CSE114FinalPracticeSpring2024Solutions
.pdf
keyboard_arrow_up
School
Stony Brook University *
*We aren’t endorsed by this school
Course
114
Subject
Computer Science
Date
May 7, 2024
Type
Pages
14
Uploaded by SargentMongoose3260 on coursehero.com
1 CSE 114 Final Exam Spring 2024: Practice Solutions Question Value Score 1 2 2 2 3 2 4 2 5 2 6 2 7 2 8 2 9 2 10 2 11 4 12 4 13 4 14 4 15 4 16 4 17 4 18 4 19 4 20 4 21 10 22 10 23 10 24 10 Total 100
2 1.
(2 points) Java class inheritance supports multiple inheritance.
TRUE
FALSE 2.
(2 points) ArrayList provides the constant time access and update like an array data structure
TRUE
FALSE 3.
(2 points) Constructors of the parent class are inherited in the child class
TRUE
FALSE 4.
(2 points) An abstract class can have a non-abstract method
TRUE
FALSE 5.
(2 points) An abstract class can be used as a reference type in polymorphism.
TRUE
FALSE 6.
ArithmeticException is an example of a checked exception.
TRUE
FALSE 7.
(2 points) IOException is an example of a checked exception.
TRUE
FALSE 8.
(2 points) An interface can be used as a reference type in polymorphism.
TRUE
FALSE 9.
(2 points) When a class overrides a method, what object reference is used to call the method inherited from the super class?
inherited
super
class
methodName 10.
(2 points) An interface can have a non-abstract method.
TRUE
FALSE
3 11.
(4 points) Explain why the following program displays [1, 3] rather than [2, 3] import
java.util.ArrayList; public
class
Problem { public
static
void
main(String[] args
) { ArrayList<Integer> list = new
ArrayList<>(); list
.add(1); list
.add(2); list
.add(3); list
.remove(1); System.
out
.println(
list
); } } How do you remove integer 3 from the list, when the list has elements 1, 2 and 3 in this order (without using remove with index)? Solution:
because list.remove(1) deletes the second element of the list which is 2. We can remove integer 3 from the list by using the statement list.remove(new Integer(3)); 12.
(4 points) The for loop in the following program is converted into the while loop below. What is wrong? Correct it. public
class
Test { public
static
void
main(String[] args
) { int
sum = 0; for
(
int
i = 0; i < 4; i
++) { if
(
i % 3 == 0) continue
; sum += i
; } System.
out
.println(
sum
); } } public
class
Test { public
static
void
main(String[] args
) { int
sum = 0, i = 0; while
(
i < 4) { if
(
i % 3 == 0) continue
; sum += i
; i
++; }
4 System.
out
.println(
sum
); } Solution:
infinite loop. To fix the problem increment i inside the if- condition. public
class
Test { public
static
void
main(String[] args
) { int
sum = 0, i = 0; while
(
i < 4) { if
(
i % 3 == 0) { i
++; continue
; } sum += i
; i
++; } System.
out
.println(
sum
); } } 13.
(4 points) What is the difference between the method overloading and method overriding in Java? Solution:
Method overloading
: It happens when two methods in the class have same name but different signature. Method overriding
: It happens in inheritance when the non-static method in the child class has the same method signature as the corresponding non-static method in the parent class. Here the non-static method in the child class is said to override the corresponding method in the parent class. 14.
(4 points) You coded the following in the Test.java class in order to output the smallest element in the array a:
public
class
Test { public
static
void
main(String[] args
) { int
[][] a = {{9, 8, 7, 6},{10, 20, 30, 40}}; int
min = a
[0][0]; for
(
int
i = 1; i < a
.
length
; i
++) {
5 for
(
int
j = 0; j < a
[
i
].
length
; j
++) { if
(
a
[
i
][
j
] < min
) min = a
[
i
][
j
]; } } System.
out
.println(
"The minimum is:-->" + min
); } } The program compiles properly, but when you run, you get the following output: The minimum is 9. You expect the value of min to be 6. Explain what the problem is and how to fix it. Solution: The outer for loop should start from i = 0; currently we are not considering the first row except the first element which is 9.
15.
Complete the following program. public
class
Quiz { public
static
void
main(String[] args
) { B b = new
B(); b
.m1(); } } interface
A{ public
void
m1(); } // complete the following code, where B implements interface A class
B ……………………………………………………
{ } Solution:
public
class
Quiz { public
static
void
main(String[] args) { B b = new
B(); b.m1(); } } interface
A{ public
void
m1(); }
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Software Requirements:
• Latest version of NetBeans IDE
• Java Development Kit (JDK) 8
Procedure:
1. our progras from
rhFactor non-static and private. Remove the constructor with two (2) parameters.
2o upply uncapsutation Make bloodType and
2. The names of the public setter and getter methods should be:
• setBloodType()
• setRhFactor()
getBlood Type()
getRhFactor()
3. Use the setter methods to accept user input.
4. Display the values by calling the getter methods.
Sample Output:
Enter blood type of patient:
Enter the Rhesus factor (+ or -):
O+ is added to the blood bank.
Enter blood type of patient: B
Enter the Rhesus factor (+ or -):
B- is added to the blood bank.
arrow_forward
OOP (Object-Oriented Programming) in Java
Applying the composite pattern, you may create a replica of any environment you choose. Any number of media may be used, from photographs to simulators to movies to video games, and so on.
arrow_forward
PROGRAMMING LANGUAGE: JAVA
SUBJECT: ADVANCED OOP WITH JAVA
QUESTION NO 1:
Make an abstract class named A1 with 2 abstract methods, then implement those methods in child class.
arrow_forward
Instructions:
modify the code below and add these features to this dice game:
Multilevel Inheritance with polymorphism and includes at least one abstract class.
Dynamic memory (new, delete) using pointers within a class.
At least one template class.
At least one operator overloading
Add comments
Program:
File GamePurse.h:
class GamePurse
{
// data
int purseAmount;
public:
// public functions
GamePurse(int);
void Win(int);
void Loose(int);
int GetAmount();
};
File GamePurse.cpp:
#include "GamePurse.h"
// constructor initilaizes the purseAmount variable
GamePurse::GamePurse(int amount){
purseAmount = amount;
}
// function definations
// add a winning amount to the purseAmount
void GamePurse:: Win(int amount){
purseAmount+= amount;
}
// deduct an amount from the purseAmount.
void GamePurse:: Loose(int amount){
purseAmount-= amount;
}
// return the value of purseAmount.
int GamePurse::GetAmount(){
return purseAmount;
}
File main.cpp:
// include necessary…
arrow_forward
Classes & Objects Part I DB In Java
What is the purpose of Constructor Overloading? How is this related to method overloading? Provide a unique coded example of constructor overloading.
arrow_forward
JAVA Language
Caesar Shift
Question:
Modify the Caesar class so that it will allow various sized shifts to be used, instead of just a shift of size 3. (Hint: Use an instance variable in the Caesar class to represent the shift, add a constructor to set it, and change the encode method to use it.)
import java.util.*;
public class TestCipher { public static void main(String[] args) { int shift = 7; Caesar caesar = new Caesar(); String text = "hello world"; String encryptTxt = caesar.encrypt(text); System.out.println(text + " encrypted with shift " + shift + " is " + encryptTxt); } }
abstract class Cipher { public String encrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into its words while (words.hasMoreTokens()) { // For each word in s…
arrow_forward
Association Relationships in Java
Using the revised UML Class diagram Resto Fun Final i posted, continuedeveloping the Resto Fun system by modifying class definitions
Create an Order class to break the Many-To-Many relationship between Customer and Item. Thisis a Relationship Class, a class that contains information on the association between to classes.Implement the relationship WaitsOn between Waiter and Customer, knowing that therelationship indicates that this is a One-to-Many relationship mandatory in both ends, i.e., awaiter must wait on a customer and a customer must be waited on by a waiter.Implement the relationship Orders between Customer (Table) and Item, another One-to-Many,since there are many Items a Customers can order, but each Item ordered is for one customeronly.Implement the Add relationship between Order and Item. Include a method to assign the Orderto a Customer before you start adding Items to the Order!
There is enough information now to implement the…
arrow_forward
Java
All classes must be named as shown in the above UML diagram except the concrete child classes. The
ItemList is a singly-linked list with the following methods:
• insert(index: int, item: Item) : boolean
o Inserts the given item at the given index if an equal item is not already in the list. If the
method is successful it returns true. If an equal item already exists in the list or the index
is out of range, this method returns false.
• add(item: Item): boolean
o Adds the given item to the front of the list. Returns true if successful and false if an
equal item is already in the list.
• set(index: int, item: Item) : Item
o Replaces the object in the list at the given index with this object. If the index is out of
range or the given item is already in the list, the method returns null. If the operation is
successful, the method returns the object that was replaced by this one.
• delete(index: int) : Item
o Deletes the object at the given index from the list. If the index is out of…
arrow_forward
SECTION C(JAVA)Look at the class definitions below and answer the questions based on it:class Node{// attributesint ID;String name; Node left; // left child Node right; // right child}class BinarySearchTree{// attributesNode root;// methodssearch(int key, Node root);void insert(int key, Node root);void delete(int key, Node root);}Write code for a method split(int key, Node root) that divides a binary searchtree into two parts. The split should occur at key and create two new binary searchtrees. Return the root of each resulting tree.Test your code with the input in the worked exampleBELOW IS THE INPUT:// id, name41, notes11, personal61, work30, shopping5, recipes55, proposal70, thesis10, muffins43, draft
arrow_forward
Mixed Fraction Arithmetic Java Project:
Problem Description:
Make a java program that facilitates addition, subtraction, multiplication, and division of mixed numbers (similar with the first activity) but this time all of the inputs /outputs may involve fractions, mixed fractions, or a combination.
Points to consider:
MixedFraction class must override the operations (add, subtract, multiplyBy, divideBy) inherited from Fraction class to have a different implementation if the MixedFraction object will have a Fraction object as parameter in performing the required operation, On top of that, the inherited operations must also be overloaded to allow a MixedFraction object to accept other parameters such as a whole number or another MixedFraction object.
The toString method which is inherited must also be overridden by MixedFraction to have a more appropriate return value. Further formatting must also be considered such that if the result of the operation has a zero value for the whole…
arrow_forward
Computer Science
Multiple inheritances (if supported by a language) occurs when a single class inherits properties from 2 or more classes. Multiple inheritance is NOT supported in Java. One can enable multiple inheritances in Java through the use of interfaces. Show how you can enable multiple inheritance in Java and give one or more simple examples.
arrow_forward
language: Java
Class Inheritance with an abstract class
Define a class Employee with the following fields: _name, _empId, _department, _position (_title), _yearlySalary, _fullTime (a boolean: true/false) , _month , _year (time of hire)
Add constructor and properties
Define an abstract method GetBiMonthlySalary() (if the employee is paid twice a month)
Define an abstract method GetVacationTime() that returns the number of vacation time this employee would have based on time of hire
Override the ToString() method to return a string that contains the information pertaining to an employee such as name, empId, department, and where full time or not.
Define a class HourlyEmployee that inherits from Employee.
This class should have 2 fields of its own: _hours (hours worked in half a month) and _wage (hourly wage)
Provide necessary constructor, properties and methods
Override the ToString() to also return the additional fields
Gets 3 days of vacation after first year if the…
arrow_forward
1- Based on the UML shown below, Implement in Java the following inheritance hierarchy (the
classes' description comes next):
Disk
- title: String
- playTime: int // in minutes
+ Disk(title: String, playTime: int)
+ getTitle(): String
+ getPlay Time(): int
+ toString(): String
Audio
Video
- artist: String
- director: String
· tracksList: java.util.ArrayList
- description: String
+ Audio(artist: String, title: String,
+ Video(director: String, description:
play Time: int)
String, title: String, playTime: int)
+ getArtitst(): String
+ getDirector(): String
+ getTracksList()
+ getDescription(): String
+ addTrack(name: String): void
+ toString(): String
+ removeTrack(name: String): void
+ toString(): String
A. Disk class is characterized by a String attributes title and an integer playTime. This class has:
• A constructor that initializes all the attributes.
•
The getter and setter methods for the attributes.
•
A to String() method that returns each data field at a line in the form:…
arrow_forward
Q# In the company example, a supervisor has a supervisee. This “has-a” relationship is called:
Group of answer choices
1. parent
2. composition
3. extending
3. inheritance
arrow_forward
Javascript
Part 1
Design a Monster class. A Monster is created with a language argument string and a stomach represented by an array. The Monster should support functions eat() and speak(). Use the class notation to define your Monster object's interface.
eat(food) will store the passed argument into its stomach and return what it has eaten thus far.
speak(sentence) will simply return the passed in phrase.
class Monster(language) {constructor(language){// set language}// takes a food_item STRING and returns everything eaten so far ARRAYeat(food_item) {// ... complete }// takes in a sentence STRING and returns the passed in sentence STRING with no changespeak(sentence) {// ... complete}}
Part 2
Create an object, Gremlin, that extends the Monster interface and takes the same argument language.
Gremlins inherits how a monster eats.
Gremlins speaks differently. Gremlins replace each word in a sentence with its only known language, "gar". For example, if the sentence is "I like chicken",…
arrow_forward
Javascript
Step 1
Design a Monster class. A Monster is created with a language argument string and a stomach represented by an array. The Monster should support functions eat() and speak(). Use the class notation to define your Monster object's interface.
eat(food) will store the passed argument into its stomach and return what it has eaten thus far.
speak(sentence) will simply return the passed in phrase.
class Monster(language) {constructor(language){// set language}// takes a food_item STRING and returns everything eaten so far ARRAYeat(food_item) {// ... complete }// takes in a sentence STRING and returns the passed in sentence STRING with no changespeak(sentence) {// ... complete}}
Step 2
Create an object, Gremlin, that extends the Monster interface and takes the same argument language.
Gremlins inherits how a monster eats.
Gremlins speaks differently. Gremlins replace each word in a sentence with its only known language, "gar". For example, if the sentence is "I like chicken",…
arrow_forward
Data class encapsulate an integer array x
class dataA and class dataB is-A Data
write an inheritance code in C# to create a constructor for these 3 classes with array x acquired from dependency injection
arrow_forward
Create an object-oriented information system for a RESTAURANT.
The program requires all OOP concepts with different class
1. Inheritance
2. Polymorphism
3. Encapsulation
4. Abstraction- (includes implementatio of inheritance)
Program must:
Read and Display, and Write operations (has transactions(shows reservation, bill and change), display data)
Static only (previous transaction not saved, one time run only)
Terminal only(no GU1, no Database)
arrow_forward
Subject: Object Oriented PrgrammingLanguage: Java Topic: Exception
(SEE ATTACHED PHOTO FOR THE PROBLEM)
Initial code to be completed:public class Matrix{ private int matrix[][]; public Matrix(){} public Matrix(int row, int column){ matrix = new int[row][column]; } //your methods here}
arrow_forward
Create an object-oriented information system for a RESTAURANT.
Requires all OOP concepts:
With different class
1. Inheritance
2. Polymorphism
3. Encapsulation
4. Abstraction - (includes implementatio of inheritance)
Program must:
Read and Display, and Write operations (has transactions(shows bill and change), display data)
Static only (previous transaction not
saved, one time run only)
-Terminal only(no GUI, no Database)
arrow_forward
SV
What is polymorphism.
What is packed array
arrow_forward
Computer Science
There are clearly some similarities in the implementations of Taxi and Shuttle that suggest use of inheritance to represent them. Introducing inheritance is the primary task of this assessment.
-The Ezcab class, The Ezcab class maintains separate lists of taxis and shuttles and destinations with destination fares (you can hardcode destination fares). It has a lookup method that searches for a taxi/shuttle with a given ID.
-The Taxi and Shuttle classes share some common attributes – location and destination. They also have some common methods – getLocation, getDestination, getStatus and setDestination.
-Vehicle is the superclass of both Taxi and Shuttle. This class involves placing the common fields and methods into Vehicle and removing them from Taxi and Shuttle.
-Modify Taxi and Shuttle class to indicate that it is a subclass of Vehicle. You can keep the id field in Vehicle class and getId,SetId methods.
