4. What is the output of the following program: void fun (int n) { cout << n; if (n = 3) return; fun (n+1); cout << n; a. 12 b. 132 c. 12321 d. 1234 e. 12345 int main () { } fun (1); return 0;
4. What is the output of the following program: void fun (int n) { cout << n; if (n = 3) return; fun (n+1); cout << n; a. 12 b. 132 c. 12321 d. 1234 e. 12345 int main () { } fun (1); return 0;
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.4: A Case Study: Rectangular To Polar Coordinate Conversion
Problem 9E: (Numerical) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question

Transcribed Image Text:4. What is the output of the following program:
void fun (int n) {
}
cout << n;
if (n = 3)
return;
fun (n+1);
cout << n;
a. 12
b. 132
c. 12321
d. 1234
vector <int> v2 = {3};
v2 = v1;
e. 12345
5. Consider the following code segment, and select the best answer:
vector <int> vl =
(11, 23, 99};
for (int i = 0; i < 3; i++)
cout << v2.at (i) << " ";
The output will be:
a. 11 23 99
b. 3 23 99
int main()
{
}
a. This code gives a compilation error on the second line.
b. This code prints 6.
c. This code prints -6.
d. None of the above
fun (1);
return 0;
c. 3
d. There will be no output due to improper assignment operation in the
third line.
6. Consider the following code segment. Which one of the following statements is true?
string s1 = "893";
s1 += "99";
int y = s1.at (2) sl.at (4);
cout << y;
![15. In a C or C++ program, header files normally do NOT contain:
a. include directives
b. constants
C. struct definitions
d. function prototypes
e. function definition/implementation
16. Consider the following code segment that dynamically allocates memory on the heap to create and use it as a
matrix with 4 rows, and 3 columns:
int **a = new int* [4];
for (int i = 0; i <4; i++) {
}
Which one of the following statements is the proper way to de-allocate/remove the allocated memory from heap:
for (int i= 0; i < 3; i++)
delete [ a[i];
a
b
с
a[i] = new int [3];
for (int j=0; j < 3; j++)
a[i] [j] = 0;
delete [] a;
delete [] a;
for (int i = 0; i < 4; i++)
delete [] a [i];
for (int i = 0; i < 4; i++)
delete [] a[i];
delete [] a;
d delete [] a;
};
Consider the following definition of class Box and main function. Assuming constructor, copy constructor,
assignment operator, and destructor of this class are properly without any error implemented, answer the
following four questions:
class Box {
public:
Box();
~Box ();
Box (const Box & source);
Box& operator= (const Box & rhs);
private:
int* pointer;
int main (void) {
Box x;
Box y (x);
Box *z = new Box;
x = y;
return 0;
17. How many times the constructor of class Box is called?
Once
a.
b. Twice
c. Three times
d. None of the above
18. How many times the copy-constructor of class Box is called?
a. Once
b. Twice
c. Three times
d. None of the above
20. How many times destructor of class Box is called
}
a. Once
b. Twice
c. Three times
d. None of the above
19. How many times assignment operator of class Box is called
a. Once
b. Twice
c. Three times
d. None of the above
m reaches point 1, for the second](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F94884f69-365e-43dc-a457-f3f8066ccdfc%2F252eed10-416b-4cab-b7c5-c160681751ba%2Fn2bvej5_processed.png&w=3840&q=75)
Transcribed Image Text:15. In a C or C++ program, header files normally do NOT contain:
a. include directives
b. constants
C. struct definitions
d. function prototypes
e. function definition/implementation
16. Consider the following code segment that dynamically allocates memory on the heap to create and use it as a
matrix with 4 rows, and 3 columns:
int **a = new int* [4];
for (int i = 0; i <4; i++) {
}
Which one of the following statements is the proper way to de-allocate/remove the allocated memory from heap:
for (int i= 0; i < 3; i++)
delete [ a[i];
a
b
с
a[i] = new int [3];
for (int j=0; j < 3; j++)
a[i] [j] = 0;
delete [] a;
delete [] a;
for (int i = 0; i < 4; i++)
delete [] a [i];
for (int i = 0; i < 4; i++)
delete [] a[i];
delete [] a;
d delete [] a;
};
Consider the following definition of class Box and main function. Assuming constructor, copy constructor,
assignment operator, and destructor of this class are properly without any error implemented, answer the
following four questions:
class Box {
public:
Box();
~Box ();
Box (const Box & source);
Box& operator= (const Box & rhs);
private:
int* pointer;
int main (void) {
Box x;
Box y (x);
Box *z = new Box;
x = y;
return 0;
17. How many times the constructor of class Box is called?
Once
a.
b. Twice
c. Three times
d. None of the above
18. How many times the copy-constructor of class Box is called?
a. Once
b. Twice
c. Three times
d. None of the above
20. How many times destructor of class Box is called
}
a. Once
b. Twice
c. Three times
d. None of the above
19. How many times assignment operator of class Box is called
a. Once
b. Twice
c. Three times
d. None of the above
m reaches point 1, for the second
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
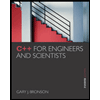
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
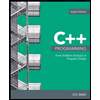
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
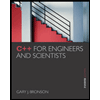
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
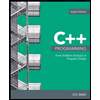
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning