3. 24-hour time (also known in the U.S. as military time) is widely used around the world. Time is expressed as hours since midnight. The day starts at 0000, and ends at 2359. • Write a program that converts am/pm time to 24-hour time. • Hints: o Think of how each hour should be handled. 12:00 am -12:59 am becomes what? 8:00 am becomes what? 12:00 pm? 1:00 pm? Group the hours into cases that should be handled similarly (e.g., 1:00 am to 11:00 am are handled the same). o Declare variables for hoursAmPm, minAmPm, and hours24. Note that minutes for 24-hour time remain the same as for am/pm, so no extra variable is needed. o Use an if-else statement to detect each case, and set the hours24 appropriately. o When outputting hour24, check if the hour is 0-9 (just check for < 10). If so, output a "0". So 7 will be output as 07. Do the same when outputting the minutes. 4. Make sure to follow the Grading Rubric and Academic Integrity guidelines outlined in the syllabus. Expected Program Output: Test Run #1 2:30 pm 1430 Test Run #2 12:01 am 0001
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
C++ please - 24-hour time (also known in the U.S. as military time) is widely used around the world. Time is expressed as hours since midnight. The day starts at 0000, and ends at 2359. Write a program that converts am/pm time to 24-hour time. If the input is 2:30 pm, the output should be 1430. If the input is 12:01 am, the output should be 0001.
Hints:
-
Think of how each hour should be handled. 12:00 am -12:59 am becomes what? 8:00 am becomes what? 12:00 pm? 1:00 pm? Group the hours into cases that should be handled similarly (e.g., 1:00 am to 11:00 am are handled the same).
-
Declare variables for hoursAmPm, minAmPm, and hours24. Note that minutes for 24-hour time remain the same as for am/pm, so no extra variable is needed.
-
Use an if-else statement to detect each case, and set the hours24 appropriately.
-
When outputting hour24, check if the hour is 0-9 (just check for < 10). If so, output a "0". So 7 will be output as 07. Do the same when outputting the minutes.
#include <iostream>
int main()
{
std::string time;
std::cout << "Enter the time as (hh:mm xm) ";
std::getline(std::cin, time);
/* Type your code here. */
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

This doesn't solve the problem nor does it implement the below.
int main()
{
std::string time;
std::cout << "Enter the time as (hh:mm xm) ";
std::getline(std::cin, time);
/* Type your code here. */
return 0;
}
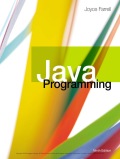
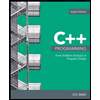
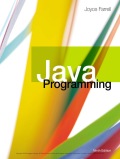
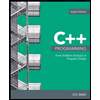