Apply your HeapMax class to solve the following problem: In a research project your teammate who is responsible for data collection retrieves the rankings of a set of experiment results, and she chooses to use integers to represent the success rate of each experiment. The rankings are stored in a n*n matrix where each row and column are sorted in increasing order. Your project manager wants to know the ranking of kth most successful experiment. In other words, your task is to find the kth largest number in the matrix. Please solve the problem to help your teammate. Please note that it is the kth largest element in the sorted order, not the kth distinct element./ Example: matrix = [ [1 5, 9], [10, 11, 13], [12, 13, 15] ], k = 2, return 13. This class is named "HeapMaxApp". The method that solves this problem should be declared static and named as “kthBiggest", which accepts two required parameters:
Apply your HeapMax class to solve the following problem: In a research project your teammate who is responsible for data collection retrieves the rankings of a set of experiment results, and she chooses to use integers to represent the success rate of each experiment. The rankings are stored in a n*n matrix where each row and column are sorted in increasing order. Your project manager wants to know the ranking of kth most successful experiment. In other words, your task is to find the kth largest number in the matrix. Please solve the problem to help your teammate. Please note that it is the kth largest element in the sorted order, not the kth distinct element./ Example: matrix = [ [1 5, 9], [10, 11, 13], [12, 13, 15] ], k = 2, return 13. This class is named "HeapMaxApp". The method that solves this problem should be declared static and named as “kthBiggest", which accepts two required parameters:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please answer the problem in the screenshot. Please use the methods in the second screenshot.
![Apply your HeapMax class to solve the following problem:
In a research project your teammate who is responsible for data collection retrieves the
rankings of a set of experiment results, and she chooses to use integers to represent the
success rate of each experiment. The rankings are stored in a n*n matrix where each
row and column are sorted in increasing order. Your project manager wants to know the
ranking of kth most successful experiment. In other words, your task is to find the kth
largest number in the matrix. Please solve the problem to help your teammate.
Please note that it is the kth largest element in the sorted order, not the kth distinct
element./
Example:
matrix =
[
[1, 5, 9],
[10, 11, 13],
[12, 13, 15]
],
k = 2, return 13.
This class is named "HeapMaxApp". The method that solves this problem should be
declared static and named as “kthBiggest", which accepts two required parameters:
public static int kthBiggest(int[][] matr, int k)
You should also create a main method under this class, in which at least two test cases
need to be given to test kthBiggest method by printing necessary information. For
instance, the printed messages of an example are:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1725b614-c61a-470a-b05f-008b5304bcc7%2F29a680b6-9d85-4d07-a1cc-f1cec42eb0b1%2Fuz8anxf_processed.png&w=3840&q=75)
Transcribed Image Text:Apply your HeapMax class to solve the following problem:
In a research project your teammate who is responsible for data collection retrieves the
rankings of a set of experiment results, and she chooses to use integers to represent the
success rate of each experiment. The rankings are stored in a n*n matrix where each
row and column are sorted in increasing order. Your project manager wants to know the
ranking of kth most successful experiment. In other words, your task is to find the kth
largest number in the matrix. Please solve the problem to help your teammate.
Please note that it is the kth largest element in the sorted order, not the kth distinct
element./
Example:
matrix =
[
[1, 5, 9],
[10, 11, 13],
[12, 13, 15]
],
k = 2, return 13.
This class is named "HeapMaxApp". The method that solves this problem should be
declared static and named as “kthBiggest", which accepts two required parameters:
public static int kthBiggest(int[][] matr, int k)
You should also create a main method under this class, in which at least two test cases
need to be given to test kthBiggest method by printing necessary information. For
instance, the printed messages of an example are:
![Testing of kthBiggest starts.
The given matrix is:
1, 5, 9
10, 11, 13
12, 13, 15
The 4nd biggest element is 12.
Testing of kthBiggest ends.
Note that it is the kth largest element in the sorted order, not the kth distinct element.
Hint: You only need a max heap that always has matr.length elements.
import java.util. *:
public class HeapMaxApp{
public static void main(String[] args) {
// in case if you want to test your solution
}
// return the kth largest element from matr
// you can assume that k is between 1 and matr.length * matr[0].length
public static int kthBiggest(int[]) matr, int k) {
// remove this line
return 0;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1725b614-c61a-470a-b05f-008b5304bcc7%2F29a680b6-9d85-4d07-a1cc-f1cec42eb0b1%2F3cpo8m_processed.png&w=3840&q=75)
Transcribed Image Text:Testing of kthBiggest starts.
The given matrix is:
1, 5, 9
10, 11, 13
12, 13, 15
The 4nd biggest element is 12.
Testing of kthBiggest ends.
Note that it is the kth largest element in the sorted order, not the kth distinct element.
Hint: You only need a max heap that always has matr.length elements.
import java.util. *:
public class HeapMaxApp{
public static void main(String[] args) {
// in case if you want to test your solution
}
// return the kth largest element from matr
// you can assume that k is between 1 and matr.length * matr[0].length
public static int kthBiggest(int[]) matr, int k) {
// remove this line
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
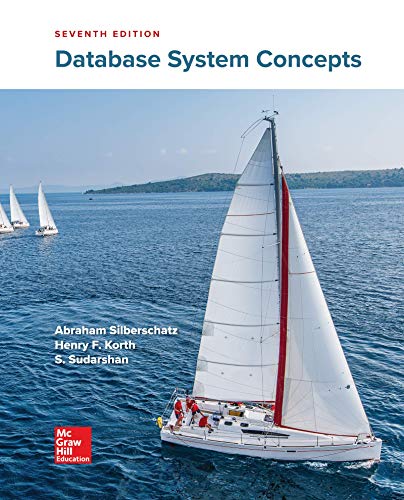
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
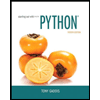
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
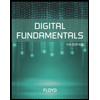
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
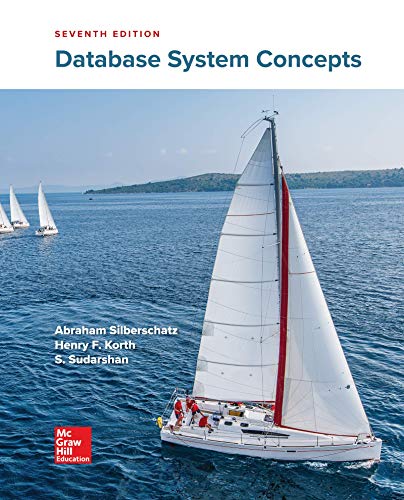
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
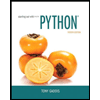
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
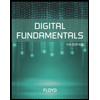
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
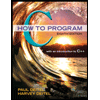
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
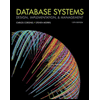
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
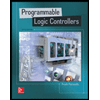
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education