C++ HurdleWords The HurdleWords class is mostly provided to you. HurdleWords is constructed from two files in the data/ folder: ● valid_guesses.txt (all 5 letter guesses considered to be valid words to guess), and ● valid_hurdles.txt (all words that may be selected to be the secret Hurdle.) ● Note: you may edit both text files if you’d like to add custom words to your game. HurdleWords stores all potential valid Hurdles from valid_hurdles.txt into a vector of strings (valid_hurdles_), and all valid guesses from valid_guesses.txt into an unordered set of strings (valid_guesses_). A set is simply a data structure that contains no duplicates and allows for a speedy lookup to check if a given element exists within the set. Because there are over 10,000 valid guesses, we store them in an unordered set to leverage their speediness, as you will need to check if a user-submitted guess is considered valid (i.e. their guess is considered a valid guess in the dictionary). You are responsible for implementing two short functions in the HurdleWords class. You can implement each function in less than 3 lines, so try not to overcomplicate these!
C++
HurdleWords The HurdleWords class is mostly provided to you. HurdleWords is constructed from two files in the data/ folder:
● valid_guesses.txt (all 5 letter guesses considered to be valid words to guess), and
● valid_hurdles.txt (all words that may be selected to be the secret Hurdle.)
● Note: you may edit both text files if you’d like to add custom words to your game.
HurdleWords stores all potential valid Hurdles from valid_hurdles.txt into a
You are responsible for implementing two short functions in the HurdleWords class. You can implement each function in less than 3 lines, so try not to overcomplicate these!
hurdlewords.h file
#include <string>
#include <unordered_set>
#include <vector>
#ifndef HURDLEWORDS_H
#define HURDLEWORDS_H
class HurdleWords {
public:
HurdleWords(const std::string &valid_hurdles_filename,
const std::string &valid_guesses_filename);
// Returns true if the given word is a valid guess
// according to the words in this class.
bool IsGuessValid(const std::string &word) const;
// Returns a random hurdle from the list of valid
// hurdles (secret words) stored in the words in this class.
const std::string &GetRandomHurdle() const;
private:
// valid_hurdles_ is a list of valid words from which the
// secret hurdle may be chosen.
std::vector<std::string> valid_hurdles_;
// valid_guesses_ is a list of valid words that are considered
// acceptable gueses a user can submit. We use a set here because
// checking if a word is in a set is more time efficient than a vector.
std::unordered_set<std::string> valid_guesses_;
};
#endif // HURDLEWORDS_H
hurdlewords.cc file
#include "hurdlewords.h"
#include <cstdlib>
#include <ctime>
#include <fstream>
#include <string>
HurdleWords::HurdleWords(const std::string& valid_hurdles_filename,
const std::string& valid_guesses_filename) {
// Read from the file containing all valid secret
// hurdles, and insert them into the valid_hurdles_ vector.
std::ifstream hurdles_file(valid_hurdles_filename);
std::string word;
while (hurdles_file >> word) {
valid_hurdles_.push_back(word);
}
// Read from the file containing all valid guesses,
// and insert them into the valid_guesses_ set.
std::ifstream guesses_file(valid_guesses_filename);
while (guesses_file >> word) {
valid_guesses_.insert(word);
}
// Use the current time as a seed for the random number generator.
srand(time(nullptr));
}
bool HurdleWords::IsGuessValid(const std::string& word) const {
//=================== YOUR CODE HERE ===================
// TODO: Return true if the given `word` is considered
// a valid guess. If the guess is invalid, return false.
//======================================================
}
const std::string& HurdleWords::GetRandomHurdle() const {
//=================== YOUR CODE HERE ===================
// TODO: Select and return a random Hurdle from the
// list of valid Hurdles stored in the valid_hurdles_
// vector.
// Hint: we suggest using the rand() function to
// generate a random number.
//======================================================
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

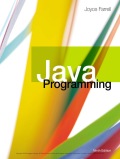
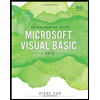
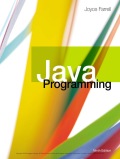
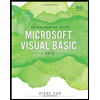
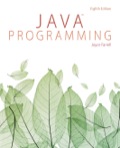
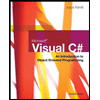