Build a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one private member field, double value, for the calculator's current value. Implement the following Constructor and instance methods as listed below: • public Calculator() - Constructor method to set the member field to 0.0 • public void add(double val) - add the parameter to the member field • public void subtract(double val) - subtract the parameter from the member field • public void multiply(double val) - multiply the member field by the parameter • public void divide(double val) - divide the member field by the parameter • public void clear() - set the member field to 0.0 • public double getValue() - return the member field Given two double input values num1 and num2, the program outputs the following values:
Build a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one private member field, double value, for the calculator's current value. Implement the following Constructor and instance methods as listed below: • public Calculator() - Constructor method to set the member field to 0.0 • public void add(double val) - add the parameter to the member field • public void subtract(double val) - subtract the parameter from the member field • public void multiply(double val) - multiply the member field by the parameter • public void divide(double val) - divide the member field by the parameter • public void clear() - set the member field to 0.0 • public double getValue() - return the member field Given two double input values num1 and num2, the program outputs the following values:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Java - Calculator Class
![Build a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one
private member field, double value, for the calculator's current value. Implement the following Constructor and instance methods as listed
below:
}
• public Calculator() - Constructor method to set the member field to 0.0
• public void add(double val) - add the parameter to the member field
• public void subtract(double val) - subtract the parameter from the member field
• public void multiply(double val) - multiply the member field by the parameter
public void divide (double val) - divide the member field by the parameter
• public void clear() - set the member field to 0.0
• public double getValue() - return the member field
Given two double input values num1 and num2, the program outputs the following values:
1. The initial value of the instance field, value
2. The value after adding num1
3. The value after multiplying by 3
4. The value after subtracting num2
5. The value after dividing by 2
6. The value after calling the clear() method
Ex: If the input is:
10.0 5.0
the output is:
0.0
10.0
30.0
25.0
12.5
0.0
import java.util.Scanner;
public class calculator {
// TODO: Build calculator class with methods and fields Listed above
/* Type your code here. */
public static void main(String[] args) {
calculator calc = new Calculator();
}
Scanner keyboard = new Scanner(System.in);
double num1 keyboard.nextDouble();
double num2 = keyboard.nextDouble();
// 1. The initial value
System.out.println(calc.getvalue());
// 2. The value after adding num1
calc.add(num1);
System.out.println(calc.getvalue());
// 3. The value after multiplying by 3
calc.multiply(3);
System.out.println(calc.getvalue());
// 4. The value after subtracting num2
calc.subtract (num2);
System.out.println(calc.getvalue());
// 5. The value after dividing by 2
calc.divide (2);
system.out.println(calc.getvalue());
// 6. The value after calling the clear() method
calc.clear();
System.out.println(calc.getvalue());](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F73901183-513c-4b44-b5b0-c95e8b35aeb4%2Fefc05cd2-11a9-45c0-a20f-2fd48a7ef29c%2Fvuzuils_processed.png&w=3840&q=75)
Transcribed Image Text:Build a class called Calculator that emulates basic functions of a calculator: add, subtract, multiply, divide, and clear. The class has one
private member field, double value, for the calculator's current value. Implement the following Constructor and instance methods as listed
below:
}
• public Calculator() - Constructor method to set the member field to 0.0
• public void add(double val) - add the parameter to the member field
• public void subtract(double val) - subtract the parameter from the member field
• public void multiply(double val) - multiply the member field by the parameter
public void divide (double val) - divide the member field by the parameter
• public void clear() - set the member field to 0.0
• public double getValue() - return the member field
Given two double input values num1 and num2, the program outputs the following values:
1. The initial value of the instance field, value
2. The value after adding num1
3. The value after multiplying by 3
4. The value after subtracting num2
5. The value after dividing by 2
6. The value after calling the clear() method
Ex: If the input is:
10.0 5.0
the output is:
0.0
10.0
30.0
25.0
12.5
0.0
import java.util.Scanner;
public class calculator {
// TODO: Build calculator class with methods and fields Listed above
/* Type your code here. */
public static void main(String[] args) {
calculator calc = new Calculator();
}
Scanner keyboard = new Scanner(System.in);
double num1 keyboard.nextDouble();
double num2 = keyboard.nextDouble();
// 1. The initial value
System.out.println(calc.getvalue());
// 2. The value after adding num1
calc.add(num1);
System.out.println(calc.getvalue());
// 3. The value after multiplying by 3
calc.multiply(3);
System.out.println(calc.getvalue());
// 4. The value after subtracting num2
calc.subtract (num2);
System.out.println(calc.getvalue());
// 5. The value after dividing by 2
calc.divide (2);
system.out.println(calc.getvalue());
// 6. The value after calling the clear() method
calc.clear();
System.out.println(calc.getvalue());
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
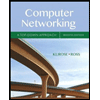
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
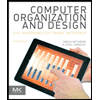
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
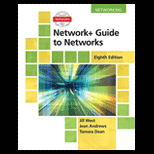
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
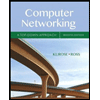
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
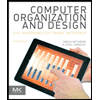
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
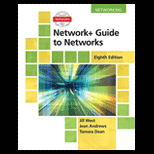
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
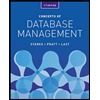
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
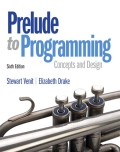
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
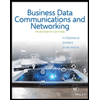
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY