C LANGUAGE Randomly generate 50 integers within the range of 0 – 999. Store the integers in an array.Pass the array to different functions that perform the tasks below: i. Print out the randomly generated integers in 5 rows, each row having 10 integer. Input: array return: none Random integer generation: #include rand(); //generate random integer rand()%999; //generate random integer between 0-999 ii. Show the range of integers generated: Output the smallest and largest integer and their index in the array.
C LANGUAGE
Randomly generate 50 integers within the range of 0 – 999. Store the integers in an array.Pass the array to different functions that perform the tasks below:
i. Print out the randomly generated integers in 5 rows, each row having 10 integer.
Input: array
return: none
Random integer generation:
#include<stdlib.h>
rand(); //generate random integer
rand()%999; //generate random integer between 0-999
ii. Show the range of integers generated: Output the smallest and largest integer and their index in the array.
Input: array
return: none
iii. Sort the integers in ascending order
Input: array
return: none
findsmallestnumber(int start, int end, array)
input: start index of array, end index of array, array
return: smallest number index or position in array
iv. Generate another array of 50 integers. Compare the 2 arrays and count the similarity percentage of the 2 arrays.
Input: Array1, Array2
Return: none
** Use 2 for loops for this function, One to loop Array1 and another one inside to
loop Array2
Sort
sort(array)
{
loop(array start to end)
{
smallestNumberIndex = findsmallestnumber(array, currentindex, end);
if (currentIndex != smallestNumberIndex)
{ //swap places
temp =array[smallestNumberIndex];
array[smallestNumberIndex] = array[currentIndex];
array[currentIndex] = temp;
}
}
}
![Example of sorting strategy:
arr[] = 64 25 12 22 11
// Find the minimum element in arr[0...4]
// and place it at beginning
11 25 12 22 64
// Find the minimum element in arr[1...4]
// and place it at beginning of arr[1...4]
11 12 25 22 64
// Find the minimum element in arr[2...4]
// and place it at beginning of arr[2...4]
11 12 22 25 64
// Find the minimum element in arr[3...4]
// and place it at beginning of arr[3...4]
11 12 22 25 64](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe20081f6-3e71-4df1-8a15-056fbe8295f3%2F358041e5-041d-465c-a175-b5cb1356ca15%2Fdyjglr8_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

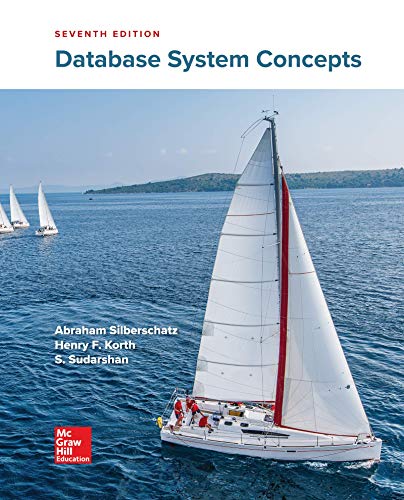
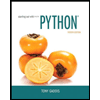
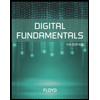
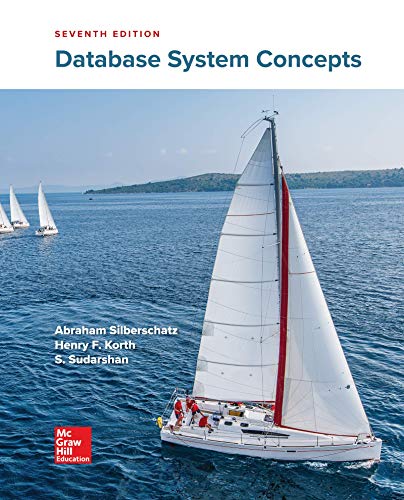
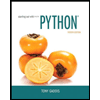
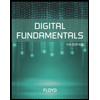
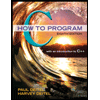
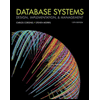
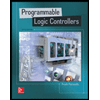