Complete the code provided below, so that it : Accepts strings from the user till the user enters "End!", and creates a BST from them with respect to the order of their entry if the user enters "End!" as the first input, then it should: Display "BST needs at least one node, enter a valid input please!" Accepts strings from the user till the user enters "End!" Next, it should display "which node would you pick?", and accept a string (i.e. the key attribute for this Node) from user as an input. Then, display the distance between the given node from the root of the BST. The distance between root and a node can be calculated by the number of edges between them If the string (ie node) is not found then: Your code should display "Not Found!" Then, your code should display: ' Would you like to choose another node? Y/N' If the user enters 'N' end the program, otherwise let the user choose another node and display the distance for the new node. The program should continue till the user chooses 'N' as a response to the above question. class Node: def __init__(self, key): self.key = key self.left = None self.right = None class BinarySearchTree: def __init__(self): self.root = None def search(self, desired_key): current_node = self.root while current_node is not None: # Return the node if the key matches. if current_node.key == desired_key: return current_node elif desired_key < current_node.key: current_node = current_node.left else: current_node = current_node.right # The key was not found in the tree. return None def insert(self, node): # Check if the tree is empty if self.root is None: self.root = node else: current_node = self.root while current_node is not None: if node.key < current_node.key: if current_node.left is None: current_node.left = node current_node = None else: current_node = current_node.left else: if current_node.right is None: current_node.right = node current_node = None else: current_node = current_node.right def Distance(self,node): # ** Your code goes here ** if __name__ == '__main__': # ** Your code goes here **
Complete the code provided below, so that it :
-
Accepts strings from the user till the user enters "End!", and creates a BST from them with respect to the order of their entry
- if the user enters "End!" as the first input, then it should:
- Display "BST needs at least one node, enter a valid input please!"
- Accepts strings from the user till the user enters "End!"
- if the user enters "End!" as the first input, then it should:
-
Next, it should display "which node would you pick?", and accept a string (i.e. the key attribute for this Node) from user as an input.
-
Then, display the distance between the given node from the root of the BST.
- The distance between root and a node can be calculated by the number of edges between them
- If the string (ie node) is not found then:
- Your code should display "Not Found!"
-
Then, your code should display: ' Would you like to choose another node? Y/N'
- If the user enters 'N' end the program, otherwise let the user choose another node and display the distance for the new node.
-
The program should continue till the user chooses 'N' as a response to the above question.
class Node:
def __init__(self, key):
self.key = key
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def search(self, desired_key):
current_node = self.root
while current_node is not None:
# Return the node if the key matches.
if current_node.key == desired_key:
return current_node
elif desired_key < current_node.key:
current_node = current_node.left
else:
current_node = current_node.right
# The key was not found in the tree.
return None
def insert(self, node):
# Check if the tree is empty
if self.root is None:
self.root = node
else:
current_node = self.root
while current_node is not None:
if node.key < current_node.key:
if current_node.left is None:
current_node.left = node
current_node = None
else:
current_node = current_node.left
else:
if current_node.right is None:
current_node.right = node
current_node = None
else:
current_node = current_node.right
def Distance(self,node):
# ** Your code goes here **
if __name__ == '__main__':
# ** Your code goes here **



Step by step
Solved in 4 steps with 3 images

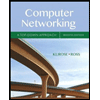
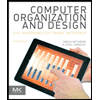
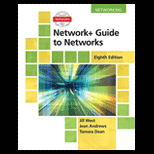
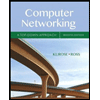
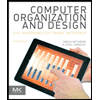
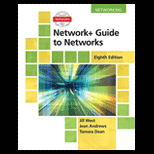
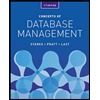
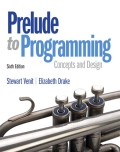
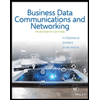