C++ language. Using the coding prepared below, add and create the Undo last pop, Display undo list and Redo Functions void functions but keep them as they are. NOTE: use the coding given, i don't want a new coding The code: #include #include #include using namespace std; struct nodeWords { char words[100]; struct nodeWords *next; }; nodeWords* top; //these pointers are used to hold the linked list. nodeWords* current; //use for traversal nodeWords* newNode; //use to create new node for new record nodeWords* pop; void Push() { char answer; do { newNode = new nodeWords; cout << "\nWrite what's in your mind.." << endl; cout << "Your words > "; cin >> ws; cin.getline(newNode->words, 100); newNode->next = top; top = newNode; cout << "\nWant to write more? Press Y for yes and N for no: "; cin >> answer; } while (toupper(answer) == 'Y'); } void stacktop() { if (top == NULL) { cout << "\nThere is no words!\n"; } else { cout << "\nTHE TOP ELEMENT IS..... "; cout << "\nYour words > " << top->words << endl; } } void display() { char answer2; int no = 1; int totalRecord = 0; int view; { cout << endl << left << setw(5) << "No" << setw(50) << "Your words" << endl; cout << "----------------------------------------------------------------------" << endl; current = top; while (current != NULL) { cout << left << setw(5) << no << setw(50) << current->words << endl; no++; current = current->next; totalRecord++; } cout << "----------------------------------------------------------------------" << endl; cout << "\nTotal Number of Records: " << totalRecord << endl; } cout << "\nPress Y to go back to MAIN MENU: "; cin >> answer2; } void Pop() { current = top; while (current != NULL) { pop = current; if (current == top) { top = top->next; current = NULL; delete pop; cout << "\n\nPop Operation Success!!\n"; } else{ top = NULL; current = NULL; current = NULL; delete pop; cout << "\n\nPop Operation Success!!\n"; } } } int main() { int menuOption; top = NULL; //create list do { cout << "--------------------------------------\n"; cout << "\t\tMain Menu\n"; cout << "--------------------------------------\n"; cout << "1 - Push/Add Record \n"; cout << "2 - Peek/Stack Top \n"; cout << "3 - Pop/Remove Record \n"; cout << "4 - Display\n"; cout << "5 - Undo\n"; cout << "6 - Redo\n"; cout << "7 - Exit\n"; cout << "---------------------------------------\n"; cout << "Enter your Option [1, 2, 3, 4, 5, 6, 7]: "; cin >> menuOption; switch (menuOption) { case 1: Push(); break; case 2: stacktop(); break; case 3: current = top; if (current == NULL) { cout << "\n\n The List is Empty!!\n\n"; cout << "Unable to Perform the Delete Operation.\n\n"; } else { Pop(); } break; case 4: display(); break; /* case 5: undo(); break; case 6: redo(); break; */ case 7: cout << system("CLS") << "\n\n\t /////PROGRAM ENDED/////\n\n"; break; default: cout << "Wrong Option. Please re-enter your Option\n"; } } while (menuOption != 5); return 0; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
C++ language. Using the coding prepared below, add and create the Undo last pop, Display undo list and Redo Functions void functions but keep them as they are.
NOTE: use the coding given, i don't want a new coding
The code:
#include<iostream>
#include<iomanip>
#include<ctype.h>
using namespace std;
struct nodeWords
{
char words[100];
struct nodeWords *next;
};
nodeWords* top; //these pointers are used to hold the linked list.
nodeWords* current; //use for traversal
nodeWords* newNode; //use to create new node for new record
nodeWords* pop;
void Push()
{
char answer;
do
{
newNode = new nodeWords;
cout << "\nWrite what's in your mind.." << endl;
cout << "Your words > ";
cin >> ws;
cin.getline(newNode->words, 100);
newNode->next = top;
top = newNode;
cout << "\nWant to write more? Press Y for yes and N for no: ";
cin >> answer;
} while (toupper(answer) == 'Y');
}
void stacktop()
{
if (top == NULL)
{
cout << "\nThere is no words!\n";
}
else
{
cout << "\nTHE TOP ELEMENT IS..... ";
cout << "\nYour words > " << top->words << endl;
}
}
void display()
{
char answer2;
int no = 1;
int totalRecord = 0;
int view;
{
cout << endl << left << setw(5) << "No" << setw(50) << "Your words" << endl;
cout << "----------------------------------------------------------------------" << endl;
current = top;
while (current != NULL)
{
cout << left << setw(5) << no << setw(50) << current->words << endl;
no++;
current = current->next;
totalRecord++;
}
cout << "----------------------------------------------------------------------" << endl;
cout << "\nTotal Number of Records: " << totalRecord << endl;
}
cout << "\nPress Y to go back to MAIN MENU: ";
cin >> answer2;
}
void Pop()
{
current = top;
while (current != NULL)
{
pop = current;
if (current == top)
{
top = top->next;
current = NULL;
delete pop;
cout << "\n\nPop Operation Success!!\n";
}
else{
top = NULL;
current = NULL;
current = NULL;
delete pop;
cout << "\n\nPop Operation Success!!\n";
}
}
}
int main()
{
int menuOption;
top = NULL; //create list
do
{
cout << "--------------------------------------\n";
cout << "\t\tMain Menu\n";
cout << "--------------------------------------\n";
cout << "1 - Push/Add Record \n";
cout << "2 - Peek/Stack Top \n";
cout << "3 - Pop/Remove Record \n";
cout << "4 - Display\n";
cout << "5 - Undo\n";
cout << "6 - Redo\n";
cout << "7 - Exit\n";
cout << "---------------------------------------\n";
cout << "Enter your Option [1, 2, 3, 4, 5, 6, 7]: ";
cin >> menuOption;
switch (menuOption)
{
case 1: Push();
break;
case 2: stacktop();
break;
case 3: current = top;
if (current == NULL)
{
cout << "\n\n The List is Empty!!\n\n";
cout << "Unable to Perform the Delete Operation.\n\n";
}
else {
Pop();
}
break;
case 4: display();
break;
/* case 5: undo();
break;
case 6: redo();
break; */
case 7: cout << system("CLS") << "\n\n\t /////PROGRAM ENDED/////\n\n";
break;
default: cout << "Wrong Option. Please re-enter your Option\n";
}
} while (menuOption != 5);
return 0;
}

Step by step
Solved in 2 steps

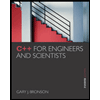
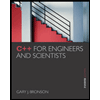