-Arrange for Taxi's constructor to call the constructor of…
arrow_forward
Java Programming Problem 2 – Message Encoder, Decoder
Create an interface MessageEncoder that has a single abstract method encode(plainText), where plainText is the message to be encoded. The method will return the encoded message.
Create a class SubstitutionCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called shift. Define the method encode so that each letter is shifted by the value in shift. Defne the method encode so that each letter is shifted by the value in shift. For example, if shift is 3, a will be replaced by d, b will be replaced by e, c will be replaced by f, and so on. (Hint: You may wish to define a private method that shifts a single character.)
Create a class ShuffleCipher that implements the interface MessageEncoder, as described above. The constructor should have one parameter called n. Define the method encode so that the message is shuffled n times. To perform one shuffle, split the message…
arrow_forward
Create an object-oriented information system for a RESTAURANT.
Requires all OOP concepts:
1. Inheritance
2. Polymorphism
3. Encapsulation
4. Abstraction - (includes implementation of inheritance)
Program must:
- Read and Display, and Write operations (has transactions, display data)
- Static only (previous transaction not saved, one time run only)
- Terminal only(no GUI, no Database)
arrow_forward
1
2
3
4
5 package polynomials
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
// Scala 3 (DO NOT EDIT OR REMOVE THIS LINE!!!)
/*
* Your tasks (indicated with 'Task X' below):
*
* Task 1:
* Implement mutable internal state (=current value) for Variables.
Complete the 'set' and 'value' methods in class Variable,
* and the 'value' method in class Constant.
* Task 2:
* Implement polynomial value computation using structural recursion.
* Complete the 'value methods in all the classes extending Polynomial.
* Hint: you may want to take a look at how the method 'asString'
* is implemented.
* Task 3:
* Implement programmer-defined operators to ease working with Polynomial
* objects. That is, complete the methods '*' and '+' in class Polynomial
* so that you may use the operators '*' and '+' to construct Product and
* Sum objects from Polynomial objects. For more on operators in Scala,
see here
*
* http://docs.scala-lang.org/tutorials/tour/operators.html
*/
/**…
arrow_forward
Computer Science
Exercise 1 (java)– Horses .Create a class Horse containing the attributes name, color and year of birth. Implement getters and setters for the member variables Implement a constructor Create the classes (a) Racehorse and (b) Workhorse. In the case (a), you must add field a field holding the number of races won. In the case (b), add a field that contains the number of years the horse has been working. Write the appropriate getters and setters. Test your classes
arrow_forward
Intermediate Java
The Class class has six methods that yield a string representation of the type represented by the Class object. How do they differ when applied to arrays, generic types, inner classes, and primitive types?
arrow_forward
1- Based on the UML shown below, Implement in Java the following inheritance hierarchy (the
classes' description comes next):
Disk
title: String
playTime: int // in minutes
+ Disk(title: String, playTime: int)
+ getTitle(): String
+ getPlayTime(): int
+ toString(): String
Audio
Video
-artist: String
director: String
- tracksList: java.util.ArrayList
- description: String
+ Video(director: String, description:
+ Audio(artist: String, title: String,
playTime: int)
String, title: String, playTime: int)
+ getArtitst(): String
+ getDirector(): String
+ getTracksList()
+ getDescription(): String
+ addTrack(name: String): void
+ toString(): String
+ removeTrack(name: String): void
toString(): String
C. Video class inherits the class Disk. It has as attributes two Strings director and description. This
class has:
A constructor that initializes all attributes.
A toString() method that overrides the toString() method in the Disk class by adding the data
fields of the Video class in the same…
arrow_forward
Map each of the keywords on the left to the statement that describes it the best.
Inheritance
Class
Object
Polymophism
Instance variable
[Choose ]
✓ It is any variable that does not represent a living being.
It allows properties and functions to be passed to another object when an object is deleted.
It represents a blue print of an object.
It is like a global variable that can be accessed in all functions of a program.
It is a category to organize variables and objects in groups.
It is an instance of a class.
It is a programming command with a special meaning.
Classes can acquire properties and functions from other classes.
It is a property inside that class that exists throughout the lifetime of an object.
It is used to define the relationship between classes.
It is a variable of a primitive type.
It is a variable that can store values of multiple types.
It allows a variable of a super class type to be assigned an instance of a subclass type.
It is used to describe that a class…
arrow_forward
Part 2. Library Class
Implement a class, Library, as described in the class diagram below.
Library must implement the Comparable interface.
The compareTo() method must compare the branch names and only the branch names. The comparison must be case insensitive.
The equals() method must compare the branch names and only the branch names. The comparison must be case insensitive.
Be sure to test the equals() and compareTo() methods before proceeding.
Library
- state: String
- branch: String
- city: String
- zip: String
- county: String
- int squareFeet: int
- int hoursOpen: int
- int weeksOpen: int
+ Library(state: String, branch: String, city: String, zip: String, county: String, squareFeet: int, hoursOpen: int, weeksOpen: int)
+ getState(): String
+ getBranch(): String
+ getCity(): String
+ getZip(): String
+ getCounty(): String
+ getSquareFeet(): int
+ getHoursOpen(): int
+ getWeeksOpen(): int
+ setState(state: String): void
+ setBranch(branch: String):void
+…
arrow_forward
Oriented Programming
purses / ITSE203 / Chapter 4 : Inheritance [Week 61 Qu
Consider the given code and match the following:
class Level {
int total;
class Diploma{
void display(){
System.out.print(total)
}
}
Inner class name
Diploma
Outer class name
Level
Name of the method
display
mod/quiz/attempt.php?attempt=434502&cmid%3D50477&page=D4#
4>
arrow_forward
Write a c++ oop code following these instructions only.
Class Scene:
capture_date : int
draw() : void // Abstract method
Class Landscape:
area : double
render(): void
Class FlightSimulator:
It has aggregation relationship with abstract class
Redefine draw() function that has only a print statement of “Drawing Scene”.
Class MountainScene:
Call render function of Landscape as mentioned in diagram.
(a) Add a function getArea() in 'Scene' class.
Class Scene:
capture_date : int
draw() : void // Abstract method
getArea() : string // Displays 'Area of Scene'
Class CityScene:
draw() : void
getArea() : string // Displays 'Area of City Scene'
Class MountainScene:
draw() : void
getArea() : string // Displays 'Area of Mountain Scene'
(b) 1- Create a pointer of Scene class
2- Assign object of MountainScene class to the pointer created in part 1
3- Call getArea() through the pointer
4- Report the output you get in part 3
arrow_forward
* CENGAGE MINDTAP
Q Search this course
• Jar
Programming Exercise 1-8
Instructions
TableAndChairs.java
+
>- Terminal
1 class TableAndChairs {
sandbox $ |
public static void main(String[] args) {
// Write your code here
}
2
Write, compile, and test the TableAndChairs class that displays the
pattern shown in Figure 1-25.
4
5 }
XXXXXXXXXX
X
XXXXX X
X
XXXXX
X X
X
X
X
X
X
X
Figure 1-25 Output of TableAndChairs
program
Open image in a new tab
The exact pattern is displayed below:
X
X
X
X
XXXXXXXXXХ
X
XXXXX
X
XXXXX
X
X
X X
X
X
X
X X
Grading
Write your Java code in the area on the right. Use the Run button to compile
and run the code. Clicking the Run Checks button will run pre-configured
tests against your code to calculate a grade.
Once you are happy with your results, click the Submit button to record your
score.
https://storage.googleapis.com/codevolve-assets/cengage/cengage-java-programming-9e/Figure_1-25.png
arrow_forward
Explain How inheritance is used for reusability of code. Coding example would be encourage?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
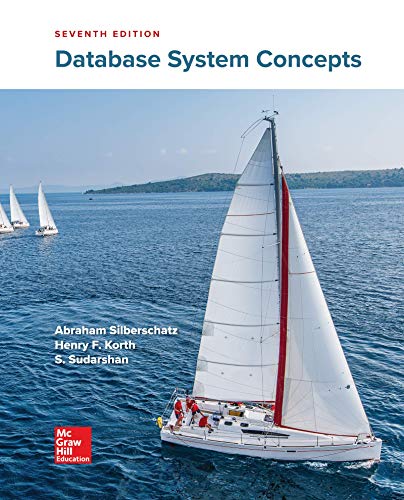
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
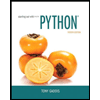
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
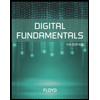
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
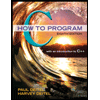
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
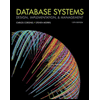
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
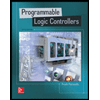
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Software Requirements: • Latest version of NetBeans IDE • Java Development Kit (JDK) 8 Procedure: 1. our progras from rhFactor non-static and private. Remove the constructor with two (2) parameters. 2o upply uncapsutation Make bloodType and 2. The names of the public setter and getter methods should be: • setBloodType() • setRhFactor() getBlood Type() getRhFactor() 3. Use the setter methods to accept user input. 4. Display the values by calling the getter methods. Sample Output: Enter blood type of patient: Enter the Rhesus factor (+ or -): O+ is added to the blood bank. Enter blood type of patient: B Enter the Rhesus factor (+ or -): B- is added to the blood bank.arrow_forwardOOP (Object-Oriented Programming) in Java Applying the composite pattern, you may create a replica of any environment you choose. Any number of media may be used, from photographs to simulators to movies to video games, and so on.arrow_forwardPROGRAMMING LANGUAGE: JAVA SUBJECT: ADVANCED OOP WITH JAVA QUESTION NO 1: Make an abstract class named A1 with 2 abstract methods, then implement those methods in child class.arrow_forward
- Instructions: modify the code below and add these features to this dice game: Multilevel Inheritance with polymorphism and includes at least one abstract class. Dynamic memory (new, delete) using pointers within a class. At least one template class. At least one operator overloading Add comments Program: File GamePurse.h: class GamePurse { // data int purseAmount; public: // public functions GamePurse(int); void Win(int); void Loose(int); int GetAmount(); }; File GamePurse.cpp: #include "GamePurse.h" // constructor initilaizes the purseAmount variable GamePurse::GamePurse(int amount){ purseAmount = amount; } // function definations // add a winning amount to the purseAmount void GamePurse:: Win(int amount){ purseAmount+= amount; } // deduct an amount from the purseAmount. void GamePurse:: Loose(int amount){ purseAmount-= amount; } // return the value of purseAmount. int GamePurse::GetAmount(){ return purseAmount; } File main.cpp: // include necessary…arrow_forwardClasses & Objects Part I DB In Java What is the purpose of Constructor Overloading? How is this related to method overloading? Provide a unique coded example of constructor overloading.arrow_forwardJAVA Language Caesar Shift Question: Modify the Caesar class so that it will allow various sized shifts to be used, instead of just a shift of size 3. (Hint: Use an instance variable in the Caesar class to represent the shift, add a constructor to set it, and change the encode method to use it.) import java.util.*; public class TestCipher { public static void main(String[] args) { int shift = 7; Caesar caesar = new Caesar(); String text = "hello world"; String encryptTxt = caesar.encrypt(text); System.out.println(text + " encrypted with shift " + shift + " is " + encryptTxt); } } abstract class Cipher { public String encrypt(String s) { StringBuffer result = new StringBuffer(""); // Use a StringBuffer StringTokenizer words = new StringTokenizer(s); // Break s into its words while (words.hasMoreTokens()) { // For each word in s…arrow_forward
- Association Relationships in Java Using the revised UML Class diagram Resto Fun Final i posted, continuedeveloping the Resto Fun system by modifying class definitions Create an Order class to break the Many-To-Many relationship between Customer and Item. Thisis a Relationship Class, a class that contains information on the association between to classes.Implement the relationship WaitsOn between Waiter and Customer, knowing that therelationship indicates that this is a One-to-Many relationship mandatory in both ends, i.e., awaiter must wait on a customer and a customer must be waited on by a waiter.Implement the relationship Orders between Customer (Table) and Item, another One-to-Many,since there are many Items a Customers can order, but each Item ordered is for one customeronly.Implement the Add relationship between Order and Item. Include a method to assign the Orderto a Customer before you start adding Items to the Order! There is enough information now to implement the…arrow_forwardJava All classes must be named as shown in the above UML diagram except the concrete child classes. The ItemList is a singly-linked list with the following methods: • insert(index: int, item: Item) : boolean o Inserts the given item at the given index if an equal item is not already in the list. If the method is successful it returns true. If an equal item already exists in the list or the index is out of range, this method returns false. • add(item: Item): boolean o Adds the given item to the front of the list. Returns true if successful and false if an equal item is already in the list. • set(index: int, item: Item) : Item o Replaces the object in the list at the given index with this object. If the index is out of range or the given item is already in the list, the method returns null. If the operation is successful, the method returns the object that was replaced by this one. • delete(index: int) : Item o Deletes the object at the given index from the list. If the index is out of…arrow_forwardSECTION C(JAVA)Look at the class definitions below and answer the questions based on it:class Node{// attributesint ID;String name; Node left; // left child Node right; // right child}class BinarySearchTree{// attributesNode root;// methodssearch(int key, Node root);void insert(int key, Node root);void delete(int key, Node root);}Write code for a method split(int key, Node root) that divides a binary searchtree into two parts. The split should occur at key and create two new binary searchtrees. Return the root of each resulting tree.Test your code with the input in the worked exampleBELOW IS THE INPUT:// id, name41, notes11, personal61, work30, shopping5, recipes55, proposal70, thesis10, muffins43, draftarrow_forward
- Mixed Fraction Arithmetic Java Project: Problem Description: Make a java program that facilitates addition, subtraction, multiplication, and division of mixed numbers (similar with the first activity) but this time all of the inputs /outputs may involve fractions, mixed fractions, or a combination. Points to consider: MixedFraction class must override the operations (add, subtract, multiplyBy, divideBy) inherited from Fraction class to have a different implementation if the MixedFraction object will have a Fraction object as parameter in performing the required operation, On top of that, the inherited operations must also be overloaded to allow a MixedFraction object to accept other parameters such as a whole number or another MixedFraction object. The toString method which is inherited must also be overridden by MixedFraction to have a more appropriate return value. Further formatting must also be considered such that if the result of the operation has a zero value for the whole…arrow_forwardComputer Science Multiple inheritances (if supported by a language) occurs when a single class inherits properties from 2 or more classes. Multiple inheritance is NOT supported in Java. One can enable multiple inheritances in Java through the use of interfaces. Show how you can enable multiple inheritance in Java and give one or more simple examples.arrow_forwardlanguage: Java Class Inheritance with an abstract class Define a class Employee with the following fields: _name, _empId, _department, _position (_title), _yearlySalary, _fullTime (a boolean: true/false) , _month , _year (time of hire) Add constructor and properties Define an abstract method GetBiMonthlySalary() (if the employee is paid twice a month) Define an abstract method GetVacationTime() that returns the number of vacation time this employee would have based on time of hire Override the ToString() method to return a string that contains the information pertaining to an employee such as name, empId, department, and where full time or not. Define a class HourlyEmployee that inherits from Employee. This class should have 2 fields of its own: _hours (hours worked in half a month) and _wage (hourly wage) Provide necessary constructor, properties and methods Override the ToString() to also return the additional fields Gets 3 days of vacation after first year if the…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
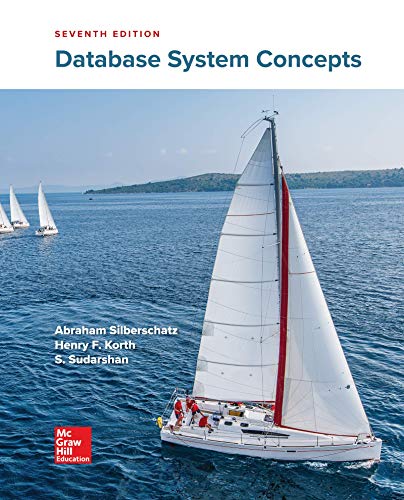
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
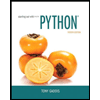
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
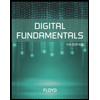
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
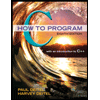
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
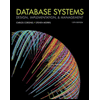
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
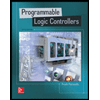
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education