Create a complete program that contains the following: a) Create a looping menu with the following options: 1. Calculate n! (n factorial). 2. Calculate n to the power of m. 3. Exit program. b) If option 1 is selected: - Request an integer value for n from 1 to 9 inclusive. - Validate that n is an integer. Re-enter n if n is not an integer. - Validate that n is less than 10. Re-enter n if n is not in the range. - Calculate and output n factorial (n!). I c) If option 2 is selected: Request integer values for n and m from 1 to 9 inclusive. - Validate that n and m are both integers Re-enter n and m if one of them is not an integer. - Validate that both n and m are from 1 to 9 inclusive. Re-enter n and m if one of them is not in the range. - Calculate and output n to the power of m. d) If option 3 is selected: - Exit the program. e) If an invalid option is selected: - Inform the user with the error. Re-enter option. f) Use loops to create factorial and power operations: - Do not use library functions to do them. g) Use validate() function to validate all the input that is of improper type such as characters, symbols, or floats. 1) You may create your own factorial () and power () functions. any math functions such as pow (x, y) from library. 2) Add comments to explain the algorithm of your program. 3) Keep your project coding clear with your own programming style. But do not use
Create a complete program that contains the following: a) Create a looping menu with the following options: 1. Calculate n! (n factorial). 2. Calculate n to the power of m. 3. Exit program. b) If option 1 is selected: - Request an integer value for n from 1 to 9 inclusive. - Validate that n is an integer. Re-enter n if n is not an integer. - Validate that n is less than 10. Re-enter n if n is not in the range. - Calculate and output n factorial (n!). I c) If option 2 is selected: Request integer values for n and m from 1 to 9 inclusive. - Validate that n and m are both integers Re-enter n and m if one of them is not an integer. - Validate that both n and m are from 1 to 9 inclusive. Re-enter n and m if one of them is not in the range. - Calculate and output n to the power of m. d) If option 3 is selected: - Exit the program. e) If an invalid option is selected: - Inform the user with the error. Re-enter option. f) Use loops to create factorial and power operations: - Do not use library functions to do them. g) Use validate() function to validate all the input that is of improper type such as characters, symbols, or floats. 1) You may create your own factorial () and power () functions. any math functions such as pow (x, y) from library. 2) Add comments to explain the algorithm of your program. 3) Keep your project coding clear with your own programming style. But do not use
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter7: User-defined Simple Data Types, Namespaces, And The String Type
Section: Chapter Questions
Problem 7PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
100%
Please answer in c++ with showing code
1st image is the ques... 2nd image is the output t.
Also show me the code

Transcribed Image Text:Create a complete program that contains the following:
1
a) Create a looping menu with the following options:
1. Calculate n! (n factorial).
2. Calculate n to the power of m.
3. Exit program.
b) If option 1 is selected:
Request an integer value for n from 1 to 9 inclusive.
- Validate that n is an integer.
Re-enter n if n is not an integer.
Validate that n is less than 10.
Re-enter n if n is not in the range.
- Calculate and output n factorial (n!).
X
c) If option 2 is selected:
Request integer values for n and m from 1 to 9 inclusive.
Validate that n and m are both integers
Re-enter n and m if one of them is not an integer.
Validate that both n and m are from 1 to 9 inclusive.
Re-enter n and m if one of them is not in the range.
- Calculate and output n to the power of m.
d) If option 3 is selected:
- Exit the program.
e) If an invalid option is selected:
- Inform the user with the error.
- Re-enter option.
f) Use loops to create factorial and power operations:
- Do not use library functions to do them.
g) Use validate() function to validate all the input that is of
improper type such as characters, symbols, or floats.
1) You may create your own factorial () and power () functions. But do not use
any math functions such as pow (x, y) from <cmath> library.
2) Add comments to explain the algorithm of your program.
3) Keep your project coding clear with your own programming style.

Transcribed Image Text:6:56
< Back Project1_mathMenuValidation.pdf
MATH MENU
1. Calculate n? <n factorial).
2. Calculate n to the m power.
3. Exit program.
Please enter your selection: 0
Invalid option. Please re-enter.
1. Calculate n? <n factorial).
2. Calculate n to the m power.
3. Exit program.
Please enter your selection: 1
Enter an integer value for n (1-9): 2.2
Invalid input. Please re-enter.
Enter an integer value for n (1-9): -2
Invalid input. Please re-enter.
Enter an integer value for n (1-9): 2
2 = 2
1. Calculate n? <n factorial).
2. Calculate n to the m power.
3. Exit program.
Please enter your selection: 2
Enter an integer value for n (1-9): 1.1
Invalid input. Please re-enter.
Enter an integer value for n (1-9): 0
Enter an integer value for n (1-9): 11
Invalid input. Please re-enter.
Enter an integer value for n (1-9): 10
Enter an integer value for m (1-9): 2
Invalid input. Please re-enter.
Enter an integer value for n (1-9): 2
Enter an integer value for m (1-9): 3
2 to the power of 3 - 8
1. Calculate n! <n factorial).
2. Calculate n to the m power.
3. Exit program.
Please enter your selection: 2
Enter an integer value for n (1-9): 5
Enter an integer value for m (1-9): 5
5 to the power of 5 3125
1. Calculate n? <n factorial).
2. Calculate n to the n power.
3. Exit program.
Please enter your selection: 1
Enter an integer value for n (1-9): 5
5 120
1. Calculate n! <n factorial).
2. Calculate n to the m power.
3. Exit program.
Please enter your selection: 3
Exit program.
Math is fun. Programming is even more fun!
65
Q
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
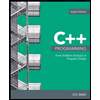
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
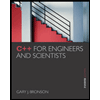
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
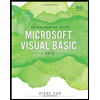
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
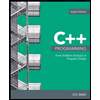
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
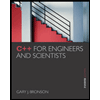
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
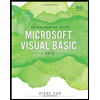
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning