Certain Federal anti-money laundering laws exists that help to thwart illegal transactions and terrorist activities in the US Banking system. Banks must flag certain cash transactions and report them to the proper authorities. To assist the CTU Bank of Laurel, you will write some code to help in this effort by finding any suspicious cash transactions. Assume a classed named BankXA that contains info about a customer's transaction. The class has 3 public attributes: string xaDetail //some detail on the transaction string type // assume the value will always be either "dep" for a deposit or "withd" for withdrawal or "END" if the last element double amt //the amount of the transaction. The amount will always be 0 or more Note that these attributes are public therefore you will not need setters and getters for this exercise. You will retrieve a pointer to an array of some number of BankXA objects. This array contains a flag object or "sentinel record" that indicates the final element in the array. This element will have a type equal to "END" and has xaDetail equal to "END REC". Either of these two values can be used to indicate there are no more records. The amt for this record should be disregarded. Requirements See the numbered comments in the template to assist in completion 1) Write a function called popBadTrans(). This function must return void and take two parameters: A vector passed by reference A BankXA pointer The function will populate the passed-in vector with BankXA objects that are suspicious. A transaction is suspicious if it is a deposit for at least $10,000 or a withdrawal that is at least $5,000. Note that this function must be able to handle 1 - any number of elements as long as the final element is the sentinel record. Do NOT include any output in this function. 2) Retrieve a pointer to an array of BankXA into some variable. This pointer variable should be assigned to the return from the included function named getXAs(). You should not change this function in any way. You will not know haw many elements the returned array has. The final element is marked with the sentinel records documented in the description above. 3) Declare a vector of BankXA. This will be passed to the function described in 1 above. 4) Call the popBadTrans function here passing in the pointer to array of XA as the first parameter, and the vector declared above as the second 5) Output the number of suspicious transactions like: xx suspicious transactions where xx is the number of suspicious transactions found in 4 above. Do NOT put this output in the popBadTrans function itself. 6) Create a function that meets these specs exactly as stated. The function must be called totalDepositAmt() and will take a pointer to a vector of BankXA objects. The function must return the total amount of all the deposit BankXA objects in the vector. If a nullptr is passed to the function, the function must return 0. code main.cpp #include #include #include using namespace std; #include"data.cpp" BankXA *getXAs(); // CHANGE NOTHING ABOVE THIS LINE //1) TODO: Implement your popBadTrans function here //6) TODO: Implement totalDepositAmt function here int main() { //2) Retrieve a pointer to an array of BankXA into some variable //3) Declare a vector of BankXA //4) Call the popBadTrans function here passing in the pointer to array of XA as the first parameter, // and the vector declared above as the second //5) Output the number of suspicious transactions like: //xx suspicious transactions. //, where xx is the number of suspicious transactions found. //6) Test totalDepositAmt here if you like, but remove any output as that will cause one of the tests to fail return 0; } // ****** CHANGE NOTHING BELOW THIS LINE!! BankXA *getXAs(){ BankXA *bxas=new BankXA[10];bxas[3].type="withd";bxas[0].xaDetail="R. Wade getting some $"; bxas[0].type="withd"; //dep, withd bxas[0].amt=45.92;bxas[1].xaDetail="J. Faune getting some $";bxas[4].type="dep";bxas[4].amt=4999.99; bxas[1].type="withd";bxas[2].xaDetail="I. Boesky got cashier's check"; bxas[2].type="withd"; //dep, withd bxas[2].amt=42885.5;bxas[3].xaDetail="S. Ronson getting some $";bxas[3].amt=100.0;bxas[4].xaDetail="E. Thomas cash deposit"; bxas[5].xaDetail="JJ Jameson cash withdrawal"; bxas[5].type="withd";bxas[9].xaDetail="END REC";bxas[9].type="END";bxas[9].amt=0; bxas[5].amt=10000.0;bxas[8].xaDetail="Earl Mason got some $";bxas[8].type="withd";bxas[8].amt=3456.0; bxas[7].xaDetail="P. Ferb getting some $"; bxas[7].type="withd";bxas[7].amt=1487.0;bxas[6].xaDetail="E. Rue made a cash deposit";bxas[6].type="dep"; bxas[6].amt=92317.0; bxas[1].amt=485.0; return bxas; } data.cpp file this file mask as read only #include using namespace std; class BankXA{ public: string xaDetail; string type; //dep, withd double amt; };
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
c++
Certain Federal anti-money laundering laws exists that help to thwart illegal transactions and terrorist activities in the US Banking system. Banks must flag certain cash transactions and report them to the proper authorities. To assist the CTU Bank of Laurel, you will write some code to help in this effort by finding any suspicious cash transactions.
Assume a classed named BankXA that contains info about a customer's transaction. The class has 3 public attributes:
string xaDetail //some detail on the transaction
string type // assume the value will always be either "dep" for a deposit or "withd" for withdrawal or "END" if the last element
double amt //the amount of the transaction. The amount will always be 0 or more
Note that these attributes are public therefore you will not need setters and getters for this exercise.
You will retrieve a pointer to an array of some number of BankXA objects. This array contains a flag object or "sentinel record" that indicates the final element in the array. This element will have a type equal to "END" and has xaDetail equal to "END REC". Either of these two values can be used to indicate there are no more records. The amt for this record should be disregarded.
Requirements
See the numbered comments in the template to assist in completion
code
main.cpp
#include
#include
#include
using namespace std;
#include"data.cpp"
BankXA *getXAs();
// CHANGE NOTHING ABOVE THIS LINE
//1) TODO: Implement your popBadTrans function here
//6) TODO: Implement totalDepositAmt function here
int main()
{
//2) Retrieve a pointer to an array of BankXA into some variable
//3) Declare a vector of BankXA
//4) Call the popBadTrans function here passing in the pointer to array of XA as the first parameter,
// and the vector declared above as the second
//5) Output the number of suspicious transactions like:
//xx suspicious transactions.
//, where xx is the number of suspicious transactions found.
//6) Test totalDepositAmt here if you like, but remove any output as that will cause one of the tests to fail
return 0;
}
// ****** CHANGE NOTHING BELOW THIS LINE!!
BankXA *getXAs(){
BankXA *bxas=new BankXA[10];bxas[3].type="withd";bxas[0].xaDetail="R. Wade getting some $";
bxas[0].type="withd"; //dep, withd
bxas[0].amt=45.92;bxas[1].xaDetail="J. Faune getting some $";bxas[4].type="dep";bxas[4].amt=4999.99;
bxas[1].type="withd";bxas[2].xaDetail="I. Boesky got cashier's check";
bxas[2].type="withd"; //dep, withd
bxas[2].amt=42885.5;bxas[3].xaDetail="S. Ronson getting some $";bxas[3].amt=100.0;bxas[4].xaDetail="E. Thomas cash deposit";
bxas[5].xaDetail="JJ Jameson cash withdrawal";
bxas[5].type="withd";bxas[9].xaDetail="END REC";bxas[9].type="END";bxas[9].amt=0;
bxas[5].amt=10000.0;bxas[8].xaDetail="Earl Mason got some $";bxas[8].type="withd";bxas[8].amt=3456.0;
bxas[7].xaDetail="P. Ferb getting some $";
bxas[7].type="withd";bxas[7].amt=1487.0;bxas[6].xaDetail="E. Rue made a cash deposit";bxas[6].type="dep"; bxas[6].amt=92317.0;
bxas[1].amt=485.0;
return bxas;
}
data.cpp file
this file mask as read only
#include<string>
using namespace std;
class BankXA{
public:
string xaDetail;
string type; //dep, withd
double amt;
};

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

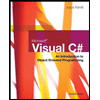
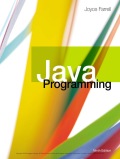
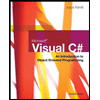
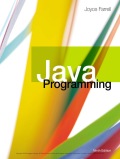