 11s rolled: 532 ( 5.32%) Number of 12s rolled: 253 ( 2.53%) Exiting program. Goodbye! Attached Files: getRandomLib.h (3.468 KB): #ifndef GET_RANDOM_LIB_H#define GET_RANDOM_LIB_H #include <cstdlib>#include <random>#include <ctime>using namespace std; const int MIN_UPPER = 65;const int MAX_UPPER = 90;const int MIN_LOWER = 97;const int MAX_LOWER = 122;const int MIN_DIGIT = 48;const int MAX_DIGIT = 57;const int MIN_CHAR = 33;const int MAX_CHAR = 126; //unsigned seed = time(0); int getRandomInt(int min, int max) {//seed random enginestatic default_random_engine gen((unsigned int)time(0));// if max < min, then swapif (min > max) {int temp = min;min = max;max = temp;}//set up random generatoruniform_int_distribution<int> dis(min, max); //range//need to throw away the first call, as it is always max...static int firstCall = dis(gen);return dis(gen);} //end getRandomInt() float getRandomFraction() {//seed random enginestatic unsigned seed = time(0);return rand() / static_cast<float>(RAND_MAX);} //end getRandomFraction() float getRandomFloat(float min, float max) {if (min > max) {float temp = min;min = max;max = temp;}return (getRandomFraction() * (max - min)) + min;} //end getRandomFloat() double getRandomDouble(double min, double max) {if (min > max) {double temp = min;min = max;max = temp;}return (static_cast<double>(getRandomFraction()) * (max - min)) + min;} //end getRandomDouble() bool getRandomBool() {if (getRandomInt(0,1) == 0)return false;elsereturn true;} //end getRandomBool char getRandomUpper() {return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER));} //end getRandomUpper() char getRandomUpper(char min, char max) { return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));} //end getRandomUpper() char getRandomLower() {return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER));} //end getRandomLower() char getRandomLower(char min, char max) {return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));} //end getRandomLower() char getRandomAlpha() {if (getRandomBool())return getRandomUpper();elsereturn getRandomLower();} //end getRandomAlpha() char getRandomDigit() {return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT));} //end getRandomDigit() char getRandomDigit(char min, char max) {return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));} //end getRandomDigit() char getRandomChar() {return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR));} //end getRandomChar() #endif
Create a new file in C++ and save it as lab12_XYZ.cpp
- Consider rolling two six-sided dice. While the result of each throw is independent of all rolls that have come before, we can still calculate the odds of rolling any given number between 2 and 12. This
program will calculate those odds by generating a large number (100,000) of random values (i.e. "rolling the dice") and storing the results in an array. When all values have been rolled, we will display the results (counts and odds to 2 decimal places) to the user.
In Lab 5, sample code was provided for generating a random number. In this lab, I've provided a header file for you to include, getRandomLib.h, which lets you get all kinds of random things.
We need some functions:- void displayMessage(); //hello, goodbye, etc.
- int rollDice(); //returns a number between 2 and 12 (by rolling two dice and summing the result)
- void displayResults(int[]); //displays results in the array
HINT: Make sure to initialize counters before starting loop
HINT: To calculate odds: odds = count * 100 / NUM_ROLLS;
- Header comments must be present
- Prototypes must be present if functions are used
- Hello and goodbye messages must be shown
- Array(s) must be used for implementation
- Results should be formatted neatly (2 decimal places for odds, decimals lined up, etc.)
- Use comments and good style practices
Make sure to #include "getRandomLib.h". Download the attached header file and place in same location as your program.
SAMPLE OUTPUT: Welcome! This program will calculate the odds of rolling 2 - 12 with two 6-sided dice.Number of 2s rolled: 270 ( 2.70%) Number of 3s rolled: 556 ( 5.56%) Number of 4s rolled: 814 ( 8.14%) Number of 5s rolled: 1136 (11.36%) Number of 6s rolled: 1379 (13.79%) Number of 7s rolled: 1705 (17.05%) Number of 8s rolled: 1397 (13.97%) Number of 9s rolled: 1140 (11.40%) Number of 10s rolled: 818 ( 8.18%) Number of 11s rolled: 532 ( 5.32%) Number of 12s rolled: 253 ( 2.53%)
Exiting program. Goodbye!
- getRandomLib.h (3.468 KB):
#ifndef GET_RANDOM_LIB_H
#define GET_RANDOM_LIB_H
#include <cstdlib>
#include <random>
#include <ctime>
using namespace std;
const int MIN_UPPER = 65;
const int MAX_UPPER = 90;
const int MIN_LOWER = 97;
const int MAX_LOWER = 122;
const int MIN_DIGIT = 48;
const int MAX_DIGIT = 57;
const int MIN_CHAR = 33;
const int MAX_CHAR = 126;
//unsigned seed = time(0);
int getRandomInt(int min, int max) {
//seed random engine
static default_random_engine gen((unsigned int)time(0));
// if max < min, then swap
if (min > max) {
int temp = min;
min = max;
max = temp;
}
//set up random generator
uniform_int_distribution<int> dis(min, max); //range
//need to throw away the first call, as it is always max...
static int firstCall = dis(gen);
return dis(gen);
} //end getRandomInt()
float getRandomFraction() {
//seed random engine
static unsigned seed = time(0);
return rand() / static_cast<float>(RAND_MAX);
} //end getRandomFraction()
float getRandomFloat(float min, float max) {
if (min > max) {
float temp = min;
min = max;
max = temp;
}
return (getRandomFraction() * (max - min)) + min;
} //end getRandomFloat()
double getRandomDouble(double min, double max) {
if (min > max) {
double temp = min;
min = max;
max = temp;
}
return (static_cast<double>(getRandomFraction()) * (max - min)) + min;
} //end getRandomDouble()
bool getRandomBool() {
if (getRandomInt(0,1) == 0)
return false;
else
return true;
} //end getRandomBool
char getRandomUpper() {
return static_cast<char>(getRandomInt(MIN_UPPER, MAX_UPPER));
} //end getRandomUpper()
char getRandomUpper(char min, char max) {
return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));
} //end getRandomUpper()
char getRandomLower() {
return static_cast<char>(getRandomInt(MIN_LOWER, MAX_LOWER));
} //end getRandomLower()
char getRandomLower(char min, char max) {
return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));
} //end getRandomLower()
char getRandomAlpha() {
if (getRandomBool())
return getRandomUpper();
else
return getRandomLower();
} //end getRandomAlpha()
char getRandomDigit() {
return static_cast<char>(getRandomInt(MIN_DIGIT, MAX_DIGIT));
} //end getRandomDigit()
char getRandomDigit(char min, char max) {
return static_cast<char>(getRandomInt(static_cast<int>(min), static_cast<int>(max)));
} //end getRandomDigit()
char getRandomChar() {
return static_cast<char>(getRandomInt(MIN_CHAR, MAX_CHAR));
} //end getRandomChar()
#endif

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

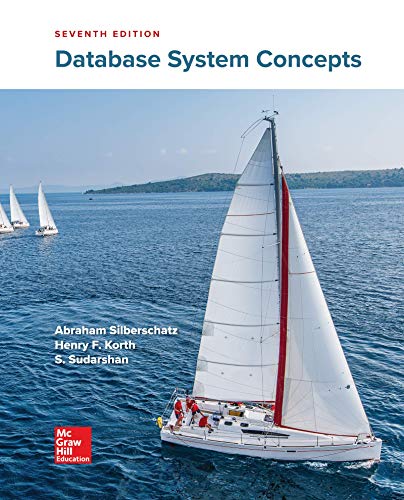
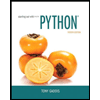
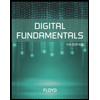
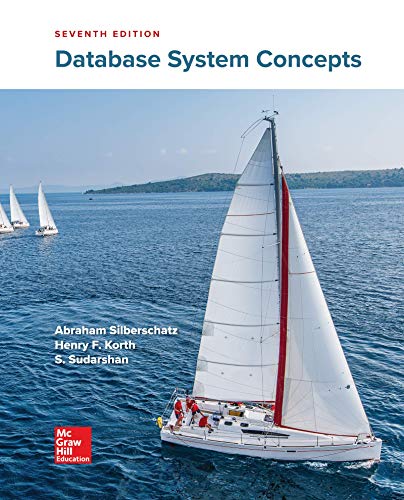
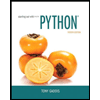
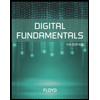
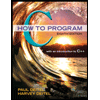
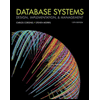
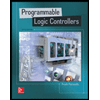