Construct a class named Square that has a floating-point data member named side. The class should have a zero-argument constructor that initializes this data member to 0. It should have member functions named calcPerimeter() and calcArea() that calculate the perimeter and area of a square respectively, a member function setSide() to set the side of the square, a member function getSide() to return the side, and a member function showData() that displays the square’s side, perimeter, and area. The formula for the area of a square is Area = side * side. The formula for the perimeter of a square is Perimeter = 4 * side. The class should use appropriate protection levels for the member data and functions. It should also follow “principles of minimalization”: that is, no member data should be part of a class unless it is needed by most member functions of the object. Use your class in a program that creates an instance of a Square (utilizing the zero-argument constructor), prompts a user for a side, calls the setSide() function to set the square’s side, and then calls showData() to display the square’s side, perimeter, and area. Your program should allow the user to enter new square dimensions until the user enters -1. Be sure to include appropriate error checking. you should ensure that the user enters numeric data for the side. Negative numbers (other than the -1 to exit) should also be prevented.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Construct a class named Square that has a floating-point data member named side. The class should have a zero-argument constructor that initializes this data member to 0. It should have member functions named calcPerimeter() and calcArea() that calculate the perimeter and area of a square respectively, a member function setSide() to set the side of the square, a member function getSide() to return the side, and a member function showData() that displays the square’s side, perimeter, and area. The formula for the area of a square is Area = side * side. The formula for the perimeter of a square is Perimeter = 4 * side.
The class should use appropriate protection levels for the member data and functions. It should also follow “principles of minimalization”: that is, no member data should be part of a class unless it is needed by most member functions of the object.
Use your class in a
- Your lab should be constructed such that separate files are used: Square.h (your class declaration file), Square.cpp (your class implementation file), and SquareDriver.cpp (the file that contains main() and any other functions that are not part of the class).
- All "get" functions should be declared as constant. In fact, any function that does not change the data in the data members should be declared as constant.
- showData() should not refer to the data members directly. Instead, it should call getSide() to retrieve its value
- CalcPerimeter, CalcArea, and ShowData should not have the side passed in as an argument because side is a data member of the class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 6 images

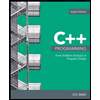
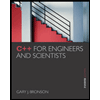
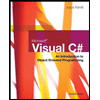
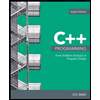
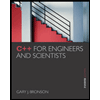
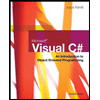