1. Format your code as provided in the Lab 2 Start Code provided below. 2. The user prompt for a size will be: "Please input your stair case size:" 3. Perform a function call within the exception handling logic (Your function that contains the code to run the program should be within a try/except block - see comments in the starter code). 4. Raise and Handle the following exceptions: A custom exception called "IntegerOutOfRangeException" when the user provides an integer value that is not within the valid integer range. ("That staircase size is out of range.") - A custom exception called "NoStairCaseException" when the user provides a staircase size of 0. ("There are no steps in the staircase.") - In ValueError for user input that are not integers. ("There are no steps in the staircase.") 5. The error messages for the above exceptions should be the same as above and printed to the shell. 6. The program should continue to run and request a new input until the user types the word "DONE" 7. Use this start code to being your project. You can use your code from lab1. (Only your code not anyone else's).
Python.
1. Format your code as provided in the Lab 2 Start Code provided below.
2. The user prompt for a size will be: "Please input your staircase size:"
3. Perform a function call within the exception handling logic (Your function that contains the code to run the program should be within a try/except block - see comments in the starter code).
4. Raise and Handle the following exceptions:
- A custom exception called "IntegerOutOfRangeException" when the user provides an integer value that is not within the valid integer range. ("That staircase size is out of range.")
- A custom exception called "NoStairCaseException" when the user provides a staircase size of 0. ("There are no steps in the staircase.")
- In ValueError for user input that are not integers. ("There are no steps in the staircase.")
5. The error messages for the above exceptions should be the same as above and printed to the shell.
6. The program should continue to run and request a new input until the user types the word "DONE"



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

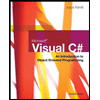
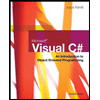