advanced java For this assignment you will write a program to represent geometric shapes and some operations that can be performed on them. The idea here is that shapes in higher dimensions inherit data from lower dimensional shapes. For example a cube is a three dimensional square. A sphere is a three dimensional circle and a glome is a four dimensional circle. A cylinder is another kind of three dimensional circle. The circle, sphere, cylinder, and glome all share the attribute radius. The square and cube share the attribute side length. There are various ways to use inheritance to relate these shapes but please follow the inheritance described in the table below. All shapes inherit getName() from the superclass Shape. Specification: The program should have the following classes: Shape, Circle, Square, Cube, Sphere, Cylinder, and Glome and two interfaces Area and Volume (Area.java and Volume.java are given below). The volume of a glome is 0.5(π2)r4 where r is the radius. Your classes may only have the class variable specified in the table below and the methods defined in the two interfaces Area and Volume. You will implement the methods specified in the Area and Volume interfaces and have them return the appropriate value for each shape. Class Shape will have a single public method called getName that returns a string. Class Class Variable Constructor Extends Implements Shape String name Shape() Circle double radius Circle( double r, String n ) Shape Area Square double side Square( double s, String n ) Shape Area Cylinder double height Cylinder(double h, double r, String n ) Circle Volume Sphere None Sphere( double r, String n ) Circle Volume Cube None Cube( double s, String n ) Square Volume Glome None Glome( double r, String n ) Sphere Volume Your program will use the following: (please separate both area and volume. Put first part and second part Area.java /* * The classes Square and Circle must implement this interface. */ public interface Area { /** * @return the area of a shape. The type is double so there won't be type casting issues with the Java Math library. */ double getArea(); Volume.java /* * The classes Cube, Sphere, Cylinder, and Glome must implement this interface. */ public interface Volume { /** * @return the volume of a shape. The type is double so there won't be type casting issues with the Java Math library. */ double getVolume();
advanced java
For this assignment you will write a
All shapes inherit getName() from the superclass Shape.
Specification:
The program should have the following classes: Shape, Circle, Square, Cube, Sphere, Cylinder, and Glome and two interfaces Area and Volume (Area.java and Volume.java are given below).
The volume of a glome is 0.5(π2)r4 where r is the radius.
Your classes may only have the class variable specified in the table below and the methods defined in the two interfaces Area and Volume. You will implement the methods specified in the Area and Volume interfaces and have them return the appropriate value for each shape. Class Shape will have a single public method called getName that returns a string.
Class |
Class Variable |
Constructor |
Extends |
Implements |
Shape |
String name |
Shape() |
||
Circle |
double radius |
Circle( double r, String n ) |
Shape |
Area |
Square |
double side |
Square( double s, String n ) |
Shape |
Area |
Cylinder |
double height |
Cylinder(double h, double r, String n ) |
Circle |
Volume |
Sphere |
None |
Sphere( double r, String n ) |
Circle |
Volume |
Cube |
None |
Cube( double s, String n ) |
Square |
Volume |
Glome |
None |
Glome( double r, String n ) |
Sphere |
Volume |
Your program will use the following: (please separate both area and volume. Put first part and second part
- Area.java
/*
* The classes Square and Circle must implement this interface.
*/
public interface Area
{
/**
* @return the area of a shape. The type is double so there won't be type casting issues with the Java Math library.
*/
double getArea();
- Volume.java
/*
* The classes Cube, Sphere, Cylinder, and Glome must implement this interface.
*/
public interface Volume
{
/**
* @return the volume of a shape. The type is double so there won't be type casting issues with the Java Math library.
*/
double getVolume();
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

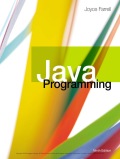
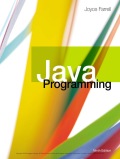